ReactJS onChange Example
In this article let us explore event handling in ReactJS and specifically on the onChange event in Reactjs. We take a look at handling the change event of HTML tags.
ReactJS has grown in popularity over the years and has become the go-to library for front end development.
We will create a controlled component that displays an input tag. Subsequently we handle the change event of this tag to run our code. To make things a little interesting let us build a password strength checker. As the user types in a new password we display a string showing the strength of the password.
So without further delay let us get started.
1. Tools and Frameworks
I will use the following tool set and frameworks to build the example in this article.
The create-react-app package quickly spins up a working ReactJS application. We use it here to create a basic application for our demonstration. The latest version of ReactJS is used by the create-react-app package. I have used the Visual Studio Code IDE but you are free to follow along in an IDE of your choice.
2. Setting up Application
We run the below command to execute create-react-app and generate our sample application
>npx create-react-app my-app
npx downloads a temporary copy of create-react-app and runs it with the parameters provided. The above command should create a project in the my-app directory. And the structure of the application should look like below:
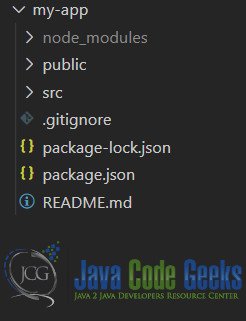
3. Building Password Checker
Let us now create our password strength checker component. To keep things simple we only set three rules for new passwords. With each pass of the rules earning the new password 1 points. For a total of 3 for a strong password, 2 for a medium one and 1 for a weak password. We write this component in a file named StrengthChecker.js. Our code for this component looks like below:
StrengthChecker.js
import React, { useState } from 'react'; function StrengthChecker(props) { const [strength, setStrength] = useState("weak"); const [password, setPassword] = useState(""); const handleChange = ({ target }) => { setPassword(target.value); let score = 0; if (password.length >= 6) { score += 1; } if ((password.match(/[A-Z]/g) || []).length >= 1) { score += 1; } if ((password.match(/[0-9]/g) || []).length >= 1) { score += 1; } switch (score) { case 1: setStrength("weak"); break; case 2: setStrength("medium"); break; case 3: setStrength("strong"); break; } } return <div style={{ display: 'flex', flexDirection: 'column', alignItems: 'center', justifyContent: 'center', height: '100vh', width: '100vw' }}> <label>Please type in a new Password</label> <input type="password" value={password} onChange={handleChange} /> <span>Password Strength</span> <span className={strength}> {strength.toUpperCase()}</span> <ul> <li>Atleast 6 characters in length</li> <li>Atleast one Capital letter</li> <li>Atleast on numeric character</li> </ul> </div> } export default StrengthChecker;
As you can see from the code above we have attached the handleChange event handler to the onChange event of the input tag. In the handler we access the value of the tag and check its strength. In the process setting our password strength checker text and corresponding CSS Class.
4. Running the application
Let us run our application to see our component in action. We run the below command at the root of our project.
/my-app> npm start
This opens the URL http://localhost:3020 in our browser. As we type in to the text box we should see the password strength update simultaneously.
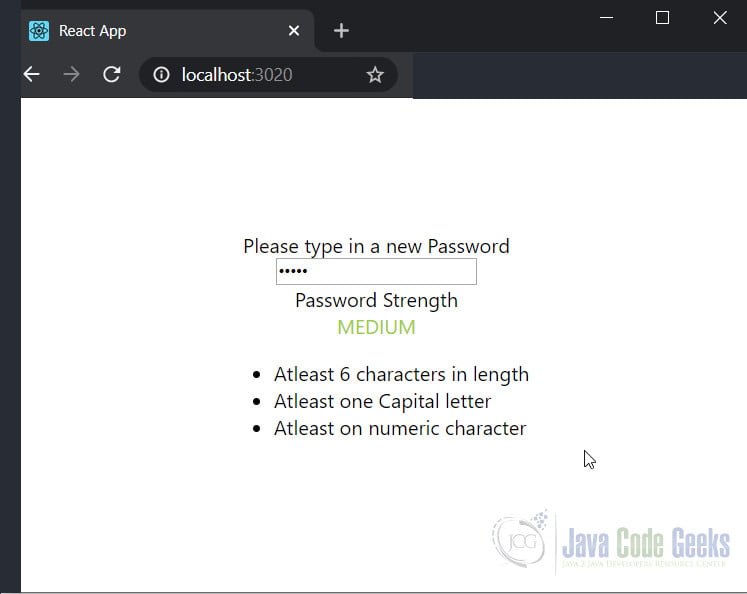
5. Download the Source Code
You can download the full source code of this example here: ReactJS onChange Example