Python vs Java: The Most Important Differences
In this post, we feature a comprehensive article about Python vs Java and their most important differences. Java is an object-oriented programming language. Python is a multi-paradigm programming language. Python and Java are platform-independent languages. In this tutorial, we see the features of Python and Java to see the differences.
You can also check this tutorial in the following video:
Table Of Contents
1. Overview
We look at the comparison of Python vs Java languages in this article. They can be used for developing software and executing the code. Python is a programming language that has dynamic types. Python started off in a research firm based at Netherlands. The goal of this language was to reduce the gap between the shell commands syntax and C. The motivation of the language comes from different languages such as Algol68, Pascal and ABC. Java code is converted into bytecode after compilation. The java interpreter runs the bytecode and the output is created.
Below is a quote about Python’s popularity:
“I’ve seen quite a rise in Python in the past years. As others have stated, I think this is largely due to data science, teaching it schools/universities, and lighter frameworks like Flask (vs Django). I don’t have anything against Python (I actually enjoy writing it), but I think it’s popularity does not represent usage for enterprise use cases. I rarely see large, distributed, enterprise applications written in Python that stay in Python. They may start out in Python, but they eventually switch to something else because of performance.”
Dustin Schultz: Lead Software Engineer, Pluralsight Author, and technology evangelist
Below is a quote about Java Virtual Machine:
“Most of the fans of the language seem to like it because it’s the “new shiny,” and/or they have Java Allergy (usually based on a view of the platform that’s >10 years out of date), but … I haven’t seen anything compelling in it that couldn’t also be done fairly easily in JVM tech (albeit with potentially a bit more ceremony) — and the JVM has much better, broader integrations with libraries.“
Ben Evans Principal Engineer and JVM Technologies Architect at New Relic, Inc
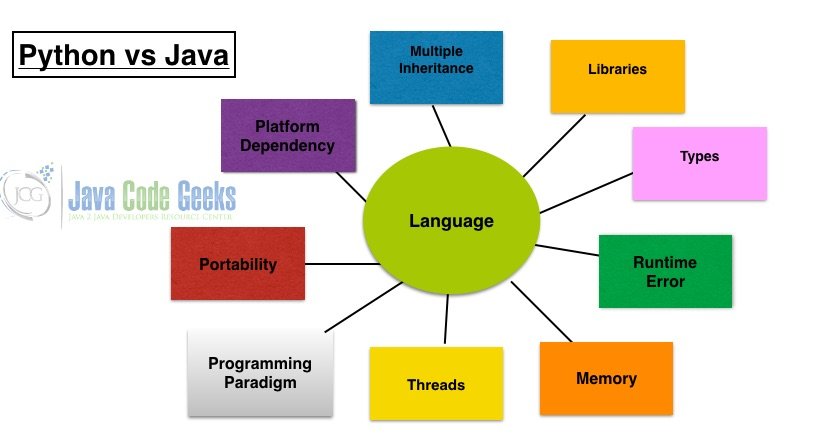
2. Python vs Java
2.1 Prerequisites
Python 3.6.8 is required on the windows or any operating system. Pycharm is needed for python programming.Java 8 is required on the linux, windows or mac operating system. Eclipse Oxygen can be used for this example.
2.2 Download
Python 3.6.8 can be downloaded from the website. Pycharm is available at this link. You can download Java 8 from the Oracle web site . Eclipse Oxygen can be downloaded from the eclipse web site.
2.3 Setup
2.3.1 Python Setup
To install python, the download package or executable needs to be executed.
2.3.2 Java Setup
Below is the setup commands required for the Java Environment.
Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.4 IDE
2.4.1 Pycharm Setup
Pycharm package or installable needs to be executed to install Pycharm.
2.4.2 Eclipse Oxygen Setup
The ‘eclipse-java-oxygen-2-macosx-cocoa-x86_64.tar’ can be downloaded from the eclipse website. The tar file is opened by double click. The tar file is unzipped by using the archive utility. After unzipping, you will find the eclipse icon in the folder. You can move the eclipse icon from the folder to applications by dragging the icon.
2.5 Launching IDE
2.5.1 Pycharm
Launch the Pycharm and start creating a pure python project named HelloWorld. The screen shows the project creation.
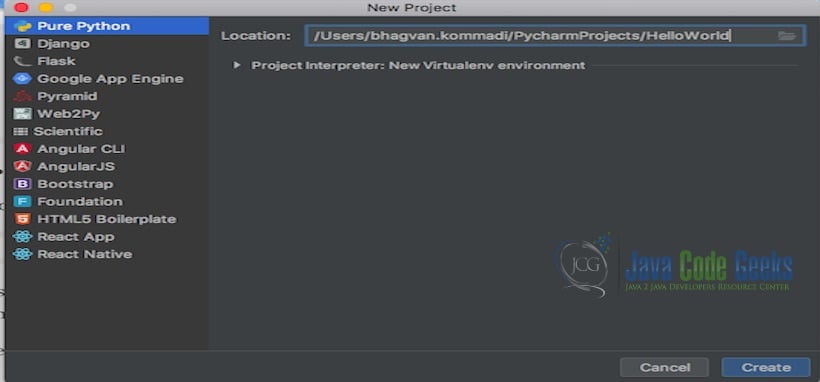
The project settings are set in the next screen as shown below.
