Core Java Tutorials
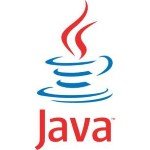
In this detailed Resource page, we feature an abundance of Core Java Tutorials!
Java is a general-purpose computer-programming language that is concurrent, class-based, object-oriented, and specifically designed to have as few implementation dependencies as possible. It is intended to let application developers “write once, run anywhere” (WORA), meaning that compiled Java code can run on all platforms that support Java without the need for recompilation.
Java applications are typically compiled to bytecode that can run on any Java virtual machine (JVM) regardless of computer architecture. As of 2016, Java is one of the most popular programming languages in use, particularly for client-server web applications, with a reported 9 million developers.
The latest version is Java 11, released on September 25, 2018, which follows Java 10 after only six months, being in line with the new release schedule. Java 8 is still supported but there will be no more security updates for Java 9. Versions earlier than Java 8 are supported by companies on a commercial basis; e.g. by Oracle back to Java 6 as of October 2017 (while they still “highly recommend that you uninstall” pre-Java 8 from at least Windows computers).
If you wish to build up your Core Java knowledge first, check out our 150 Java Interview Questions and Answers – The ULTIMATE List
Core Java Tutorials – Java Basics
Get familiar with Java with some basic examples
- Java for loop Example
If you need to execute a block of code many times, then you will definitely have to use a mechanism named as loop.
Java provides three looping mechanisms, which are the following: while Loop, do-while Loop, for Loop - Java Switch-Case Example
In this post, we feature a comprehensive Java Switch-Case Example. Java provides decision making statements so as to control the flow of your program. These statements are: if…then, if…then…else, switch..case - Java Abstract Class Example
In this tutorial we will discuss about the Java Abstract Class. An abstract class is a class that is declared using the abstract keyword. An abstract class cannot be instantiated. It can be used only as a super-class for those classes that extend the abstract class. - Encapsulation in Java
In this tutorial we will discuss about Encapsulation in Java. Encapsulation is the mechanism for restricting access to an object’s components. It aims for high maintenance and handling of the application’s code. - Transient variables in Java
In this post we are going to examine what a transient variable is in Java and learn how to use them in the right context. In order to do that we are going to also take a quick look into the Serializable interface and its usefulness. - Java Inheritance example
In this tutorial we will discuss about the inheritance in Java. The most fundamental element of Java is the class. A class represents an entity and also, defines and implements its functionality. In Java, classes can be derived from other classes, in order to create more complex relationships. - java.library.path – What is it and how to use
In this tutorial we will discuss about java.library.path, its definition and how can be used by Java applications. The Java Virtual Machine (JVM) uses the java.library.path property in order to locate native libraries. This property is part of the system environment used by Java, in order to locate and load native libraries used by an application. - Java Nested (Inner) Class Example
In this example we are going to explain what exactly nested classes are in Java. We are going to present the different categories of nested classes and their sub-types, as well as ways to use them in your program. - Java Keystore Tutorial
Who of us didn’t visit ebay, amazon to buy anything or his personal bank account to check it. Do you think that those sites are secure enough to put your personal data like (credit card number or bank account number, etc.,)? - Java instanceof Example – How to use instanceof
In this example we will show how to use the operator instanceof in Java.This operator is a Type Comparison Operator and can be used when we want to check if an object is an instance of a specific class, an instance of a subclass, or an instance of a class that implements a particular interface. - Java deployment toolkit (JDT) – How to use it
The Java Deployment Toolkit allows developers to easily deploy their applets and applications to a large variety of clients. The Deployment Toolkit script is a set of JavaScript functions that, help developers deploy their rich Internet applications (RIAs) consistently across various browsers and operating system configurations. - Java 8 Default Methods Tutorial
In this article we are going to explain how to use and take advantage of the possibility to implement default methods in interfaces. This is one of the most important features that are available since Java update 8. - Java 8 Optional Example
In this article we are going to show how to use the new java.util.Optional class. The null reference is a very common problem in Java, everybody got once a NullPointerException because some variable or input parameter was not properly validated. - Java XML parser tutorial
In this tutorial we will discuss about XML parsers in Java. XML is a markup language that defines a set of rules for encoding documents. Java offers a number of libraries in order to parse and process XML documents. An XML parser provides the required functionality to read and modify an XML file.
