ReactJS with Ajax Requests Example
In this post, we feature a comprehensive article about ReactJS with Ajax Requests.
In this article, we will have a look at making Ajax requests in a ReactJS application. We will create a simple application where the user can type in a few characters and we will pass these on to the server in an Ajax request. The server, in turn, will return names of countries with those sequences of characters in there name or code. We will display these as an unordered list on our landing page.
1. Introduction
We will create the ReactJS application using the create-react-app utility. For the server-side code, we use express as our webserver to expose an API to call from the client using Ajax Request. But do not worry, you do not need to know a lot about the ongoings on the server side since we will focus on the client-side logic. This logic remains the same regardless of the server-side technology.
2. Initial Setup
Without further delay let us get started and set up our sample app for this example. Firstly we run the following command to generate our ReactJS application.
> npx create-react-app my-app .
You can think of npx as a tool to download temporarily required tools and run them. They are disposed of once done. This prevents pollution of our global namespace. Once the command above completes our generated project structure should look like below:
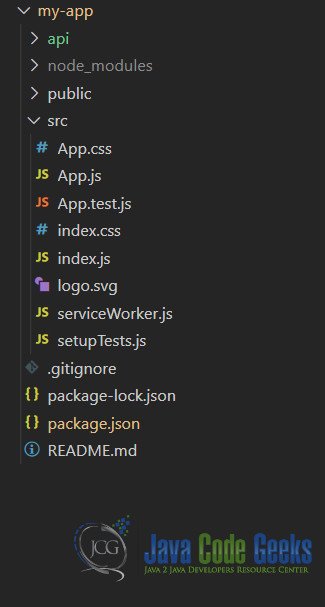
To set up our server-side code we will leverage express and the cors package. Our server-side code consists of the two files, viz., index.js and countryController.js. The code looks like below, though we need not deep dive into it for the purposes of this example. Suffice it to say that it returns a list of countries with their codes which match the text user types in.
index.js
const express = require('express'); const app = express(); const bodyParser = require("body-parser"); const api = require('./countryController'); const cors = require('cors'); app.use(bodyParser.json()); app.use(bodyParser.urlencoded({ extended: true })); app.use(cors()); app.use('/api', api()); const Port = process.env.PORT || 2019; app.listen(Port, () => { console.log(`Listening on ${Port}.`); });
countryController.js
const router = require('express').Router(); module.exports = () => { router.get('/country/:code?', (req, res) => { let code = req.params.code || ""; if (code) { return res.json(countries.filter(c => c.code.toLowerCase().indexOf(code.toLowerCase()) > -1 || c.name.toLowerCase().indexOf(code.toLowerCase()) > -1)); } return res.json(countries); }); return router; }; const countries = [ { name: 'Afghanistan', code: 'AF' }, { name: 'Åland Islands', code: 'AX' }, { name: 'Albania', code: 'AL' }, ...
Now that our server-side code is setup let us now turn our attention to the ReactJS application. We create a component named Country in a file named the same. We use function component here and make use of hooks as well. The component renders an input followed by an unordered list of countries retrieved from the API.
3. Client-Side Code
We make use of useEffect
hook to make a call to the API. Inside the useEffect
callback, we use window.fetch
to make a get call to the URL http://localhost:2019/api/country/:code?
. This API returns a list of countries with their codes as JSON. We then set the state property countries to the returned list. This will lead to the re-rendering of the component with the list of countries as well.
Initially, when the application loads we pass an empty string to the API which causes it to return the entire list of countries. As the user types in the input subsequent AJAX calls pass on the user typed text to the API. Which in turn returns a filtered list of countries. The code for our component now looks like below:
Country.js
import React, { useState, useEffect } from 'react'; function Country(props) { const [countries, setCountries] = useState([]); const [userText, setUserText] = useState(""); useEffect(() => { window.fetch(`http://localhost:2019/api/country/${userText}`).then((response) => response.json() ).then((c) => { setCountries(c); }); }, [userText]); const handleChange = (evt) => { setUserText(evt.target.value); } return <> <input type="text" onChange={handleChange} value={userText} /> {countries.length <li key={c.code}>{c.name}</li> )} </ul> </>; } export default Country;
4. Code in action
Let us now see all of this in action. We need to make additional changes to our package.json file to run both the server-side and client-side code in parallel when we run npm start. There are npm packages available to do this but for our example, I have chosen to use start instead. This will only work on window systems though.
package.json
"start": "start react-scripts start && start node api/index.js", ...
To run the application now we just need to execute the below command:
> npm start
The default browser should open pointing to URL http://localhost:3000. Below is the screengrab of what you should see on the initial load.
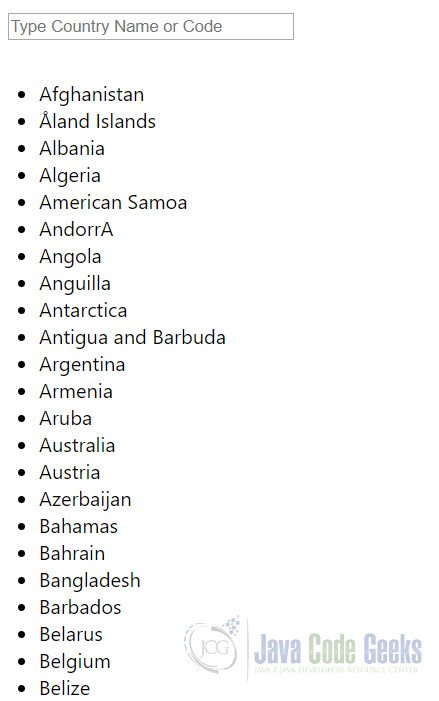
Now as we type in the text box the list is filtered and refined. An example of this in action is below:
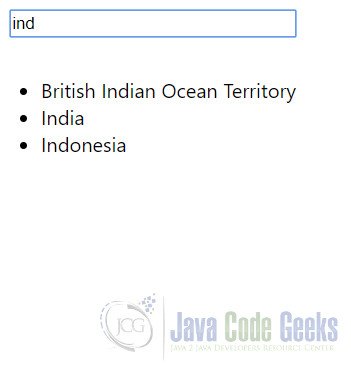
5. Download the Source Code
This was an example of AJAX Request in a ReactJS Application
You can download the full source code of this example here: ReactJS with AJAX Requests Example