A Beginner’s Guide to Architectural Patterns
Unlock the mysteries of software development with a deep dive into Architectural Patterns. This guide is your gateway to understanding various software structures, exploring their traits, applications, and impact on design. Just like time-tested recipes in modern software engineering, these Architectural Patterns address specific challenges. Whether you’re a burgeoning architect or a curious developer, this resource simplifies complexities, aiding you in choosing the right approach for your unique needs.
Below we’ll delve into eight commonly used architectural patterns, providing insights into their application and significance in software development.
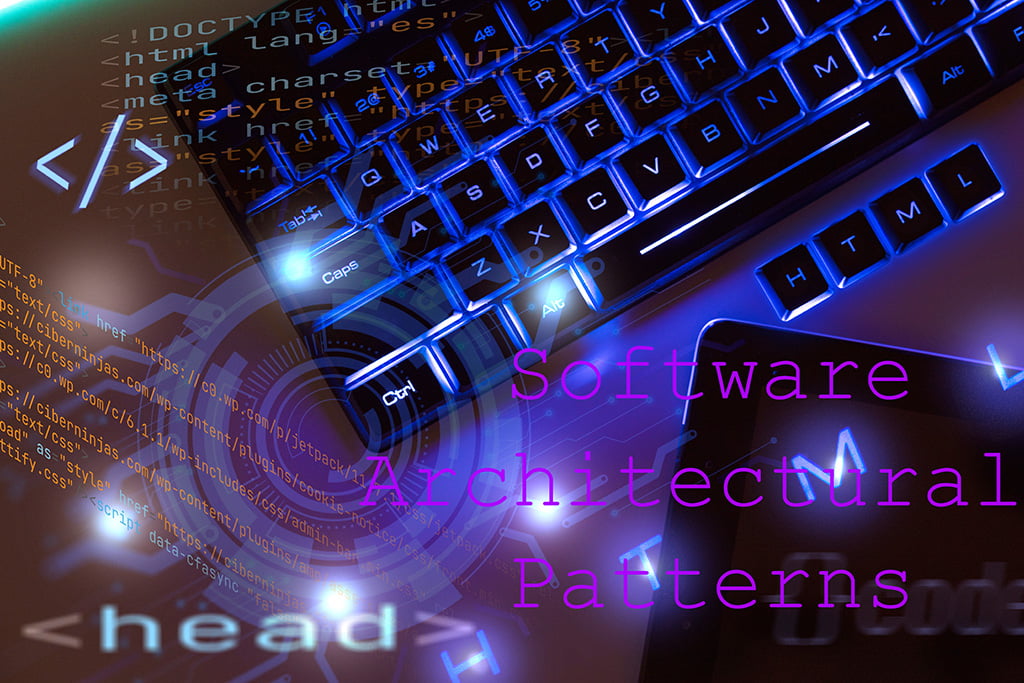
1. Monolithic Architecture
Monolithic architecture is a traditional approach where all components of an application are tightly integrated into a single codebase, sharing the same data and logic. It’s a cohesive unit where the entire application is deployed as one entity.
Pros:
- Simplicity: Easier to develop and understand as everything is in one place.
- Centralized Control: Changes are coordinated centrally, making it easier to manage.
Cons:
- Scalability Issues: Scaling one part of the application means scaling the entire monolith.
- Maintenance Challenges: As the application grows, it becomes harder to maintain and update.
Example: Consider a simple e-commerce application where all functionalities, including user authentication, order processing, and inventory management, are tightly coupled.
// User.java @Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String username; // other user-related fields... // getters and setters... } // Product.java @Entity public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private BigDecimal price; // other product-related fields... // getters and setters... } // Order.java @Entity public class Order { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @ManyToOne @JoinColumn(name = "user_id", nullable = false) private User user; @ManyToOne @JoinColumn(name = "product_id", nullable = false) private Product product; private int quantity; // other order-related fields... // getters and setters... } // OrderController.java @RestController @RequestMapping("/orders") public class OrderController { @Autowired private OrderRepository orderRepository; @Autowired private UserRepository userRepository; @Autowired private ProductRepository productRepository; @PostMapping("/place") public ResponseEntity<String> placeOrder(@RequestBody OrderRequest orderRequest) { // Logic to process an order, interact with User, Product, and Order entities... // Use userRepository, productRepository, and orderRepository... return ResponseEntity.ok("Order Placed Successfully!"); } } // OrderRequest.java public class OrderRequest { private Long userId; private Long productId; private int quantity; // other order-related fields... // getters and setters... }
In this Java/Spring Boot example, we’ve created entities for User
, Product
, and Order
, similar to the Python/Django example. The OrderController
handles the HTTP request for placing an order, and the OrderRequest
represents the request payload. This Java example demonstrates the monolithic structure, where all components are tightly coupled within a single codebase.
