Microservices and Azure: Boosting Business Potential
Switching from a big, all-in-one system to a microservices setup brings significant advantages for businesses. Microservices is a way of organizing software where applications are created as a collection of small, independent units. In the constantly changing world of technology, the Azure platform stands out as a helpful tool for businesses looking for a strong, scalable, and efficient way to develop applications. Our move from the old way of doing things to microservices on Azure isn’t just a tech change – it’s a smart move to get the most out of what our business can do.
Azure offers a bunch of services, tools, and tech support to make it easier to create microservices applications. It’s not just about writing code; it’s about building a digital setup that fits perfectly with what our business is trying to achieve.
As we start this journey, think of it as more than just a tech switch – it’s a strategic boost for your business. In this article, we’re going to look at all the great opportunities Azure gives us for making microservices – a way of doing things that promises to be flexible, scalable, and a way to discover new ways of doing things.
1. What are the Benefits of Microservices Architecture
Microservices architecture offers a multitude of advantages for businesses, fostering agility, scalability, and enhanced operational efficiency. Some key benefits include:
Benefit | Description |
---|---|
Modularity and Flexibility | Enables the development of loosely coupled, independent services, enhancing flexibility and ease of modification. |
Scalability and Resource Optimization | Allows independent scaling of specific services based on demand, optimizing resource allocation. |
Rapid Development and Deployment | Promotes faster development cycles and quicker deployment by allowing concurrent work on individual services. |
Fault Isolation and Resilience | Isolates faults to specific services, ensuring the resilience of the overall system in the face of failures. |
Technology Diversity | Permits the use of different programming languages and technologies for individual microservices. |
Improved Scaling and Load Balancing | Facilitates efficient scaling by enabling independent scaling of relevant microservices and optimizing load balancing. |
Enhanced Fault Isolation | Minimizes the impact of failures in one service on the entire system, making it easier to identify and address issues. |
Streamlined Maintenance and Upgrades | Simplifies version control and allows updates and maintenance without disrupting the entire application. |
Easier Adoption of DevOps Practices | Aligns well with DevOps principles, promoting collaboration and agility in the development process. |
Adaptability to Business Changes | Provides flexibility to adapt to changing market conditions, customer needs, and emerging technologies. |
This table provides a concise overview of the diverse advantages offered by microservices architecture.
2. A Comprehensive Guide to Building Microservices in Azure
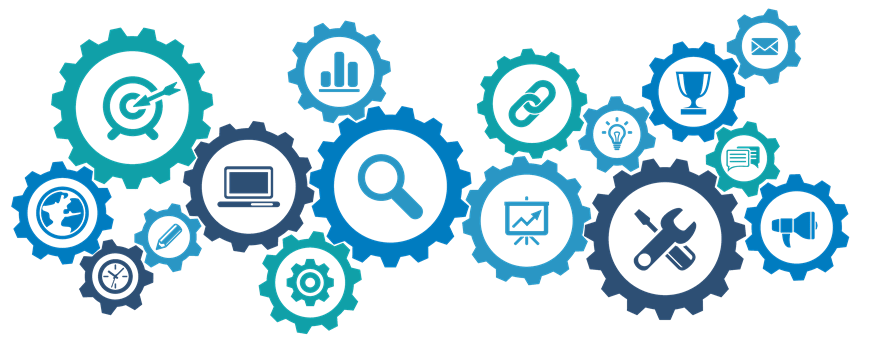
Building microservices in Azure is more than a technological transition; it’s a strategic decision to embrace scalability, flexibility, and efficiency in your application architecture. In this guide, we’ll walk you through a step-by-step process, enriched with real-life examples and code snippets, to empower you in crafting a robust microservices ecosystem on the Azure platform.
Step 1: Define Microservices Boundaries
Begin by clearly defining the boundaries of your microservices. Identify individual functionalities that can operate independently. For instance, in an e-commerce application, you might have separate microservices for user authentication, product catalog, and order processing.
Example Code Snippet (pseudo-code):
// User Authentication Microservice @RestController public class AuthController { // API endpoints for user authentication } // Product Catalog Microservice @RestController public class ProductController { // API endpoints for managing products } // Order Processing Microservice @RestController public class OrderController { // API endpoints for processing orders }
Step 2: Choose Azure Services for Microservices
Select Azure services that align with the specific requirements of each microservice. For instance, use Azure Kubernetes Service (AKS) for container orchestration, Azure Functions for serverless compute, and Azure Cosmos DB for a globally distributed, multi-model database.
Example Code Snippet (Azure Functions):
// Azure Function for processing orders public static class OrderProcessingFunction { [FunctionName("ProcessOrder")] public static void Run( [QueueTrigger("orderqueue", Connection = "AzureWebJobsStorage")] string order, ILogger log) { // Logic for processing the order } }
Step 3: Implement Communication Between Microservices
Establish communication mechanisms between microservices. Azure Service Bus or Azure Event Grid can be employed for asynchronous communication, while API Gateway or Azure API Management can handle synchronous communication.