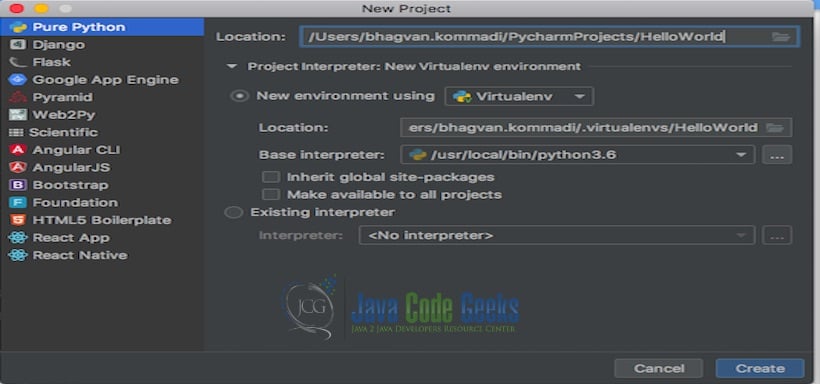
Pycharm welcome screen comes up shows the progress as indicated below.
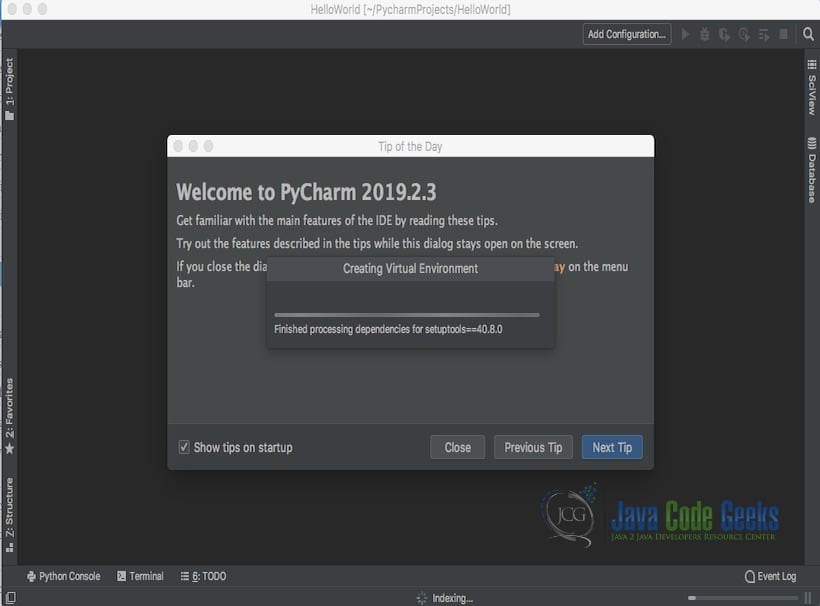
You can create a Hello.py and execute the python file by selecting the Run menu.
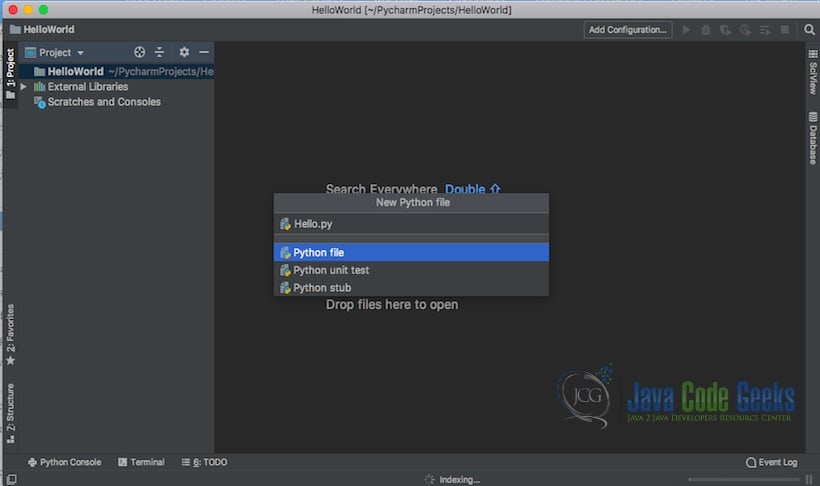
The output message “Hello World” is printed when the python file is Run.