Core Java Tutorials – Data Types, Maps and Arrays
Learn how to use the Java Data Types, Maps and Arrays
- Java Best Practices – Vector vs ArrayList vs HashSet
We are going to perform a performance comparison between the three probably most used Collection implementation classes. To make things more realistic we are going to test against a multi–threading environment so as to discuss and demonstrate how to utilize Vector, ArrayList and/or HashSet for high performance applications. - Java Best Practices – Queue battle and the Linked ConcurrentHashMap
Continuing our series of articles concerning proposed practices while working with the Java programming language, we are going to perform a performance comparison between four popular Queue implementation classes with relevant semantics. - Java.util.TreeMap Example
In this example we will see how and when to use java.util.TreeMap. A TreeMap is a Map implementation which provides total ordering on its elements. The elements are ordered using their natural ordering, or by a Comparator typically provided at sorted map creation time. - Java.util.EnumMap Example
In this example we will see how and when to use Java.util.EnumMap. EnumMap is used when we need to have keys of the map as enums. EnumMaps are represented internally as arrays. This representation is extremely compact and efficient. - ArrayList in Java Example – How to use arraylist
In this example we will show how to use ArrayList in Java. The class java.util.ArrayList provides resizable-array, which means that items can be added and removed from the list. It implements the List interface. A major question related to arraylists is about when to use arraylists instead of arrays and vice versa. - Java LinkedList Example
The LinkedList class can be considered as an alternative to ArrayList class. It extends AbstractSequentialList and implements the List interface, with a doubly-linked list. Also, the LinkedList allows the usage of iterators, so that you can iterate the list forwards or backwards and declare the exact position of the starting node. - Java Hashmap Example
HashMap is a member of the Java Collection Framework and is a very common way to collect and retrieve data. HashMap represents a data structure that offers key-value pairs storing, based on hashing. In this example we are going to show how we can create a simple HashMap and a HashMap. - Java String Class Example
In this example we are going to discuss about the basic characteristics of Java String Class. String is probably one of the most used types in Java programs. That’s why Java provides a number of API methods that make String manipulation easy and efficient, straight out of the box. - Java String format Example
In this post, we feature a comprehensive Java String format Example. We are going to see how to format Strings in Java. Developers familiar with C, will find the methods used here resembling printf function. And the formatting specifications are very similar. - Java Best Practices – String performance and Exact String Matching
We are going to talk about String performance tuning. We will focus on how to handle String creation, String alteration and String matching operations efficiently. Furthermore we will provide our own implementations of the most commonly used algorithms for Exact String Matching. - Java String Array Example
In this example we will show how to declare and populate a Java String Array as well as how to iterate through all its elements. Example of Java String Array. - Java String Compare Example
We are going to focus on how you can compare Strings in Java. By comparing, in this case, we mean to check if their values are equal. In the previous example we talked generally about the Java String Class. - Java String CompareTo Example
But consider the case when you have a collection of Strings and you want to sort it. Of course, equality check is not enough. You have to impose ordering somehow. In strings (words in general), you can use lexicographical ordering. - Java String Contains Example
In this example we are going to see how you can check if a String contains another String in Java. The Java String Class API offers a method to do that, contains. To be more specific the complete signature of contains method is public boolean contains(CharSequence s). - Java String Concatenation Example
In this example we are going to see how you can concatenate two Strings, or in fact, any Object with a String. As we’ve mention in an introductory article about Java String Class, String is one of the most used types in Java programs. So Java creators wanted to make the usage of Strings as straightforward as possible. - Java String length Example
In this example we are going to see how you can find out the length of the a String in Java. By length we mean that number of Unicode characters or Code Units. String class has a very convenient method of doing so: length(). - Java String matches Example
In this example we are going to talk about matches String Class method. You can use this method to test a String against a regular expression. Testing a String against a regular expression is a very common operation for interactive applications, as it is heavily used to perform validity checks on user input. - Java String split Example
In this example we are going to see how to split a String in Java into smaller sub strings. It is extremely common to want to split a String into parts. These parts are separated by a specific delimiter. And in order to avoid parsing the String yourself, Java offers split API method. - Java String Replace Example
In this example we will show how to modify a string by replacing either characters or sequences of characters included in the string with different ones. - Java String replaceAll example
In this example we are going to see how to use replaceAll String class API method. With replaceAll, you can replace all character sequences and single characters from a String instance. As you know, String objects are immutable. - Java Best Practices – Char to Byte and Byte to Char conversions
This article concludes with a performance comparison between two proposed custom approaches and two classic ones (the “String.getBytes()” and the NIO ByteBuffer) for converting characters to bytes and vice – versa.
