Python Formatting Strings Example
1. Introduction
In this post, we feature a comprehensive article on Python Formatting Strings. One of the tasks we face almost every day while writing code for our world-changing apps is, formatting Strings. Although it sounds like a trivial task, it is necessary to format Strings correctly because not doing it can result in a lot of memory leaks as well. These memory leaks occur because of the extra String objects made due to our incorrect formatting of the String messages.
In this quick lesson, we will see how can we format Strings in Python, how we can accommodate various data types and avoid common memory leaks. We will also look at one of the most popular String formatting libraries which can be used in production applications with built-in optimizations for this task so that you don’t have to worry about anything apart from your business logic (mostly).
2. Python Formatting Strings
In this section, let’s look at various examples on how we can format Strings and how different data types can be managed inside a String for a pretty message that we intend to show on the screen.
2.1 Simple String formatting
We will start with a very simple example where we will format a single String inside a Python print statement. To indicate placeholders in Strings, we usually make use of the % character to signify that something needs to replaced here. Here is a simple code snippet that provides the same example:
print('%s %s' % ('one', 'two'))
As shown above, the two “%” symbol indicates that there are two placeholders waiting to be replaced in the String. The next parentheses provides two String objects which acts as the objects which replaces the placeholders. We will put the above command in a Python file and simply run it on the command line. Here is how we do it:
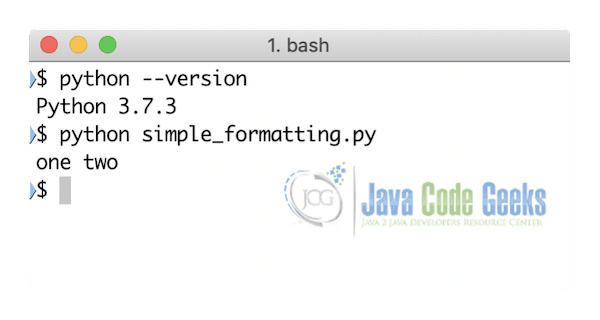
We just checked our Python version so that there is no confusion in Python version being used because there is a difference in how Strings are formatted in older Python version, which we will not look at in this lesson. Usually, this is one of the easiest and straightforward way to format Strings. Actually, not just Strings, we can even format Lists the same way:
my_list = [1, 2, 3] print('List is: %s' % my_list)
We will put the above command in a Python file and simply run it on the command line. Here is how we do it:
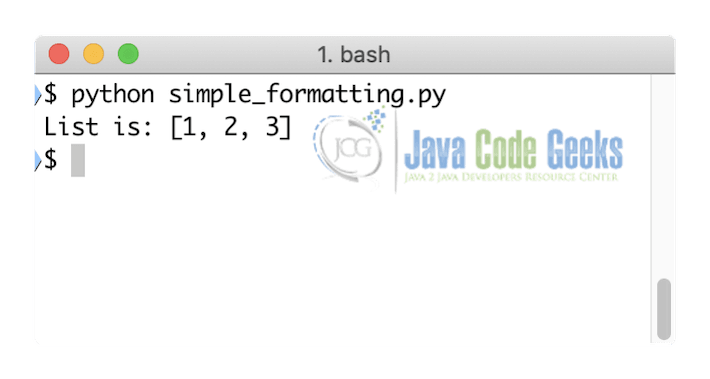
2.2 Formatting Numeric values
The formatting for numeric values is a lot similar to that of Strings. We will look at two examples here, the first one will be a lot similar to the previous example we saw. The second one will reflect the new conventions beings used now. Here is how we do it:
print('%d %d' % (45, 57))
print('{} {}'.format(45, 57))
In the above code, we used a little different placeholder than we used for String values. In the second line of code, we used a simple Python function to format the passed values in places of placeholder parentheses. Let’s run the above code now:
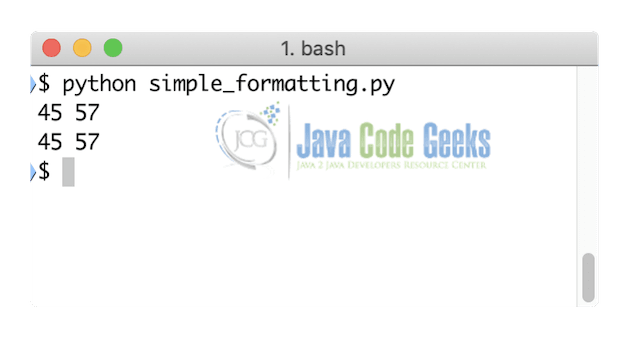
There are two ways in which we formatted the numeric values above. Note that ‘%d’ will only work with integer values and not the values with decimal places. Let’s see how to format floating point values in Python:
print('%.2f %.2f' % (45.22, 57.32))
print('{} {}'.format(45.22, 57.32))
In above code snippet, the main placeholder is ‘%f’ and the digit before that just informs Python to keep two decimal values in the numeric value passed as the arguments. Here is the output we will get when we run the above code snippet:
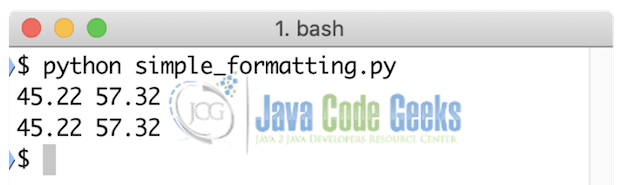
One thing to notice is that the second example, where we used curly braces remains the same irrespective of the data type being formatted. So, using curly braces is one of the safest options while formatting values.