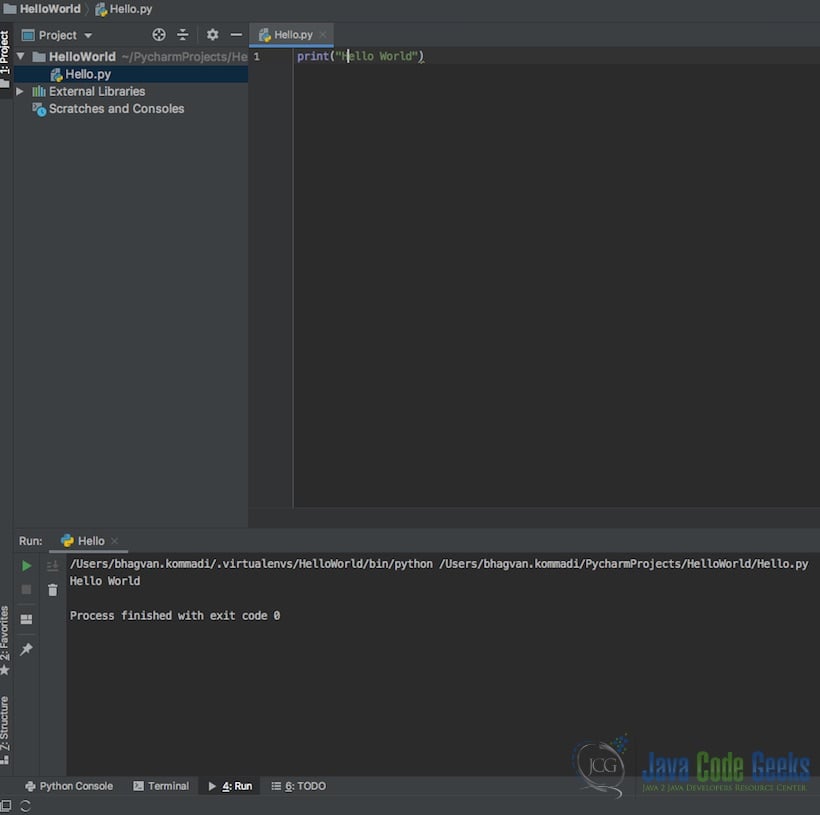
2.5.2 Eclipse Java
Eclipse has features related to language support, customization, and extension. You can click on the eclipse icon to launch eclipse. The eclipse screen pops up as shown in the screen shot below:
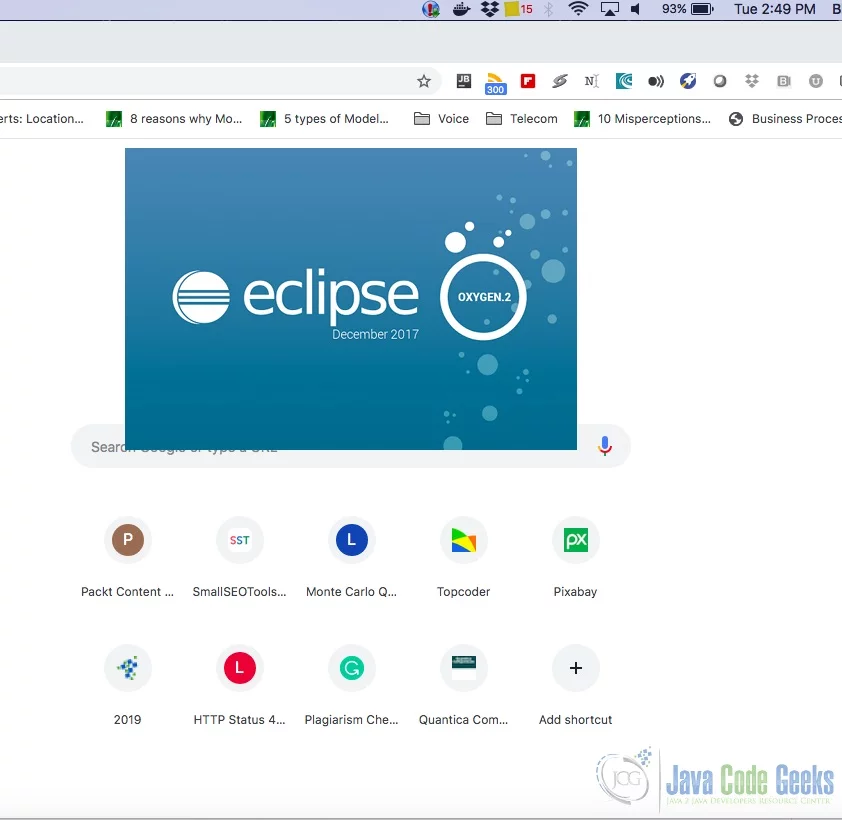
You can select the workspace from the screen which pops up. The attached image shows how it can be selected.