Core Java Tutorials – Algorithms
Learn how to use famous Algorithms in Java
- Quicksort algorithm in Java – Code Example
In this article, we will talk about how you can implement in Java one of the most famous and useful sorting techniques: Quicksort. Quicksort is the most widely used sorting algorithm. - Mergesort algorithm in Java – Code Example
In this article, we will discuss about the Mergsort algorithm. The Mergersort algorithm is much more efficient than some of the other sorting algorithms. The Mergesort works by sorting and merging two arrays into one. - Java Recursion Example
Recursion is a method of solving a problem, where the solution is based on “smaller” solutions of the same problem. In most programming languages (including Java) this is achieved by a function that calls itself in its definition. - Factorial Program in Java
In this example we are going to talk about a classic programming task, as we are going to create a Java program that computes the factorial of a non negative integer. - Palindrome Program in Java
In this example we are going to see how you can create a simple Java Application to check weather a String is a palindrome. A String is considered a palindrome if it can be similarly read both from left to right and from right to left. - Java Prime Numbers Example
In this example we are going to talk about prime numbers. Prime numbers are one of the most important subsets of physical numbers. A positive integer p > 1 is a prime if and only if its positive divisors are only itself and 1.
Core Java Tutorials – Classes, Methods, Objects and Libraries
Learn the most usable Classes, Methods, Objects and Libraries of Java
- Java Best Practices – DateFormat in a Multithreading Environment
This is the first of a series of articles concerning proposed practices while working with the Java programming language. All discussed topics are based on use cases derived from the development of mission critical, ultra high performance production systems for the telecommunication industry. - java.util.Date Example – How to use Date
In this example we will show how to use java.util.Date class. Class Date represents a specific instant in time, with millisecond precision. - Java 8 Date/Time API Tutorial
In this article we are going to explain the main features of the new Date/Time API coming with Java 8. We are going to briefly explain why a new Date/Time API is necessary in Java and what benefits it has in comparison with the “old” world. - java.util.PriorityQueue Example
In this example, we shall demonstrate how to use the java.util.PriorityQueue Class. The PriorityQueue Class implements the contract defined through the Queue interface. The PriorityQueue is like other collections as in, it is unbounded and we can specify the starting size. - java.util.Hashtable Example
In this example, we will show the range of functionality provided by the java.util.Hashtable class. Hashtable was part of the original java.util and is a concrete implementation of a Dictionary. However, with the advent of collections, Hashtable was reengineered to also implement the Map interface. - Java.util.TreeSet Example
In this example we will see how and when to use java.util.TreeSet. A TreeSet is a set implementation which provides total ordering on its elements. The elements are ordered using their natural ordering, or by a Comparator typically provided at sorted set creation time. - Java Best Practices – High performance Serialization
Continuing our series of articles concerning proposed practices while working with the Java programming language, we are going to discuss and demonstrate how to utilize Object Serialization for high performance applications. - Serialization in java
Java provides mechanism called serialization to persists java objects in a form of ordered or sequence of bytes that includes the object’s data as well as information about the object’s type and the types of data stored in the object. - Do it short but do it right !
