IntelliJ/Android Studio Plugin Development Tutorial – Getting Started
In this intellij plugin development tutorial, we’ll be taking a look at how to build a simple plugin for IntelliJ. And since Android Studio is also built on IntelliJ, it can be used in Android Studio as well.
Wait, why do I need a plugin?
Automate the boring stuff: That’s the sole reason why I use intellij plugins. I’ve used intellij plugin to automate my builds on Jenkins and Bitrise.
You can probably use it to automate some of your mundane tasks. Figure out what you do on a daily basis inside Android Studio/ IntelliJ IDEA/ Webstorm or any intellij based product and figure out how you can solve it with a plugin.
Earn a quick buck: This might not be the most exciting of the ways available, but you can make a quick buck if your plugin takes off. JetBrains has a marketplace for plugins where you can develop and publish your own plugin. Take a look here.
Show off: You can create something that everyone in your circle/org can use and make their lives easier.
What do you need?
You’ll need some prior knowledge of Java/Kotlin for this tutorial. For the most part, I’ll be using Kotlin. But if you’re from a Java background then picking up Kotlin is child’s play.
Also, for building a plugin in intellij, you’ll need to install IntelliJ on your computer. IntelliJ Community Edition works just fine!
Getting Started
First things first, create a new project in IntelliJ.
On the left hand pane select Gradle as your build tool. Also, from the right hand pane, select:
- IntelliJ platform plugin
- Java
- Kotlin/JVM
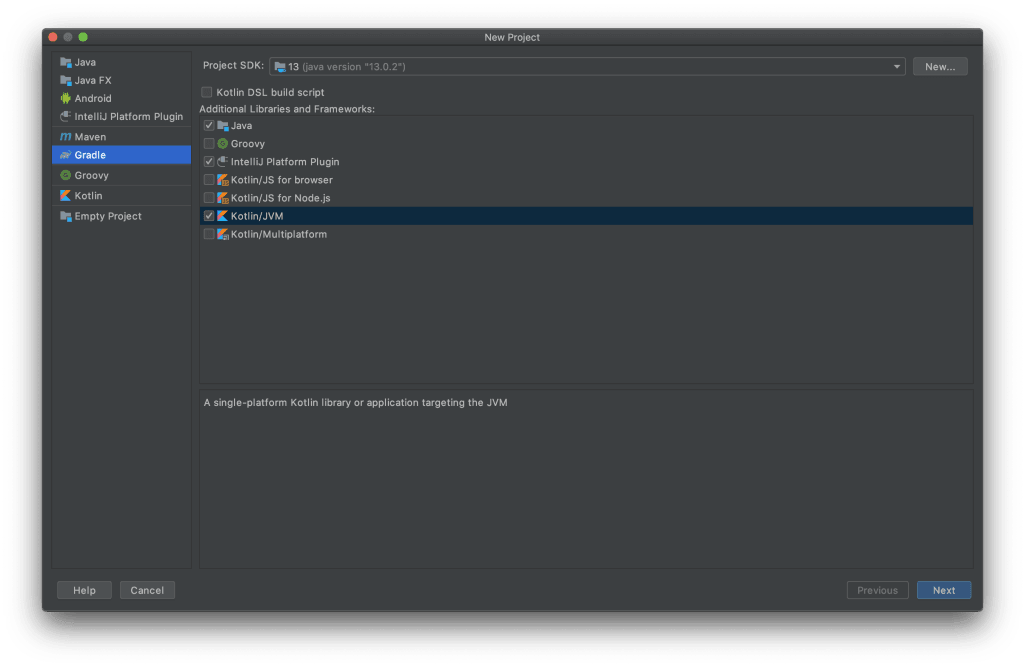
This will install the required dependencies for Kotlin/ Java and the plugin. I’ve attached a screenshot of the same above.
Understanding the project structure
Plugin.xml
This is a xml file where you have to declare all of your actions. This is like AndroidManifest.xml (if you’re an android dev you’ll relate).
It’s a configuration file where you can specify the Name of your plugin, the description of your plugin, you can specify the location/the group that your action would be a part of and much more. We’ll take a look into this file in more detail soon.
Kotlin/Java
This is where you’ll write your java/kotlin code. Since we’ve included dependencies for both, you can write your code in either Java/Kotlin whatever your prefer.
