ReactJS Infinite Scroll Example
In this article, we implement the Infinite scroll feature in a ReactJS application. This feature allows us to load and present additional content as the user scrolls the container. It creates an impression of seemingly infinite content and thus the name infinite scroll. In this article, we will create a container that, when scrolled would load additional content into the container. We will wrap this feature up into a React component to make it flexible and reusable across applications.
Although there are many ready components of this nature available, we will implement our own from scratch. So, let’s get started.
1. Tools and Frameworks
We use the below toolset for this example, some of these you can replace with those of your own choice. While others are mandatory to follow along with the example.
This npm package allows us to quickly create a skeletal React app that serves the purpose of this example.
This IDE is my editor of choice while working with React but there are other options out there and you can switch to your own.
2. Initial Setup
To start off we use the create-react-app to create the starting point of this example. Running the below command creates our application and opens it up in Visual Studio Editor for editing.
> npx create-react-app my-app .
Once this command completes we execute the below after switching to the directory where our app resides.
>code .
Now we need to add the following npm packages to setup our example.
- Typescript
We are using Typescript to write our code for this example and thus need to install typescript for the purpose.
- React Typings
React typings are required when using typescript to write a React Application.
- React Polyfill
This allows our application to run in the latest IE browser.
We can install the above dependencies by running the below command at the root of the project.
> npm install typescript @types/react react-app-polyfill --save
Once the above command completes we are good to go and can start implementing our infinite scroll feature.
3. Project Structure
The initial application or folder structure should look like below.
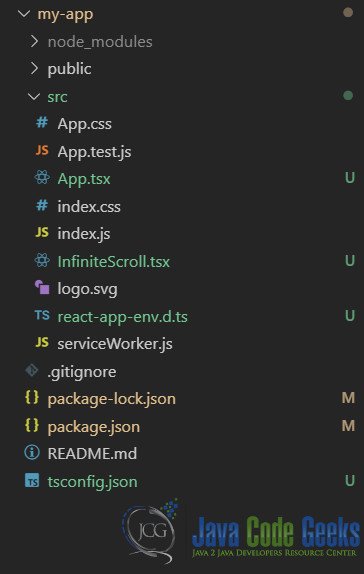
4. Implementation
Now I’ll add a file called InfiniteScroll.tsx which will be a class component and an infinitely scrolling container. In the render method of our component we return a simple div
decorated with the CSS class scroller
. We also handle the onScroll
event of this container. Through the CSS below we accomplish the following.
Disable the horizontal scrollbar and hide the vertical scrollbar across browsers but still keep the div
scrollable.
InfiniteScroll.css
.scroller { width: 50%; height: 240px; background-color: green; overflow: scroll; overflow-x: hidden; /* IE Hide Scrollbar */ -ms-overflow-style: none; } /* Chrome Hide Scrollbar */ .scroller::-webkit-scrollbar { width: 0 !important; } /* Firefox Hide Scrollbar */ .scroller { overflow: -moz-scrollbars-none; scrollbar-width: none; }
We import this file into our component and our component looks like below
InfiniteScroll.tsx
import React from 'react'; import './InfiniteScroll.css'; class InfiniteScroll extends React.Component { scroll: any; scroller: any; constructor(props: any) { super(props); this.state = { counter: 1, position: 0 }; this.scroller = React.createRef(); } render = () => { return <div className='scroller' onScroll={this.onScroll} ref={this.scroller}></div> } componentDidMount = () => { this.addDivs(5); } onScroll = () => { if(this.scroller.current.scrollTop > this.state.position){ this.addDivs(2); } this.setState({ position: this.scroller.current.scrollTop }); } addDivs = (number: any) => { let cnt = +this.state.counter; for (let i = 1; i <= number; i++) { let div = document.createElement("div"); div.innerText = cnt.toString(); div.style.width = "90%"; div.style.height = "200px"; div.style.backgroundColor = "blue"; this.scroller.current.appendChild(div); cnt = +cnt + 1; } this.setState({ counter: cnt }); } } export default InfiniteScroll;
The onScroll
method adds more div
tags to the container every time the container is scrolled down further. This creates an infinitely scrolling container. To run the code and see this in action we need to run the below command
>npm run start
Now we can navigate to http://localhost:3000
to see the results. Which look like below:
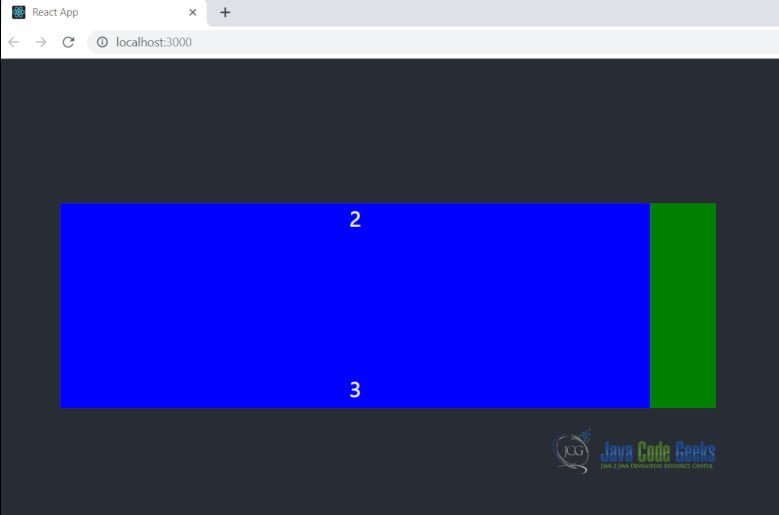
This wraps up our look at creating an Infinite Scroll Example using ReactJS.
5. Download the Source Code
You can download the full source code of this example here: ReactJS Infinite Scroll Example
Nice demo!
Thanks!