Writing concise, elegant and clear code has always been a difficult task for developers. Not only will your colleagues be grateful to you, but you would also be surprised to see how exciting it is to constantly look forward to refactoring solutions in order to do more (or at least the same) with less code. - Substring Java Example
In this post, we feature a comprehensive Substring Java Example. We will show how to use Java String substring() API method. substring() method can be expressed in two ways: String substring(int start, int end) and String substring(int start). - Java 8 Lambda Expressions Tutorial
A Lambda, in general, is a function that expects and accepts input parameters and produce output results (it may also produce some collateral changes). Java offers Lambdas as one of its main new features since Java 8. - Apache Commons IO Tutorial: A beginner’s guide
In this example, we are going to present some methods with varying functionality, depending on the package of org.apache.commons.io that they belong to. - org.apache.commons.io.FileUtils Example
In this example we are going to show some of the capabilities of the FileUtils class, which is a part of Apache Commons IO. The methods implemented in this class are all about file manipulation, and in many cases they make a developer’s life much easier. - Delete File in Java Example
In this example we are going to see how to delete a file in Java. Of course Java offers a very convenient API to perform deletion and creation. Most of them are placed in File class. We are going to use delete() methods that deletes the file or directory. If the file is deleted successfully, the methods returns true, else false. - Java 8 Stream API Tutorial
Java 8 offers several new functionalities. One of the most important is the new Streams API. Basically, Streams are sequences of elements that support concatenated operations. They used a source and allow different intermediate and terminal operations. - Java ImageIO – Write image to file
This is an example of how to write an image to a file, making use of the ImageIO utility class of Java. ImageIO class of javax.imageio package, provides methods to locate ImageReaders and ImageWriters, to perform encoding and decoding and other methods for image processing. - org.apache.commons.io.IOUtils Example
In this example we are going to elaborate the use of the IOUtils class in the package: ‘org.apache.commons.io’, as the package name says it is a part of Apache Commons IO. All members functions of this class deals with Input – Output streams Manipulations, and it really helps to write programs which deals with such matters. - org.apache.commons.io.FilenameUtils Example
Apache Commons IO is a library of utilities to assist with developing IO functionality. org.apache.commons.io package has utility classes to perform common tasks. FilenameUtils is one of the classes. This class has static methods for filename and filepath manipulation. - Java 8 Lambda Expressions Tutorial
In this article we are going to explain what Lambdas are, why are they important and how do they look like in Java. We are going to see also a couple of examples and real life applications. - Getting Started With Google’s HTTP Client Library for Java
Google’s HTTP Client Library for Java is a one-stop shop for all your HTTP client needs, irrespective of the Java platform (application server, Android, App Engine etc.). It offers a well-designed abstraction layer over a number of lower level HTTP client implementations (we’ll talk more about this later). - Java Convert Csv to Excel File Example
In this tutorial, we are going to implement the Csv to Excel file conversion by using the Apache POI library. This tutorial will show developers how to write large data to an excel file using SXSSF. - Java Annotations Tutorial
Annotations in Java are a major feature and every Java developer should know how to utilize them. In this article we are going to explain what Java annotations are, how they work and what can be done using annotations in Java. - Abstraction in Java
Abstraction occurs during class level design, with the objective of hiding the implementation complexity of how the the features offered by an API / design / system were implemented, in a sense simplifying the ‘interface’ to access the underlying implementation. - Java Reflection Tutorial
Reflection is a feature in the Java programming language. It allows an executing Java program to examine or “introspect” upon itself, and manipulate internal properties of the program. For example, it’s possible for a Java class to obtain the names of all its members and display them. - Reactive Java (RxJava) Tutorial: Introduction
Reactive Java or RxJava is an implementation and enhancement of the observer pattern. It was intended for use in event driven schemes where nesting synchronous or asynchronous callback methods becomes overly complex. - Reactive Java (RxJava) Tutorial: Advanced
This article will examine asynchronous or concurrency tasks that are made easier with RxJava. The concurrency problems that RxJava is suitable to solve and we will look at in this example include: Nested callbacks, Making asynchronous calls, Aggregating or combining asynchronous calls and Streaming - Java Performance Tuning Tutorial
Java application performance tuning is a complex subject. There are many articles and books dedicated on the details and research of performance tuning. There is no silver bullet and often fixing performance issues is a long and tedious process. This article attempts to suggest a few pointers to get started with performance tuning and discusses one tool for profiling Java applications. - JVM Architecture: Overview of JVM and JVM Architecture