This tutorial would mostly be Kotlin first, but if you’re comfortable with Java you’ll catch up kotlin in no time.
build.gradle
We’re using gradle as our build tool and you can declare all the dependencies for your project in build.gradle. This can be found in the root directory of your plugin.
For the sake of this tutorial, we’ll just have dependencies for Kotlin and Java installed.
Creating an Action
Now let’s write some code. For this intellij plugin development tutorial, we’ll learn how to show a notification on the bottom right corner of the IDE.
I’m sure most of you would’ve noticed this when you make a change in your build.gradle/package.json. It reminds you to sync your project. It reminds you of new updates for your IDE or the latest kotlin version.
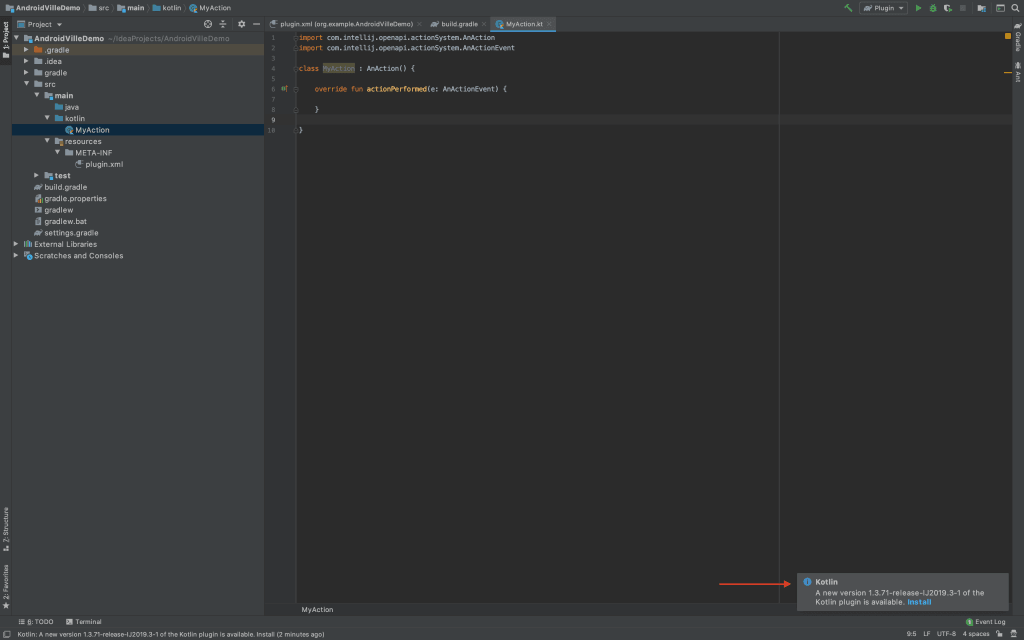
An action is basically any menu option that allows you to perform a function in the IDE. For example in File -> Open, Open is an action. It allows you to open a new project.
Actions are the way you can add your desired option to IntelliJ based IDEs (such as Android Studio).
Create a file named MyNotificationAction.kt inside the kotlin directory. Let’s create a class in this file named MyNotificationAction and extend from AnAction.
1 2 3 4 5 | class MyNotificationAction : AnAction() { } |
AnAction is an abstract class from which all the actions are derived. IDE calls methods on these action classes whenever the user triggers an Action.
Override the actionPerformed method. This gets called whenever the user triggers an action.
1 2 3 4 5 6 7 8 | class MyNotificationAction : AnAction() { override fun actionPerformed(e: AnActionEvent) { } } |
This is the place where we’ll write the code to create and display a notification.
Creating the Notification
Creating a notification in intellij plugin is fairly simple. Here’s how to create a notification:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 | class MyNotificationAction : AnAction() { override fun actionPerformed(e: AnActionEvent) { val notification = NotificationGroup( "AndroidVille" , NotificationDisplayType.BALLOON, true ) notification .createNotification( "Hello from first plugin" , "Welcome to AndroidVille's intellij plugin development tutorial" , NotificationType.INFORMATION, null ).notify(e.project) } } |
Notification type is BALLOON which is the notification that comes in bottom right.