Example Code Snippet (Azure Service Bus – Sender):
// Sender Microservice QueueClient queueClient = new QueueClient(connectionString, queueName); await queueClient.SendAsync(new Message(Encoding.UTF8.GetBytes("New order placed")));
Example Code Snippet (Azure Service Bus – Receiver):
// Receiver Microservice QueueClient queueClient = new QueueClient(connectionString, queueName); queueClient.RegisterMessageHandler(ProcessMessagesAsync, exceptionReceivedHandler);
Step 4: Ensure Data Consistency Across Microservices
Maintain data consistency by employing distributed transactions or eventual consistency patterns. Azure provides services like Azure SQL Database or Cosmos DB, which can be configured for multi-region, multi-model data storage.
Example Code Snippet (Azure Cosmos DB – Java SDK):
// Cosmos DB Client Initialization CosmosClient cosmosClient = new CosmosClientBuilder() .endpoint(cosmosEndpoint) .key(cosmosKey) .buildClient(); // CRUD Operations CosmosContainer container = cosmosClient.getDatabase(databaseName).getContainer(containerName); ItemResponse<MyObject> response = container.createItem(myObject);
Step 5: Implement Monitoring and Logging
Ensure robust monitoring and logging across microservices. Leverage Azure Monitor, Azure Application Insights, or ELK (Elasticsearch, Logstash, and Kibana) stack for comprehensive insights into application performance and behavior.
Example Code Snippet (Azure Application Insights in ASP.NET Core):
// Startup.cs public void ConfigureServices(IServiceCollection services) { // Add Application Insights telemetry services.AddApplicationInsightsTelemetry(Configuration["ApplicationInsights:InstrumentationKey"]); // Other configurations... }
Each decision, backed by real-life examples and code snippets, is aimed at empowering you to build not just an application but a dynamic ecosystem that adapts and scales with the evolving needs of your business.
3. Microservices Integration with DevOps for Seamless Development and Operations
Microservices architecture and DevOps practices, when seamlessly integrated, form a powerful alliance that transforms the development and operations landscape. In this exploration, we’ll delve into how microservices and DevOps work in tandem, enhancing agility, collaboration, and the overall efficiency of the software development lifecycle.
Let’s present the information in five tables, each focusing on a specific aspect of how microservices work with DevOps.
Table 1: Continuous Integration and Continuous Deployment (CI/CD)
Microservices Integration | DevOps Harmony | Example |
---|---|---|
Microservices are conducive to CI/CD pipelines. | DevOps practices emphasize the automation of build, test, and deployment processes. | In an e-commerce application, the payment microservice undergoes updates independently, triggering automated tests and deployments without affecting other services. |
Table 2: Automated Testing
Microservices Integration | DevOps Harmony | Example |
---|---|---|
Microservices promote independent testing of each service. | DevOps advocates for automated testing at every stage of the development pipeline. | A healthcare application’s patient registration microservice undergoes automated unit tests and integration tests to ensure seamless interactions with other services. |
Table 3: Infrastructure as Code (IaC)
Microservices Integration | DevOps Harmony | Example |
---|---|---|
Microservices are inherently decoupled, allowing for flexible deployment. | DevOps practices leverage Infrastructure as Code (IaC) to automate the provisioning and management of infrastructure. | A logistics application’s tracking microservice defines its infrastructure requirements in code, enabling consistent deployment across environments. |
Table 4: Monitoring and Logging
Microservices Integration | DevOps Harmony | Example |
---|---|---|
Microservices generate diverse data streams, requiring comprehensive monitoring and logging. | DevOps emphasizes real-time monitoring and logging for prompt issue detection. | An online gaming platform’s matchmaking microservice logs performance metrics, with DevOps tools aggregating and analyzing data to address performance bottlenecks proactively. |
Table 5: Collaboration and Communication
Microservices Integration | DevOps Harmony | Example |
---|---|---|
Microservices development requires close collaboration among cross-functional teams. | DevOps fosters a culture of collaboration between development, operations, and stakeholders. | A content delivery platform’s content indexing microservice team collaborates seamlessly with operations to ensure efficient scaling during peak usage periods. |
These tables show how microservices and DevOps work together at different stages of making software.
4. Conclusion
In wrapping up our exploration of the symbiotic relationship between microservices and DevOps, we’ve unraveled a narrative of efficiency, agility, and collaboration. The essence of this journey lies in the seamless integration of microservices architecture with the principles of DevOps—a dynamic partnership that propels software development and operations to new heights.
As you navigate the intricacies of building, testing, and deploying applications, envision a future where every development cycle is a stride toward innovation, fueled by modular microservices and orchestrated by the collaborative spirit of DevOps. Embrace this harmonious dance between agility and reliability, for it’s not just about code; it’s about sculpting a resilient and responsive digital ecosystem.