In this tutorial, we will understand and learn the Java Virtual Machine (JVM) and its architecture. This tutorial will help you to correctly answer the below questions: What is JVM in Java?, Different components of JVM and Difference between JVM, JRE, and JDK. - JVM Architecture: JVM Class loader and Runtime Data Areas
This tutorial will help developers to correctly answer the questions on below topics: ClassLoader Subsystem and Runtime Data Areas. - JVM Architecture: Execution Engine in JVM
This tutorial will help developers to correctly understand the Execution Engine in JVM. - ByteCode primer for Java Class Files
In this article we will kick start our adventures in Java byte code. Byte code makes it possible for Java applications to run on varying hardware architectures. Very often we ignore the byte code layer. Understanding it just a little, can go a long way to help us write better Java code. - TestNG Configuration Annotations Example
In this example we shall show you the TestNG Configuration Annotations. TestNG is a testing framework designed to simplify a broad range of testing needs, from unit testing to integration testing. - TestNG beforeTest Example
In this article, I will show you an example of the TestNG @beforeTest annotation. In TestNG, you can configure your tests using annotations that start with @Before or @After. TestNG defines many configuration annotations, each one belonging to a specific event in its lifecycle. - TestNG beforeSuite Example
In this article, we will show you how to use the TestNG @BeforeSuite annotation. If you have used JUnit annotations, you will be familiar with @BeforeClass. In TestNG, apart from @BeforeClass, we also have additional annotations like @BeforeTest and @BeforeSuite. - TestNG beforeMethod Example
In this article, I will show you an example of TestNG @BeforeMethod. This is one of the annotations provided by TestNG that gets invoked before the execution of each test method. It is very similar to JUnit’s setUp() and is useful if you want to setup some test data before the start of the test method. - TestNG DataProvider Example
In this article, I am going to show you some examples of DataProvider. It is one of the methods used in TestNG to support data-driven testing. Before I proceed with my examples, I will brief you on data-driven testing. - TestNG Listeners Example
This article aims to introduce you to TestNG listeners and show you an example for each of the listeners. In TestNG, a listener is represented by the marker interface org.testng.ITestNGListener. - Gradle “Hello World” Tutorial
In this post we’ll look at Gradle, its installation and configuration, and how to automate stages of development and release of software through its base concept, the Gradle tasks. Gradle is a build and automation tool, that can automate our building, testing, deploying tasks and many more. - Gradle SourceSets Example
Gradle SourceSets are a key concept for the Gradle Java Plugin which define the structure of Java Source Files. In this example will see how to use this concept, customize them through gradle properties, create a new sourceset, get documentation and assembling them in a JAR. - Gradle Properties: Build and Configuration Example
In this detailed Gradle Properties tutorial, we shall see how to access several default properties in Gradle, and how to set our custom properties. Also, what methods are to set these properties and how Gradle processes them. - Gradle Wrapper Example
In this example, we will understand how Gradle can be used to build projects even if the developer machine doesn’t have Gradle installed, by using Gradle Wrapper. This is a best practice to unify the Gradle version used by the entire development team. - An Introduction to Apache Lucene for Full-Text Search
Lucene is an open-source project that provides Java-based indexing and search technology. Using its API, it is easy to implement full-text search. - “Did you mean” feature with Apache Lucene Spell-Checker
This feature can be implemented in Java using Lucene project and this tutorial will show you how. The implementation will be based on one of Lucene’s sub-projects, named SpellChecker (see spell checker API). - Debugging a Production Server – Eclipse and JBoss showcase
Do you write code that has bugs? No, of course not. For the rest of us, mere mortals, who do write code with bugs, I would like to address a very sensitive issue: debugging an application that runs on a production server. - Findbugs Eclipse Example
In this tutorial, we will learn to install the FindBugs Eclipse Plugin and use it for code analysis within Eclipse. As most of us know, FindBugs is a static code analyser which operates on Java bytecodes, and helps identify a range of potential errors / bad code practice in Java programs. - GPGPU Java Programming
Before we dive into coding some background. There are two competing GPGPU SDKs: OpenCL and CUDA. OpenCL is an open standard supported by all GPU vendors (namely AMD, NVIDIA and Intel), while CUDA is NVIDIA specific and will work only on NVIDIA cards. - GPGPU with Jcuda the Good, the Bad and … the Ugly
In this article we will see how we can utilize a GPU do what is doing best: parallel processing. Through this example we will take some metrics and see where GPU processing is stronger or weaker than using a CPU …and of course as the title suggests there is an ugly part at the end.
Core Java Tutorials – Exceptions Handling and Errors
Simple examples on how to handle Exceptions and Errors in Java
Check out this Java Exception Handling Tutorials page to find examples and learn more.
Core Java Tutorials – Advanced Java
Become a great Java developer by studying Java’s more advanced concepts
Advanced Java Course
This course is designed to help you make the most effective use of Java. It discusses advanced topics, including object creation, concurrency, serialization, reflection and many more. It will guide you through your journey to Java mastery!