Putting it all together
I mentioned earlier that we need to register all the actions in the Plugin.xml file for the IDE to recognise it and place it correctly. We’ll be making our plugin a part of the top menu bar.
Here’s how to register the plugin in plugin.xml
01 02 03 04 05 06 07 08 09 10 11 12 13 | <actions> <group id= "MyCustomPlugin.TopMenu" text= "AndroidVille" description= "This is a plugin to generate a simple notification" > <add-to-group group-id= "MainMenu" anchor= "last" /> <action id= "MyCustomAction" class = "MyNotificationAction" text= "Trigger Notification" description= "Click to display notification" /> </group> </actions> |
- <group> is used to group together similar actions. If you want to group together notifications, messagebox or anything else in the similar dropdown menu, you can declare your actions in this group.
- <add-to-group> is used to specify which existing group to place your group in. It takes mandatory group id which is specific to each group. For adding to the top menu we have group id is MainMenu. You can find the group Ids for other places in the docs or by Ctrl/Cmd + click on the group id. It’ll list all existing groups with their ids.
- <action> is the tag used to register your action finally. Important attributes are id and class. In class, mention the reference to your action class.
Running/Debugging your plugin
We’re almost ready to test out our plugin. Head over to build.gradle file and inside intellij section, declare your testing IDE. It should be and intellij based IDE like Android Studio, WebStorm etc.
1 2 3 4 5 | intellij { version '2019.3.3' alternativeIdePath "/Applications/WebStorm.app" } |
You’ll get a notification to import gradle changes. Do that and then finally click on the play button. Your IDE will show up and you can see the plugin in the toolbar
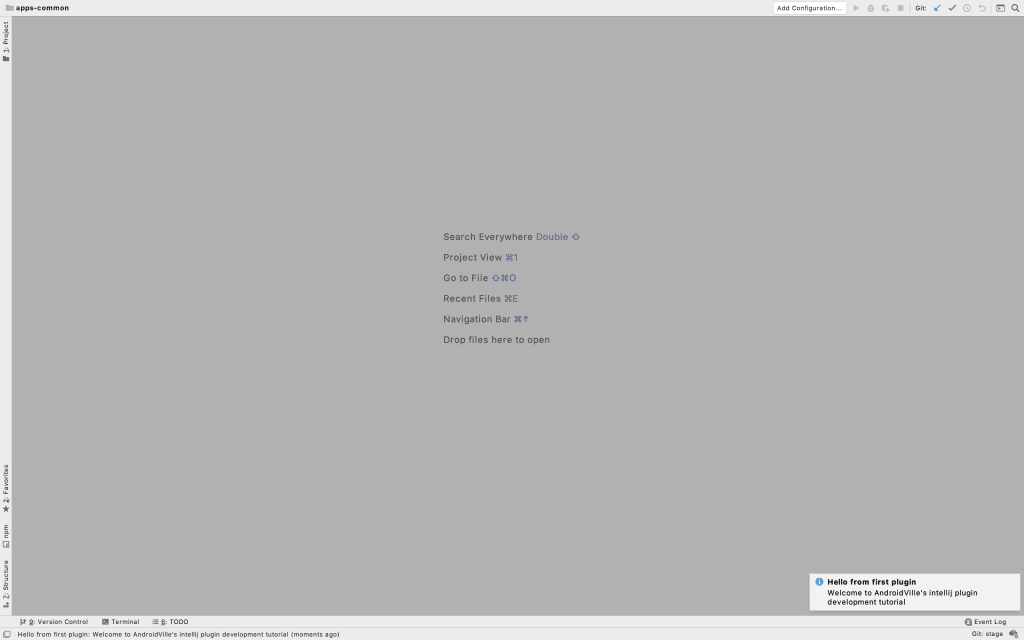
Conclusion
This ends the part 1 of our intellij plugin development tutorial. We saw how to create a notification in intellij.
In the upcoming parts, we’ll take a look at how to show message box to the user, handling user inputs and finally, I’ll show you how to build a plugin to automate your builds on Bitrise. So stay tuned as the upcoming tutorials are gonna be really exciting and helpful.
Published on Java Code Geeks with permission by Ayusch Jain, partner at our JCG program. See the original article here: IntelliJ/Android Studio Plugin Development Tutorial – Getting Started Opinions expressed by Java Code Geeks contributors are their own. |