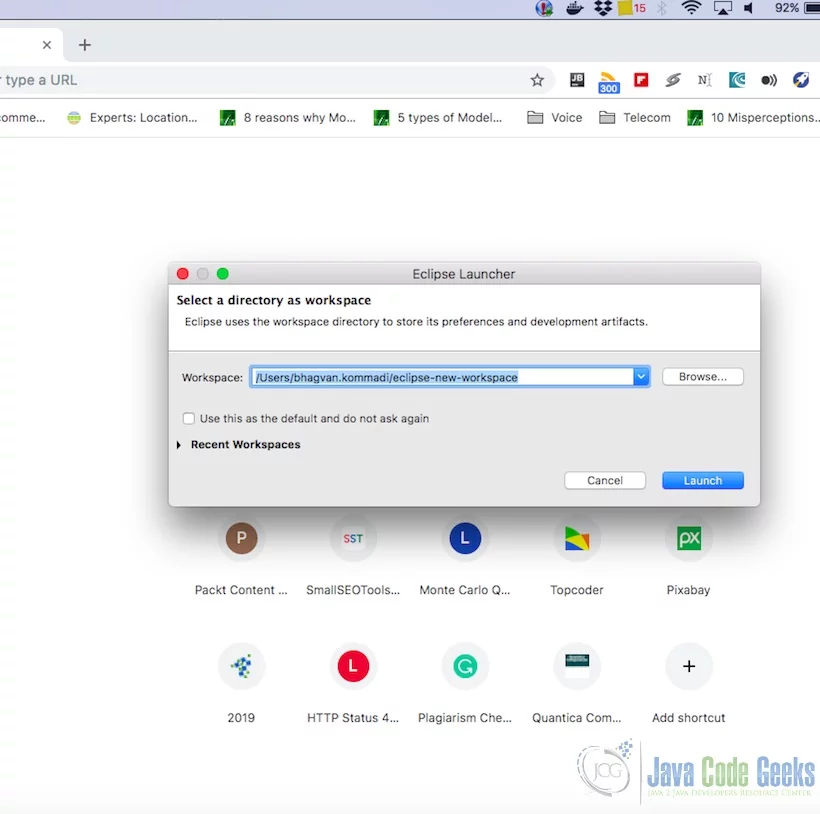
You can see the eclipse workbench on the screen. The attached screen shot shows the Eclipse project screen.
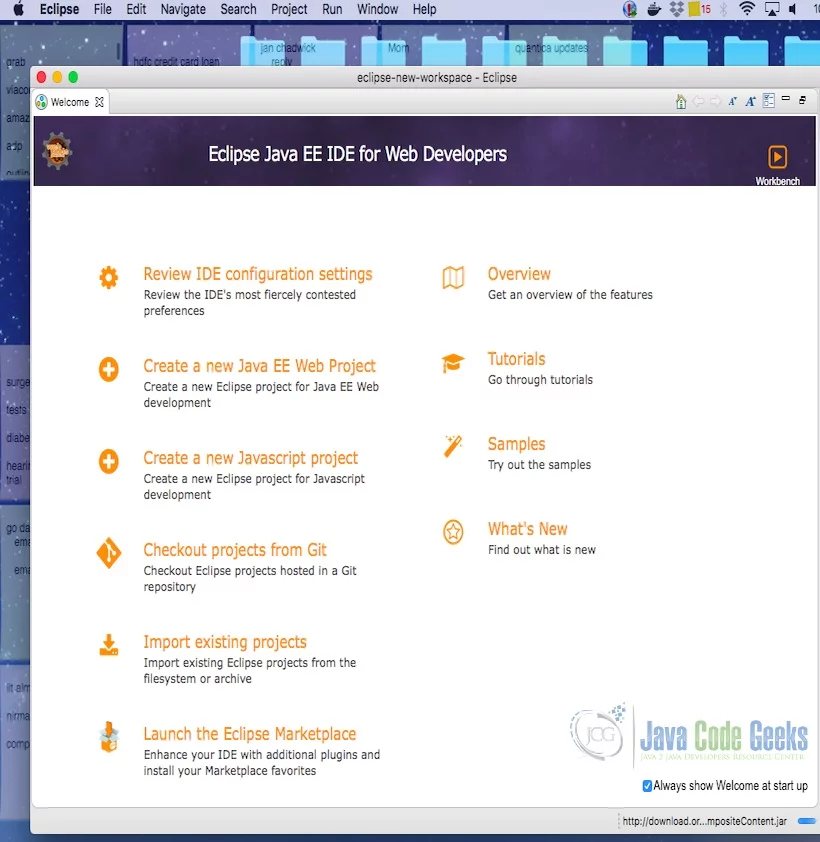
Java Hello World
class prints the greetings. The screenshot below is added to show the class and execution on eclipse.
