ReactJS Event Listener Example
In this article, we take a look at event handling with ReactJS. ReactJS is a very popular front-end library used to build Front ends of high-performance applications. Handling events in ReactJS is very similar to handling DOM events using plain JavaScript. But there are some differences as we will see in this article.
1. Introduction
Let us get started by building a sample application to learn and demonstrate the concepts covered in the article. I have used the following tech stack and tools to build the sample application we will see in this example.
First up we will create a React app using the create-react-app package from npm. The below command should do the job for us:
> npx create-react-app my-react-app .
This will create a React application in the current directory and install all the dependencies as well.
Our newly created project structure should look like the following:
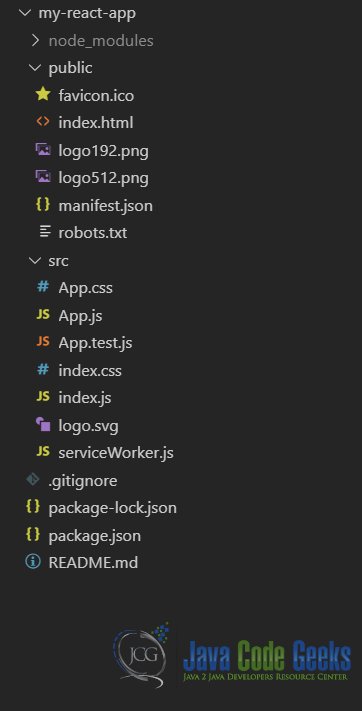
2. ReactJS Event Listener Example
Now let us create a couple of components and try and learn how to handle events while at it. First Component would have a button whose click event will be handled. On the click of the button, we will show an alert popup to greet the user. The code for the component is listed below and the explanation of the same follows:
Greet.tsx
import React from 'react'; class Greet extends React.Component { constructor (props: any) { super(props); this.handleClick = this.handleClick.bind(this); } render = () => { return <div> <button onClick={this.greetUser}>Press Me</button> <button onClick={this.handleClick}>Do Not Press</button> </div> } handleClick() { alert('Warning! Do Not Press!'); } greetUser = () => { alert('Hello there!'); } } export default Greet;
Here this component renders two buttons and handles their click event. Things to note about the syntax are the camel casing of names of events in React. Also, if you are not using arrow functions you need to bind the methods to this
in the constructor like in the code above. Apart from these one more thing of note is that we pass a function instead of a string to the event. As in onClick={this.handleClick}
instead of onClick="handleClick()"
.
Now when we run the project using npm start
and navigate to http://localhost:3000
in the browser, we see the following results.
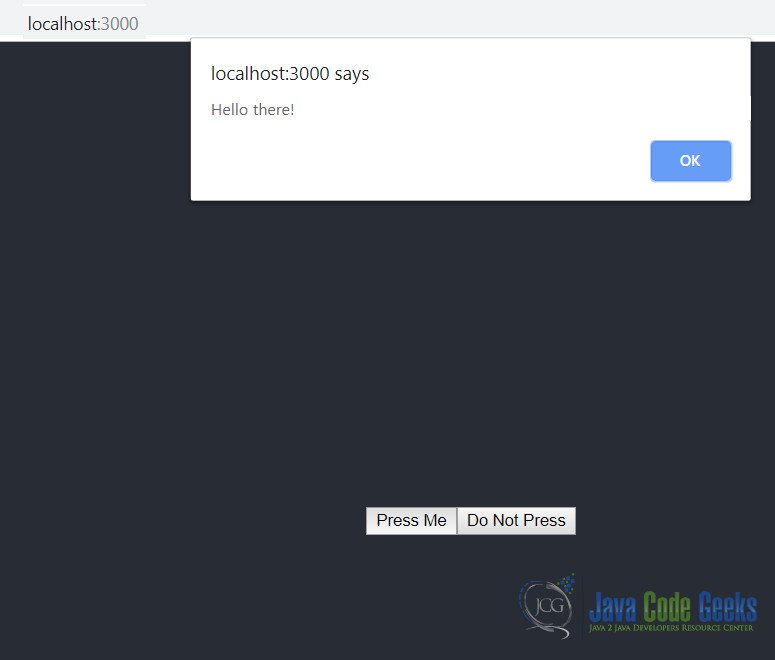
3. Binding Event Listeners
One more nuance of event handling in React is that the default action cannot be prevented by returning a false. We need to explicitly call the preventDefault
method on the event
object passed in. The event object passed into the event handler is created by React itself to iron over the differences in implementations across browsers. Let us take a look at how that works in another example where we handle the click event of an anchor tag.
Our code for this example looks like below and the explanation follows:
Link.tsx
import React from 'react'; class Link extends React.Component { render = () => { return <div> <a href="http://www.javacodegeeks.com" onClick={this.handleClick}>Take me to Java Code Geeks!</a> </div> } handleClick = (e: any) => { e.preventDefault(); alert('Wait...'); } } export default Link;
Here as you can see we call the preventDefault method on the event object passed into the event handler. This prevents the default action of navigating to JavaCodeGeeks.com website and instead shows an alert message to the user like below:
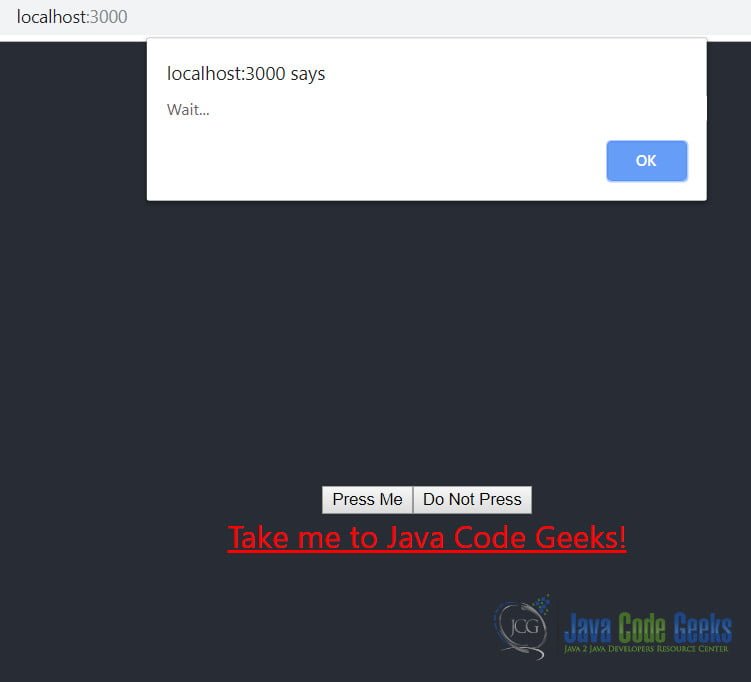
This wraps up our look at event handling with ReactJS.
4. Download the Source Code
You can download the full source code of this example here: ReactJS Event Listener Example