ReactJS onClick Example
In this example, we will see an example of the ReactJS onClick event. We will discover ways to handle the click event of HTML elements using ReactJS. As you know ReactJS has soared in popularity. And has become one of the most favored libraries for front end development. We explore how to attach event handlers and handle the click event of various HTML elements. In the process, we explore various nuances around it.
So let us get started and build a simple application to demonstrate how to handle the click event.
1. Tools and Frameworks
We use the following toolset and frameworks to build a simple ReactJS application for this example
create-react-app is a package to quickly generate a ReactJS application. Moreover, it uses the latest version of ReactJS. Also, I have used my favorite editor Visual Studio Code although you are free to follow along in one of your choices.
2. Generating ReactJS Application
We run the following command to generate our starting application.
> npx create-react-app my-app
npx comes with Nodejs and basically downloads a temporary copy of packages and executes them with the provided parameters. This prevents pollution of the global namespace with rarely used packages. Once the above command completes we should have a project structure that looks like below:
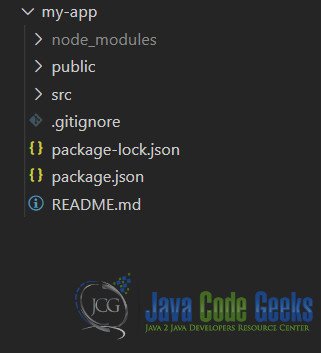
3. Event Handling
Let us build a simple component with a couple of HTML tags. We will use this component to learn how to handle the click event. The component displays a Button and an anchor tag. We will handle the click event of both these tags. To handle the click event of an HTML tag we pass an event handler method to the onClick property of the tag like below:
... <a href="#" onClick={handleLinkClick}>Click Me!</a> <button onClick={handleButtonClick}>Click Me!</button> ...
The code for our component looks like below:
ClickDemo.js
import React from 'react'; function ClickDemo(props) { const handleLinkClick = (event) => { event.preventDefault(); alert('Link Clicked!'); } const handleButtonClick = ({ target }) => { target.innerText = "Clicked"; alert('Button Clicked!'); } return <> <div style={{ flexDirection: 'column', height: '100vh', display: 'flex', alignItems: 'center', justifyContent: 'center' }}> <a onClick={handleLinkClick} href="http://google.com">Go to Google</a> <button onClick={handleButtonClick}> Click Me!</button> </div> </>; } export default ClickDemo;
As you can see in the code above. We prevent the default navigation to http://google.com on the click of the anchor tag. Also, we show an alert with the message Link Clicked!. Similarly, on the click of the button we change the text of the button to “clicked” apart from showing an alert box.
4. Running the Application
We run the application using below command:
>npm start
This command opens the browser and displays our component as below:
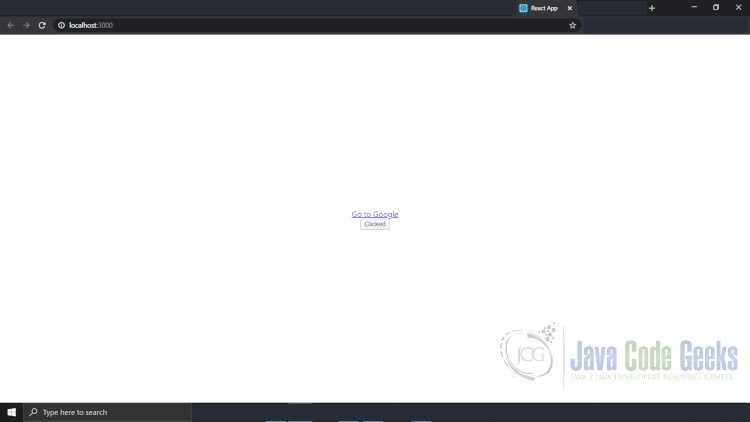
5. Download the Source Code
You can download the full source code of this example here: ReactJS onClick Example