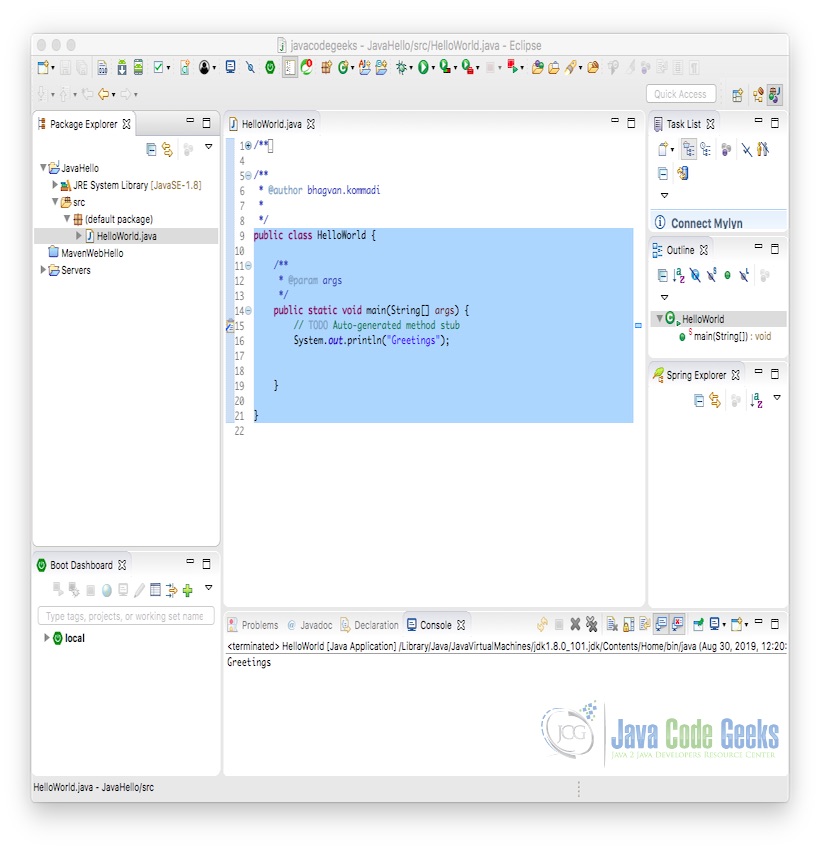
2.6 Memory Management
Python language has reference counting and garbage collection features related to memory management. Java language has features related to memory management and it is a memory safe language. Garbage collection is a feature which helps in collecting the resources which are free and released. Developer in java cannot go beyond the allocated memory. In java, when the memory is consumed beyond the allocation, it throws an error.
2.7 Exceptional Handling
Python has try-except-else-finally statements for exception handling. In Java, exception handling is possible by using try, catch and finally blocks.
2.8 Multiple Inheritance
Multiple inheritance is supported in Python. Let us take an example to see how it is handled in Python and Java. Truck is an vehicle and a machine.
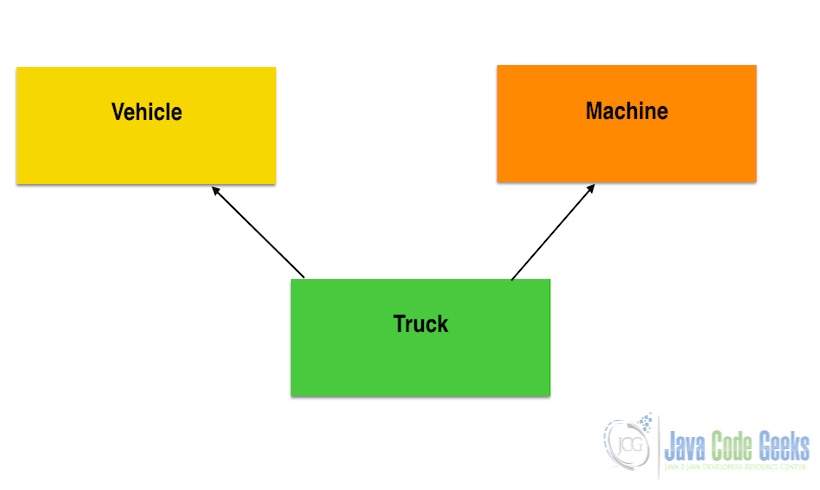
Multiple Inheritance
class Vehicle: def __init__(self): self.velocity = 50 def getVelocity(): return self.velocity class Machine: def __init__(self): self._distanceTravelled = 100 def getDistance(): return self.distanceTravelled class Truck(Vehicle, Machine): def __init__(self, velocity,time): Vehicle.__init__(self) Machine.__init__(self) self.velocity = velocity self.time = time def getVelocity(): return self.distanceTravelled/self.time def getDistance(): return self.velocity * time
.Java does not support multiple inheritance. Each class is able to extend only on one class but is able to implement more than one interfaces. The sample code shows below Truck
class implementing interfaces Machine
and Vehicle
Interfaces
interface Machine { int distanceTravelled=100; public int getDistance(); } interface Vehicle { int velocity=50; public int getVelocity(); } public class Truck implements Machine, Vehicle { int time; int velocity; int distanceTravelled; public Truck(int velocity, int time) { this.velocity = velocity; this.time = time; } public int getDistance() { distanceTravelled= velocity*time; System.out.println("Total Distance is : "+distanceTravelled); return distanceTravelled; } public int getVelocity() { int velocity=distanceTravelled/time; System.out.println("Velocity is : "+ velocity); return velocity; } public static void main(String args[]) { Truck truck = new Truck(50,2); truck.getDistance(); truck.getVelocity(); } }
2.9 Threads
Python has inbuilt threads which can be used. The example shown below has the features related to creating and starting new thread.