2.3 Padding and aligning strings
Be default, formatted strings only take up space which is enough for the provided values but sometimes, we need to add some alignment or spaces before or after the values as well. The default alignment differs in old and new style of formatting. The old style defaults to right aligned while for new style it’s left. Here is the sample code snippet to align a String to the right:
print('%5s' % ('JCG'))
print('{:>5}'.format('JCG'))
Here is the output we will get when we run the above code snippet:
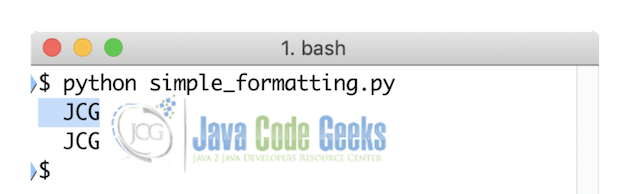
In a very similar fashion, here is the sample code snippet to align a String to the left:
print('%-5s' % ('JCG')) print('{:<5}'.format('JCG'))
Here is the output we will get when we run the above code snippet:
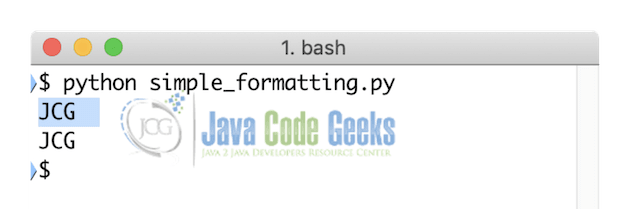
In above output, we show how spaces are added at the end of the String while in the earlier example, it was added at the beginning of the String.
2.4 Formatting numbers with symbol
In Python, only negative numbers are shown with a hyphen symbol and positive numbers are shown without an intent. Well, this can be changed with a formatting technique as well. Let’s try a simple code snippet for the same:
print('{:+d}'.format(23))
Here is the output we will get when we run the above code snippet:
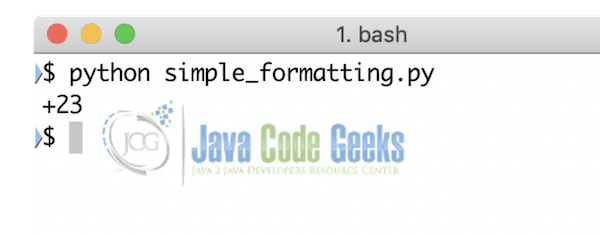
2.5 Named Placeholders
Just like what we showed in the first section where placeholders were very simple, the placeholders can become difficult to manage very easily when the text size starts to grow. To avoid the mess, we can make use of a simple Python dictionary to manage placeholders as keys and original text as values. Let’s try a simple code snippet for the same:
language = { 'one' : 'Java', 'two' : 'Python', 'three' : 'Kotlin' }
Next, we will put this Python dictionary to use in a print statement with newer statements:
print('{one} is better than {two} which might not be better than {three}'.format(**language))
Here is the output we will get when we run the above code snippet:
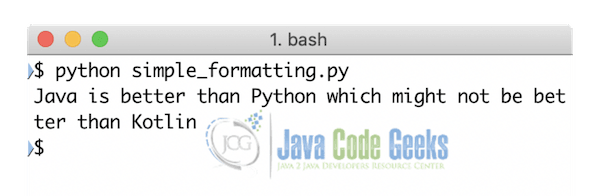
Named Placeholders offer us a very clear advantage. We don’t have to remember their index and we can reuse each placeholder any number of times we need.
3. Python Formatting Strings – Conclusion
In this lesson, we checked very simple examples of how we can format String and numbers in Python. We tried to describe a newer version of formatting methodologies which are much more intuitive than the older version of formatting methodologies.