Spring Boot Error – Error creating a bean with name ‘dataSource’ defined in class path resource DataSourceAutoConfiguration
Hello guys, If you are using Spring Boot and getting errors like “Cannot determine embedded database driver class for database type NONE” or “Error creating a bean with name ‘dataSource’ defined in class path resource ataSourceAutoConfiguration” then you have come to the right place. In this article, we’ll examine different scenarios on which this Spring Boot error comes and what you can do to solve them. The general reason for this error is Spring Boot’s auto-configuration, which is trying to automatically configure a DataSource
for you but doesn’t have enough information. It is automatically trying to create an instance of DataSourceAutoConfiguration
bean and it’s failing.
Like other Spring frameworks errors, the stack trace looks quite messy, something which they could have improved with Spring Boot, but the gest is here are these two errors I mentioned above.
Let’s see the stacktrace looks in general:
org.springframework.beans.factory.BeanDefinitionStoreException: Factory method [public javax.sql.DataSource org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration$NonEmbeddedConfiguration.dataSource()] threw exception; nested exception is org.springframework.beans.factory.BeanCreationException: Cannot determine embedded database driver class for database type NONE. If you want an embedded database please put a supported one on the classpath.:
[INFO] org.springframework.beans.factory.BeanCreationException: Cannot determine embedded database driver class for database type NONE. If you want an embedded database please put a supported one on the classpath.
1) Spring Boot Error due to Starter Dependency
Some of my friends and readers got this error even if they don’t need a Database. The main reason they were getting this error was because of starter dependency like some of they have included spring-boot-starter-data-jpa
which then included hibernate-entitymanager.jar
and they didn’t have additional things need to set that up.
Sometimes including incorrect Starter POM can also solve this problem like adding spring-boot-starter-jdbc
instead of spring-boot-starter-data-jpa
dependency.
If you know, Spring Boot auto-configuration is triggered by JAR dependencies present in the classpath and if it pulls something which you don’t need then this type of error can come.
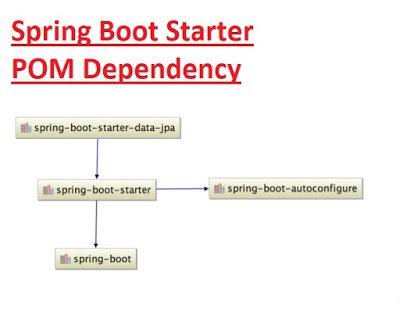
2) Due to Missing Dependency
Sometimes you do need a database but you forgot to include the
driver JAR file into the classpath, which can also cause this error. For example, you have specified the following properties in the application.properties
, spring boots configuration file but didn’t include the corresponding MySQL JDBC driver into the classpath
spring.datasource.url = jdbc:mysql://localhost/test
spring.datasource.driver-class-name= com.mysql.jdbc.Driver
In order to solve this error, either you need to include the correct Starter POM dependency or manually add the MySQL JDBC JAR file into the classpath. If you are interested, you can see this tutorial to learn more about how to connect a Java application to a database using a MySQL database inthis tutorial.
3) Due to Missing Configuration in Application.properties
Spring Boot is good at configuring in-memory Databases like H2, HSQLDB, Derby, etc and it can configure them by just adding their JAR files into the
classpath but for others, you need to give Spring Boot additional details like URL, DriverClass name, etc.
You can do that by adding some properties to application.properties file with the spring.datasource
prefix, as shown in following example:
spring.datasource.url = jdbc:mysql://localhost/abc
spring.datasource.name=testme
spring.datasource.username=xxxx
spring.datasource.password=xxxx
spring.datasource.driver-class-name= com.mysql.jdbc.Driver spring.jpa.database=mysql
spring.jpa.database-platform=org.hibernate.dialect.MySQLDialect
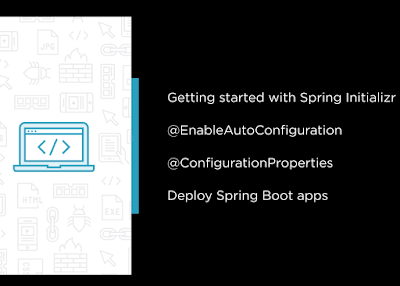
4) Exclude DataSourceAutoConfiguration
Sometimes excluding DataSourceAutoConfigution
can also solve this problem, especially if you don’t need Database. This will prevent Spring Boot from automatically configuration database and there won’t be any error. You can disable auto-configuration for certain classes by using the exclude Attribute of@EnableAutoConfiguration annotation of Spring Boot as shown below:
1 2 3 4 5 6 7 8 9 | @Configuration @EnableAutoConfiguration (exclude={DataSourceAutoConfiguration. class }) public class SpringBootDemo { public static void main(String[] args) { SpringApplication.runSpringBootDemo. class , args); } } |
You can even exclude more than one classes using exclude attribute with
@EnableAutoConfiguration as shown below:
01 02 03 04 05 06 07 08 09 10 | @Configuration @EnableAutoConfiguration ( exclude = {DataSourceAutoConfiguration. class , HibernateJpaAutoConfiguration. class }) public class SpringBootDemo { public static void main(String[] args) { SpringApplication.runSpringBootDemo. class , args); } } |
That’s all about how to solve “Cannot determine embedded database driver class for database type NONE” or “Error creating a bean with name ‘dataSource’ defined in class path resource DataSourceAutoConfiguration” problem. In most of the cases, it is because of auto-configuration doesn’t have enough details require to configure Database but sometimes it’s also the accidental trigger of database auto-configuration which can be disabled using exclude attribute of @EnableAutoConfiguration
annotation.
Btw, if you want to learn Spring Boot in depth, here are some useful resources for your learning:
Published on Java Code Geeks with permission by Javin Paul, partner at our JCG program. See the original article here: Spring Boot Error – Error creating a bean with name ‘dataSource’ defined in class path resource DataSourceAutoConfiguration Opinions expressed by Java Code Geeks contributors are their own. |
that’s great thans a lot author
I face same issue, it due to whitespace character inside my application.properties file.
Find the whitespace and remove it. It work fine now