Threads
import logging import threading import time def invokeSleep(name): logging.info("Thread is %s: starting", name) time.sleep(2) logging.info("Thread is %s: finishing", name) if __name__ == "__main__": format = "%(asctime)s: %(message)s" logging.basicConfig(format=format, level=logging.INFO, datefmt="%H:%M:%S") logging.info("creating thread") aThread = threading.Thread(target=invokeSleep, args=(1,)) logging.info("before starting thread") aThread.start() logging.info("thread finishing")
Java has in built classes to create threads. To create a new thread, a class has to extend a Thread
class and the run method has to be overridden.
Thread
public class NewThread extends Thread { public void run() { System.out.println("Thread running now"); } public static void main(String args[]) { NewThread newThread =new NewThread(); newThread.start(); } }
Java provides another way to create Threads. A class which implements Runnable
can be instantiated and passed as a parameter to the Thread
class. Sample code is provided below:
ThreadObject-Runnable
public class ThreadObject implements Runnable { public void run() { System.out.println("ThreadObject running"); } public static void main(String args[]) { ThreadObject threadObject =new ThreadObject(); Thread thread =new Thread(threadObject); thread.start(); } }
2.10 Portability
Python Language is portable. The python compiler creates python bytecode which is executed using python virtual machine.
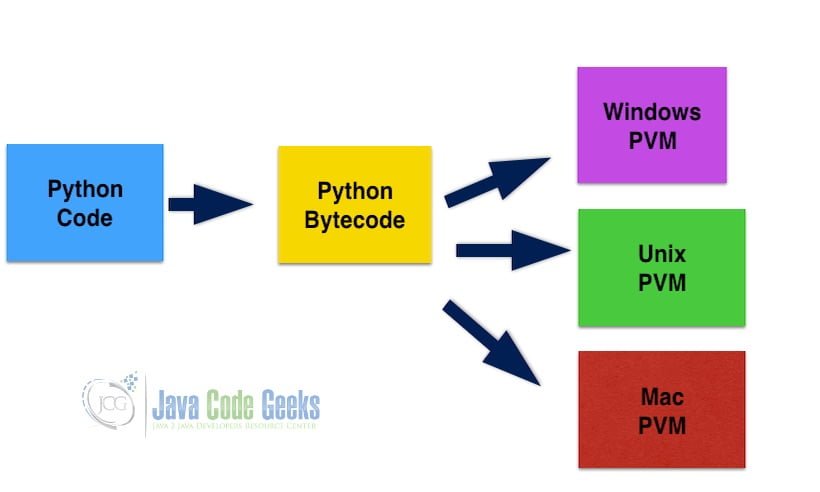
Java language is interpreted by the java interpreter on the computer independent of the operating system.
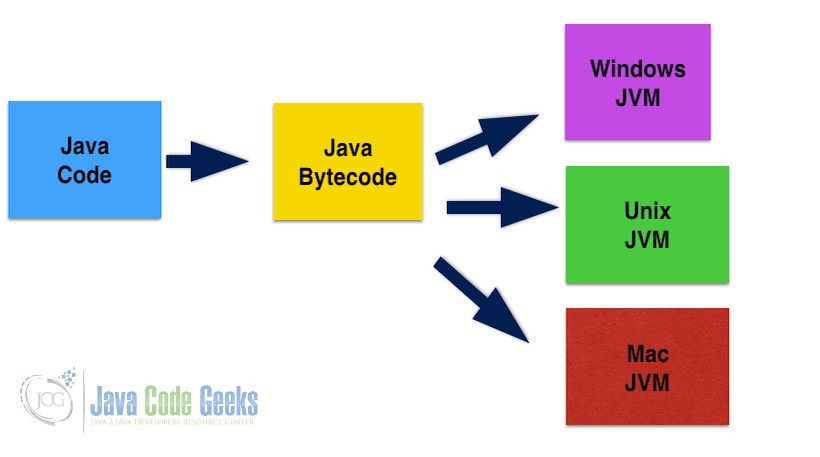
Python portability is not as popular as Java.
2.11 Types
Python compiler does type checking while the code gets intrepreted. In python, variable type can be modified during the life cycle. Duck typing is related to a concept where the definition of the method is more important than the object’s class or type.