Check out this Advanced Java Tutorial course to help you make the most effective use of Java.
Java Servlet
A Java software component that extends the capabilities of a server. Although servlets can respond to any types of requests they most commonly implement web containers for hosting web applications on web servers and thus qualify as a server-side servlet web API. Such web servlets are the Java counterpart to other dynamic web content technologies such as PHP and ASP.NET.
Check out this Java Servlet Tutorials page to help you build up your Java Servlet knowledge.
XPath
A query language for selecting nodes from an XML document. In addition, XPath may be used to compute values (e.g., strings, numbers, or Boolean values) from the content of an XML document.
Check out this XPath Tutorials page to help you build up your XPath knowledge.
More Java advanced concepts
Core Java Tutorials – JVM
Learn what is Java Virtual Machine (JVM) and what are its basic components
Core Java Tutorials – Java NIO
Learn how to handle I/O and networking transactions with Java NIO library
Check out this Java NIO Tutorials page to find examples and build your Java NIO knowledge.
Core Java Tutorials – JDBC
Examples on the Java Database Connectivity (JDBC)
Check out this JDBC Tutorials page to find examples and build up your Java Database Connectivity knowledge.
Core Java Tutorials – Java Logging
Learn how to create logs in a Java program using Java logging frameworks like SLF4J, Logback, Log4j and more
Check out this Java Logging Tutorials page to find examples and build up your Java Logging knowledge.
Core Java Tutorials – Scheduling
Learn how to use Quartz Scheduler, a Java library for job scheduling
Check out this Quartz Tutorials page to find examples and build up your Quartz knowledge.
Core Java Tutorials – Multi threading and Concurrency
Learn programming on Java using Multithreading and Concurrency techniques
Check out this Java Concurrency Tutorials page to find examples and build up your Java Concurrency knowledge.
Core Java Tutorials – Testing
Learn a variety of Testing tools and select which one is most suitable for your Java project
JMeter
An Apache project that can be used as a load testing tool for analyzing and measuring the performance of a variety of services, with a focus on web applications.
Check out this JMeter Tutorials page to find examples and build up your JMeter knowledge.
Selenium
A portable software-testing framework for web applications. It provides a playback (formerly also recording) tool for authoring tests without the need to learn a test scripting language (Selenium IDE).
Check out this Selenium Tutorials page to find examples and build up your Selenium knowledge.
JUnit
A unit testing framework for the Java programming language. JUnit has been important in the development of test-driven development, and is one of a family of unit testing frameworks which is collectively known as xUnit that originated with SUnit.
Check out this JUnit Tutorials page to find examples and build up your JUnit knowledge.
Mockito
An open source testing framework for Java released under the MIT License. The framework allows the creation of test double objects (mock objects) in automated unit tests for the purpose of test-driven development (TDD) or behavior-driven development (BDD).
Check out this Mockito Tutorials page to find examples and build up your Mockito knowledge.
TestNG
Simple examples on the TestNG testing framework
Core Java Tutorials – Build and Automation Tools
Gradle
Simple examples on Gradle Build Automation Tool for Java
Core Java Tutorials – Design Patterns
Simple examples to learn Java Design Patterns
Check out this Java Design Patterns Tutorials page to find examples and learn more about Java Design Patterns.
Core Java Tutorials – Java Versions
Check some of the latest versions of Java language
Java 11
Check out these Java 11 articles to enhance your Java knowledge with the new features.
Java 9
Java 9 should include better support for multi-gigabyte heaps, better native code integration, a different default garbage collector (G1, for “shorter response times”) and a self-tuning JVM. In early 2016, the release of Java 9 was rescheduled for March 2017, later again postponed four more months to July 2017, and changed again to be finally available on September 21, 2017, due to controversial acceptance of the current implementation of Project Jigsaw by Java Executive Committee, which led Oracle to fix some open issues and concerns, and to refine some critical technical questions.
Check out this Java 9 Tutorials page to find examples and build up your Java 9 knowledge.
Java 8
Java 8 (codename: Spider) was released on March 18, 2014, and included some features that were planned for Java 7 but later deferred.
Work on features was organized in terms of JDK Enhancement Proposals (JEPs).
Check out this Java 8 Tutorials page to find examples and build up your Java 8 knowledge.
Core Java Tutorials – Applets and Other Java Related Topics
[undereg]