Read replicas and Spring Data Part 2: Configuring the base project
In our previous post we set up multiple PostgreSQL instances with the same data.
Our next step would be to configure our spring project by using the both servers.
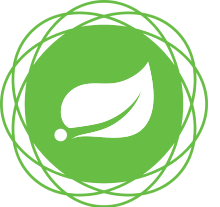
As stated previously we shall use some of the code taken from the Spring Boot JPA post, since we use exactly the same database.
This shall be our gradle build file
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | plugins { id 'org.springframework.boot' version '2.1.9.RELEASE' id 'io.spring.dependency-management' version '1.0.8.RELEASE' id 'java' } group = 'com.gkatzioura' version = '0.0.1-SNAPSHOT' sourceCompatibility = '1.8' repositories { mavenCentral() } dependencies { implementation 'org.springframework.boot:spring-boot-starter-data-jpa' implementation 'org.springframework.boot:spring-boot-starter-web' implementation "org.postgresql:postgresql:42.2.8" testImplementation 'org.springframework.boot:spring-boot-starter-test' } |
Now let’s proceed on creating the model based on the table created on the previous blog.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | package com.gkatzioura.springdatareadreplica.entity; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table (name = "employee" , catalog= "spring_data_jpa_example" ) public class Employee { @Id @Column (name = "id" ) @GeneratedValue (strategy = GenerationType.IDENTITY) private Long id; @Column (name = "firstname" ) private String firstName; @Column (name = "lastname" ) private String lastname; @Column (name = "email" ) private String email; @Column (name = "age" ) private Integer age; @Column (name = "salary" ) private Integer salary; public Long getId() { return id; } public void setId(Long id) { this .id = id; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this .firstName = firstName; } public String getLastname() { return lastname; } public void setLastname(String lastname) { this .lastname = lastname; } public String getEmail() { return email; } public void setEmail(String email) { this .email = email; } public Integer getAge() { return age; } public void setAge(Integer age) { this .age = age; } public Integer getSalary() { return salary; } public void setSalary(Integer salary) { this .salary = salary; } } |
And the next step is to create a spring data repository.
1 2 3 4 5 6 7 | package com.gkatzioura.springdatareadreplica.repository; import org.springframework.data.jpa.repository.JpaRepository; import com.gkatzioura.springdatareadreplica.entity.Employee; public interface EmployeeRepository extends JpaRepository<Employee,Long> { } |
Also we are going to add a controller.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | package com.gkatzioura.springdatareadreplica.controller; import java.util.List; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.gkatzioura.springdatareadreplica.entity.Employee; import com.gkatzioura.springdatareadreplica.repository.EmployeeRepository; @RestController public class EmployeeContoller { private final EmployeeRepository employeeRepository; public EmployeeContoller(EmployeeRepository employeeRepository) { this .employeeRepository = employeeRepository; } @RequestMapping ( "/employee" ) public List<Employee> getEmployees() { return employeeRepository.findAll(); } } |
All that it takes is to just add the right properties in you application.yaml
1 2 3 4 5 6 7 | spring: datasource: platform: postgres driverClassName: org.postgresql.Driver username: db-user password: your-password url: jdbc:postgresql: //127.0.0.2:5432/postgres |
Spring boot has made it possible nowadays not to bother with any JPA configurations.
This is all you need in order to run the application. Once your application is running just try to fetch the employees.
1 | curl http: //localhost :8080 /employee |
As you have seen we did not do any JPA configuration. Since Spring Boot 2 specifying the database url is sufficient for the auto configuration to kick in and do all this configuration for you.
However in our case we want to have multiple datasource and entity manager configurations. In the next post we shall configure the entity managers for our application.
Published on Java Code Geeks with permission by Emmanouil Gkatziouras, partner at our JCG program. See the original article here: Read replicas and Spring Data Part 2: Configuring the base project Opinions expressed by Java Code Geeks contributors are their own. |