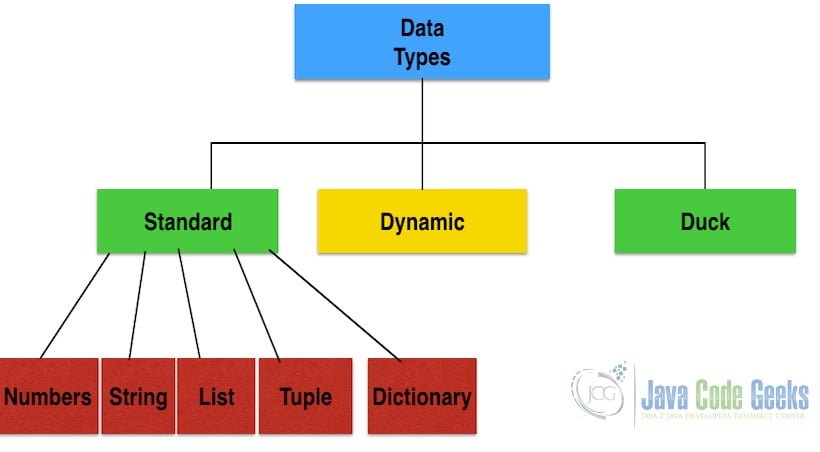
Java language has primitive and object types. Java has features related to autoboxing which converts the types automatically. The java.lang.Object
class is the base class for all the classes and Java follows the single root chain of command.
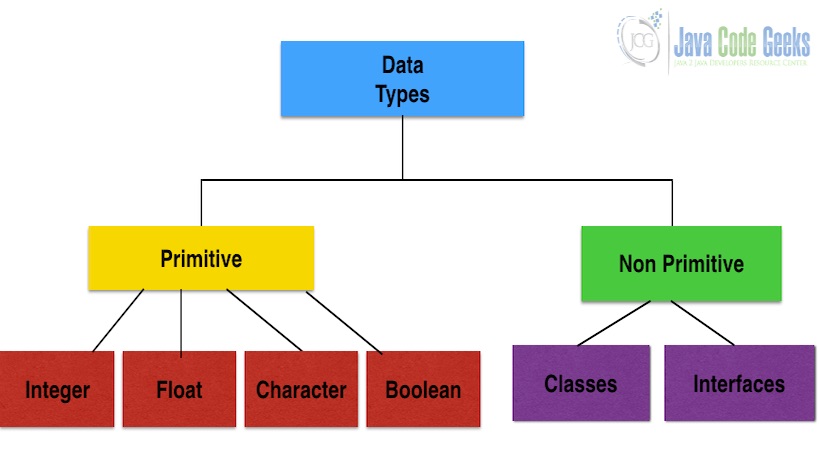
2.12 Libraries
Python libraries consists of modules. Python modules have functions and methods.
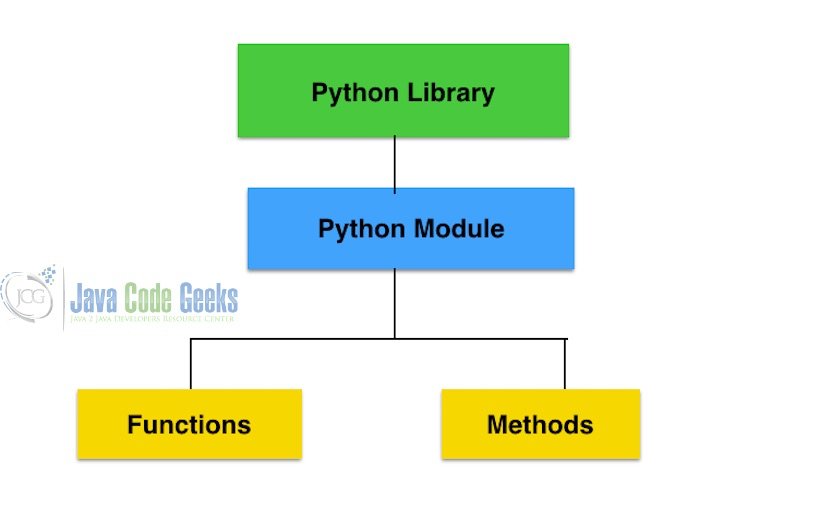
Java packages help in packaging classes. Package scope is another feature in Java language. Java archives help in grouping the package of classes for execution and installation purposes.
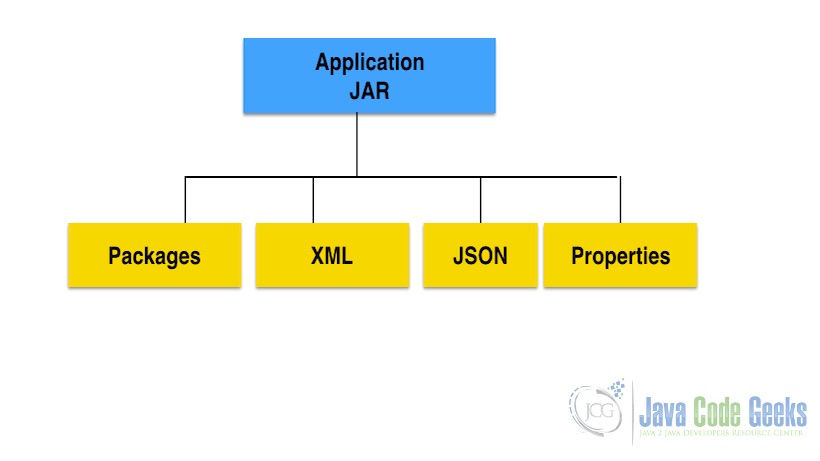
2.13 Runtime Errors
In python, runtime errors occur when RuntimeError is raised. When Illegal operations happen, exceptions are raised in python.
