gRPC API: Implementation and Testing Using a Python Framework
gRPC (Google Remote Procedure Call) is an open-source high-performance RPC (Remote Procedure Call) framework developed by Google. Designed to operate in an array of environments from mobile devices to large-scale microservices, gRPC provides developers with a host of advantages over traditional HTTP APIs and can serve as a critical component in building efficient and scalable distributed systems. This article is going to deal with the implementation level details of gRPC API using a Python framework.
Advantages of gRPC:
- Performance: gRPC optimizes for low latency, making data transmission faster and more efficient than older HTTP versions.
- Language Agnostic: With Protocol Buffers (protobufs) as its Interface Definition Language (IDL), gRPC allows developers to generate client and server code in a multitude of languages, fostering a language-independent environment.
- Streaming: gRPC natively supports streaming requests and responses, which facilitates real-time bi-directional communication, perfect for applications requiring live updates.
- Deadlines/Timeouts: gRPC allows clients to specify how long they are willing to wait for an RPC to complete. The server can check this and decide whether to complete the operation or abort if it will likely take too long.
- Pluggable: Designed to support pluggable authentication, load balancing, retries, etc., ensuring high extensibility.
- Strongly-Typed: With Protocol Buffers, both the request and response objects are strongly typed. This ensures clarity and reduces the chances of errors.
When does it make sense to use ?
- Microservices Architecture: When building a system that utilizes microservices, gRPC provides a seamless communication channel, ensuring high-speed data exchange and clear service definitions.
- Real-time Applications: For applications that demand real-time communication, like gaming or financial platforms, gRPC’s streaming feature is invaluable.
- Cross-Language Environments: For the system components written in multiple languages, gRPC’s multi-language support allows smooth and efficient communication.
- High-Performance Needs: For applications that require high-speed, low-latency communications, such as data-intensive platforms or those with vast concurrent users, gRPC’s performance advantages make it an excellent choice.
gRPC API setup and implementation:
At the outset, the main steps involved in the implementation are the following:
- Protocol Buffers (protobufs): This is a binary serialization format inherent to gRPC.
In gRPC, service and message definitions use .proto files.
The protobuf compiler then generates client and server code in multiple languages. - Service Definition: Within the .proto file, you can define a service that specifies
the RPC methods, their request and response message types. - Pluggable: gRPC supports various authentication mechanisms, load
balancing, retries, etc. It’s highly extensible. - Streaming: gRPC supports streaming requests and responses, allowing for more
complex use cases like long-lived connections, real-time updates, etc.
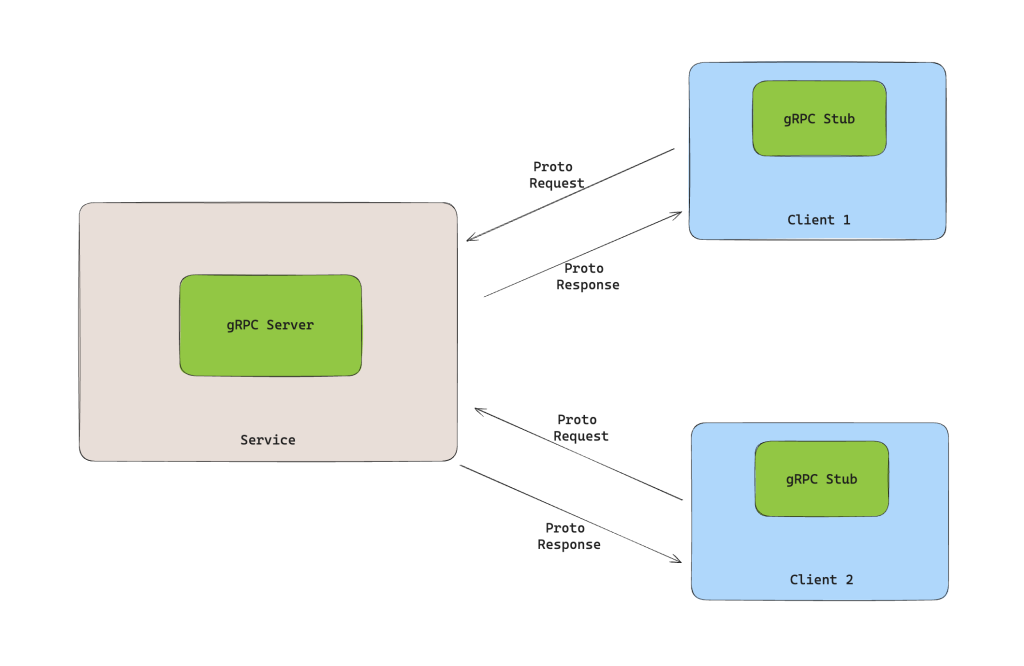
Setting up the environment:
Firstly, install the required packages:
pip3 install grpcio grpcio-tools
Sample service:
For demonstration purposes, let’s consider a simple Greeter service that has a SayHello method. We need to define a protobuf file first.
greeter.proto file:
syntax = "proto3"; package greeter; message HelloRequest { string name = 1; } message HelloResponse { string greeting = 1; } service Greeter { rpc SayHello (HelloRequest) returns (HelloResponse); }
We can generate the client and server stubs using,
python3 -m grpc_tools.protoc -I. --python_out=. --grpc_python_out=. greeter.proto
This produces two files in the folder (greeter_pb2.py and greeter_pb2_grpc.py) in which the command is run. These are called stubs and are internally used as imports by the service and the tester class.
Here are the next set of pieces that we require:
gRPC Server: Before testing, we need a running gRPC server which implements the Greeter service.
Service Implementation: In addition to defining the service in the .proto file, we need to provide the actual implementation of the service.
Here’s an example that implements these based on the Greeter service defined earlier (grpc_server.py):
import grpc from concurrent import futures import greeter_pb2 import greeter_pb2_grpc class GreeterServicer(greeter_pb2_grpc.GreeterServicer): def SayHello(self, request, context): return greeter_pb2.HelloResponse(greeting=f'Hello, {request.name}!') def serve(): server = grpc.server(futures.ThreadPoolExecutor(max_workers=10)) greeter_pb2_grpc.add_GreeterServicer_to_server(GreeterServicer(), server) server.add_insecure_port('[::]:50051') server.start() server.wait_for_termination() if __name__ == '__main__': serve()
Testing framework:
To test our gRPC service, we need a client to communicate with the server. Let’s create a testing framework that can send requests and validate responses. Create a file called grpc_tester.py which looks like below,
import grpc import greeter_pb2 import greeter_pb2_grpc class GRPCTester: def __init__(self, host, port): self.channel = grpc.insecure_channel(f'{host}:{port}') self.stub = greeter_pb2_grpc.GreeterStub(self.channel) def test_say_hello(self, name, expected_greeting): request = greeter_pb2.HelloRequest(name=name) response = self.stub.SayHello(request) assert response.greeting == expected_greeting, f"Expected {expected_greeting}, but got {response.greeting}" print(f"Test passed for input: {name}") if __name__ == "__main__": tester = GRPCTester('localhost', 50051) tester.test_say_hello('Alice', 'Hello, Alice!')
This framework initializes a connection to the server and has a test method to send a name and validate the greeting in the response. To make it extensive, you can add methods for different RPCs and include features like timeouts, metadata handling, etc.
Finally run the gRPC API service and then the tester class using the commands below,
python3 grpc_server.py python3 grpc_tester.py
In conclusion, gRPC provides an efficient and powerful way to build distributed systems, and with Python, we can build and test these systems in a short time frame. The testing framework shown here lays the foundation for more comprehensive testing solutions.