Angular Child Routes Example
Welcome readers, in this tutorial, we will learn the basic concept behind Routing and Child Routes in angular.
1. Introduction
Routing in angular is an essential part of the angular framework contained in the @angular/router
package. It,
- Helps developers build single page applications with multiple states and views using routes and components
- Allows client-side navigation and routing between various components
- Allows lazy loading of modules
- Add router guards for client-side protection and allow or disallow access to the components or modules
- Various path matching strategies (i.e. prefix and full) to tell the router how to match a specific path to a component
- Easy access to route and query parameters
1.1 Routes and Paths in Angular Routing
In angular, a route is an object that provides information about which component maps to a specific path. A path is a fragment of a url that determines where exactly the resource is located the user wants to access. In angular, developers can define a route using route configurations or instance of the Router interface. Each route has the following properties i.e.
path
: Specifies the path of the routepathMatch
: Specifies the matching strategy (i.e. prefix or full)component
: Specifies the component that is mapped to a routeredirectTo
: Url which users will be redirected to if a route is a matched
For instance,
{ path: 'products', component: ProductlistComponent }
1.2 Router Outlet
The router-outlet
tag in angular is a directive exported by the RouterModule
and acts as a placeholder to the router where it needs to insert the matched components. It is represented by the following syntax.
<router-outlet></router-outlet>
Always remember, an angular router can support more than one outlet in the same application. The main outlet is called as a primary outlet and other outlets are called secondary outlets. Now open the visual studio code and let us see how to implement this tutorial in angular 6 frameworks.
2. Angular Child Routes Example
Here is a systematic guide for implementing this tutorial.
2.1 Tools Used
We are using Visual Studio Code and Node Terminal to compile and execute the angular code on a browser.
2.2 Project Structure
In case you are confused about where you should create the corresponding files or folder, let us review the project structure of the angular application.
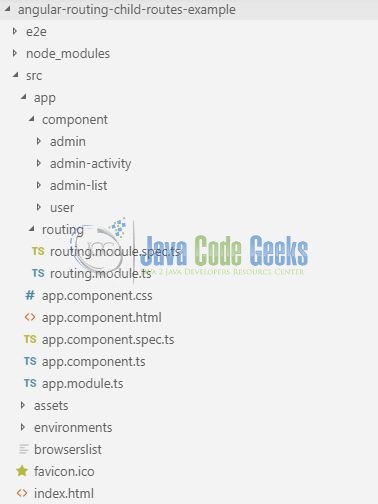
3. Creating Angular application
Run the ng new angular-routing-child-routes-example
command in the npm console to create a new angular project. Once the new project is created, following the below steps.
3.1 Import Reactive forms module
To start working with angular routing will need to import the RoutingModule
module in src/app/app.module.ts
file.
app.module.ts
// Injecting the routing module. import { RoutingModule } from './routing/routing.module'; // Declaring the routing module in the imports section of NgModule decorator. imports: [ BrowserModule, RoutingModule ],
3.2 Injecting Router Outlet in the Application module
To activate routing in the angular application, inject the routing outlet in src/app/app.component.html
file.
app.component.html
<div class="container"> <h2 align="center" class="text-info">{{ title }}</h2> <hr /> <!-- To activate the routing in the angular application. --> <router-outlet></router-outlet> </div>
3.3 Creating Components
To understand child routing in angular we will create some components. Execute the following commands in the npm console.
// Administrator component. ng generate component component/admin // Child components of Administrator module. ng generate component component/admin-activity ng generate component component/admin-list // User component. ng generate component component/user
Following commands will generate the sample components to better understand the child routing in angular. If developers have any confusion regarding the project structure, they can refer to Fig. 1. Do remember to include the router-outlet in the src/app/component/admin/admin.component.html
file to include the children components of the administrator component.
3.4 Creating a Routing module
Run the ng g m routing
command in the npm console to create a routing module. Add the following code to the src/app/routing/routing.module.ts
file.
routing.module.ts
import { NgModule } from '@angular/core'; import { Routes, RouterModule } from '@angular/router'; // Administrator component. import { AdminComponent } from '../component/admin/admin.component'; // Child components of Administrator module. import { AdminListComponent } from '../component/admin-list/admin-list.component'; import { AdminActivityComponent } from '../component/admin-activity/admin-activity.component'; // User component. import { UserComponent } from '../component/user/user.component'; // Configuring the routing paths. const routes: Routes = [ { path: '', redirectTo: '/user', pathMatch: 'full' }, { path: 'user', component: UserComponent }, { path: 'admin', component: AdminComponent, children: [ { path: 'list', component: AdminListComponent }, { path: 'activity', component: AdminActivityComponent } ] } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule], declarations: [] }) export class RoutingModule { }
4. Run the Application
As we are ready with all the changes, let us compile and run the angular application with ng serve
command. Once the projects are successfully compiled and deployed, open the browser to test it.
5. Project Demo
Open your favorite browser and hit the angular application url (http://localhost:4200/
) to display the index page of the application.

Change the browser url to http://localhost:4200/admin
to display the administrator interface.
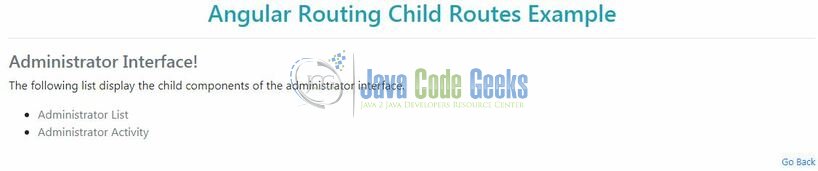
Developers can click the link present in the admin interface to display the child components within the administrator interface.
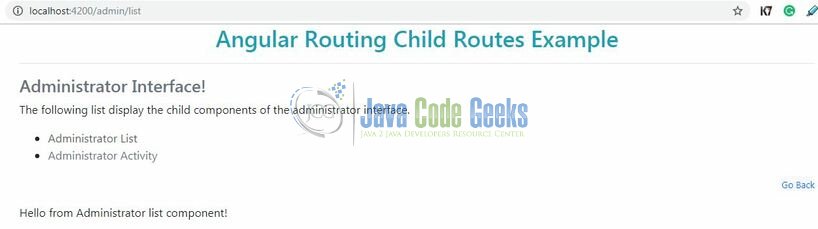
That is all for this tutorial and I hope the article served you whatever you were expecting for. Happy Learning and do not forget to share!
6. Conclusion
In this section, we learned how to create a simple angular routing application and understand the child routing in angular. Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was a tutorial of Child Routing in the angular framework.
You can download the full source code of this example here: Angular Child Routes Example