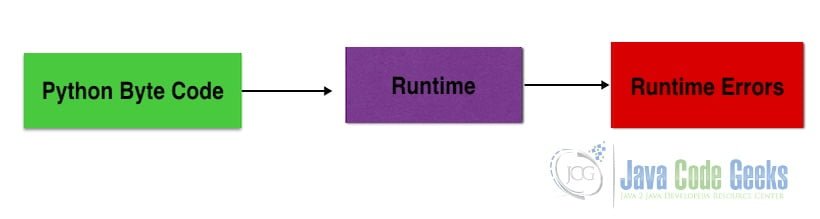
In java, runtime errors are presented by the compiler and the interpreter.
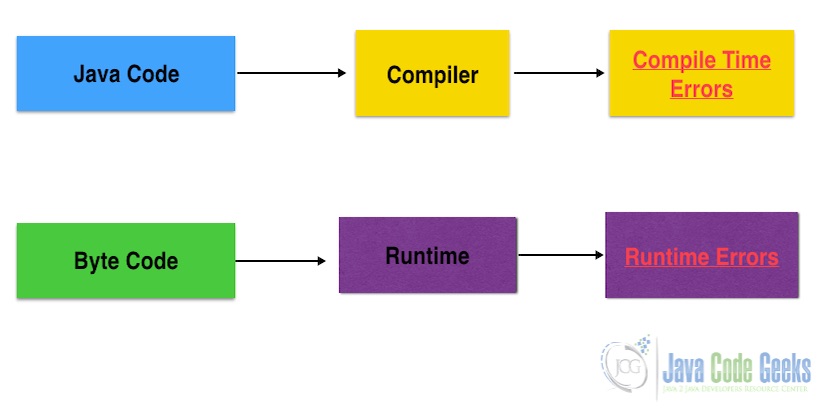
2.14 Documentation
Python has pydoc module for generating documentation. Java has feature to support comments which can be used for documentation generator.
2.15 Mobile & Web & Desktop
Python language is used for web and desktop command line applications.Java language can be used for mobile, web and desktop application development. Java is supported by Android but python is not supported.
2.16 Programming Paradigm
Python supports object-oriented, functional, imperative, and procedural programming paradigms. Java is an object-oriented, class and concurrent programming language. Java is currently supporting functional programming features from version 8.
3. Conclusion
Overall, Java has great benefits over Python. The comparison table below captures the differences between Python and Java.
Comparison Table
Feature | Python | Java |
Memory Management | Python has two features related to memory management. They are reference counting and garbage collection | Garbage collection is a feature in Java.Pointers are not there in Java. Java programs consume more memory |
Inheritance | Multiple Inheritance is supported in Python | Interfaces can be used for multiple inheritance. Single Inheritance is supported in Java. |
Threads | Python has support for threads. | Java has class Thread and interface Runnable to use threads. |
Portability | Python byte code is platform dependent | Java byte code is platform dependent. |
Access Control | Python has a concept of non public instance variables and properties . | Encapsulation helps in access control of the class variables and properties in java. |
Types | Dynamic and Duck Typing is supported in Python | Single root chain of command pattern is used in Java. |
Libraries | Python libraries consists of modules. Module has functions and methods. | Java archives are used for building java libraries. |
Runtime error | Python raises exceptions when runtime errors occur. RuntimeError is raised for programmatic error handling. | Runtime errors are detected in compilation and execution stages in Java |
Performance | Python is slower than Java | Java performance is faster compared to python |
The quote below from stack overflow summarizes the python vs Java popularity and adoption in developer community.
“Python, the fastest-growing major programming language, has risen in the ranks of programming languages in our survey yet again, edging out Java this year and standing as the second most loved language (behind Rust).”
StackOverflow 2019
4. Download the Source Code
You can download the full source code of this example here: Python vs Java: The Most Important Differences
I m mainly a Java’er :-) if that’s a word, though I use other languages including Python. It would be good to also cover local & remote debugging, security, memory footprint, etc capabilities & requirements
sure…
There were two technical issues I noticed: Java does support multiple inheritance using interfaces, and Python threading uses the Global Interpreter Lock (GIL), and so Python can’t take full advantage of multi-core processors.
available support in the languages… it may not give complete advantage
I mainly use Java, but also use Python for my own scripting and log analysis, and disagree with some of the analysis here: 1 – Your diagram of Java and Python byte code indicates that both generate platform independent byte code that runs in a virtual machine – but your chart says that Python byte code is platform dependent. 2 – While both Python and Java support threads, the thread support in Python is limited by the Global Interpreter Lock (GIL), which prevents threads from running concurrently on separate processors. Java does not have this limitation, and can make use… Read more »
byte code needs specific intrepreter on that OS… clarifying on your first point
Great Article!! Thanks for sharing this great information about python vs java. Keep Sharing