2. Microservices Architecture
Microservices architecture is an approach where a large application is divided into smaller, independent services that communicate with each other through APIs. Each service is developed and deployed independently, promoting flexibility and scalability.
Pros:
- Improved Scalability: Microservices allow for scaling specific services independently based on demand, optimizing resource usage.
- Independent Deployment: Services can be developed, deployed, and updated independently, enabling continuous delivery and reducing downtime.
- Technology Diversity: Different services can be built using various technologies, allowing for flexibility and innovation within the system.
Cons:
- Increased Complexity: Managing multiple services introduces complexity in terms of service discovery, communication, and distributed data management.
- Potential Communication Overhead: Inter-service communication may introduce latency and overhead, affecting system performance.
- Challenging Debugging: Debugging becomes challenging as issues may span multiple services, making it harder to trace and identify root causes.
Example Code (Java/Spring Boot):
UserService.java
@RestController @RequestMapping("/users") public class UserService { @Autowired private UserRepository userRepository; @GetMapping("/{userId}") public ResponseEntity<User> getUser(@PathVariable Long userId) { User user = userRepository.findById(userId) .orElseThrow(() -> new ResourceNotFoundException("User not found with id: " + userId)); return ResponseEntity.ok(user); } @PostMapping public ResponseEntity<User> createUser(@RequestBody User user) { // Logic to create a new user... userRepository.save(user); return ResponseEntity.status(HttpStatus.CREATED).body(user); } }
ProductService.java
@RestController @RequestMapping("/products") public class ProductService { @Autowired private ProductRepository productRepository; @GetMapping("/{productId}") public ResponseEntity<Product> getProduct(@PathVariable Long productId) { Product product = productRepository.findById(productId) .orElseThrow(() -> new ResourceNotFoundException("Product not found with id: " + productId)); return ResponseEntity.ok(product); } @PostMapping public ResponseEntity<Product> createProduct(@RequestBody Product product) { // Logic to create a new product... productRepository.save(product); return ResponseEntity.status(HttpStatus.CREATED).body(product); } }
In this Java/Spring Boot example, we have two microservices: UserService
and ProductService
, each handling user and product-related operations independently. This demonstrates the decentralized nature of microservices architecture, where each service is responsible for its specific functionality.
3. Layered Architecture
Layered architecture is a design pattern where an application is organized into layers, with each layer having a specific responsibility. It typically consists of presentation, business logic, and data access layers, promoting a modular and structured approach to software design.
Pros:
- Modular Design: Layers provide a clear separation of concerns, making the system more modular and easier to understand.
- Easy Maintenance: Changes in one layer typically do not affect others, simplifying maintenance and updates.
- Scalability: Scalability is achievable by scaling specific layers independently.
Cons:
- Tight Coupling: Layers may become tightly coupled, leading to challenges if changes in one layer impact others.
- Limited Flexibility: Adding new functionalities may require modifications across multiple layers, limiting flexibility.
- Potential for Duplication: Logic may be duplicated across layers, leading to redundancy and increased complexity.
Example Code (Java/Spring Boot):
UserController.java (Presentation Layer)
@RestController @RequestMapping("/users") public class UserController { @Autowired private UserService userService; @GetMapping("/{userId}") public ResponseEntity<User> getUser(@PathVariable Long userId) { return ResponseEntity.ok(userService.getUser(userId)); } @PostMapping public ResponseEntity<User> createUser(@RequestBody User user) { return ResponseEntity.status(HttpStatus.CREATED).body(userService.createUser(user)); } }
UserService.java (Business Logic Layer)
@Service public class UserService { @Autowired private UserRepository userRepository; public User getUser(Long userId) { return userRepository.findById(userId) .orElseThrow(() -> new ResourceNotFoundException("User not found with id: " + userId)); } public User createUser(User user) { // Business logic to create a new user... return userRepository.save(user); } }
UserRepository.java (Data Access Layer)
@Repository public interface UserRepository extends JpaRepository<User, Long> { // Data access methods for User entity... }
In this Java/Spring Boot example, the layered architecture is demonstrated with three layers: UserController
(Presentation Layer), UserService
(Business Logic Layer), and UserRepository
(Data Access Layer). Each layer has a specific responsibility, promoting a structured and modular design.
4. Event-Driven Architecture
Event-Driven Architecture (EDA) is a design pattern where components communicate with each other by producing or consuming events. Events, which represent significant occurrences, trigger actions or processes in a decoupled manner, allowing for increased flexibility and responsiveness.
Pros:
- Decoupled Components: Components are loosely coupled, allowing for independent development and easier maintenance.
- Real-Time Responsiveness: Events trigger immediate actions, enabling real-time responsiveness to changes or updates.
- Scalability: The architecture is inherently scalable, as components can be added or modified independently.
Cons:
- Challenging Debugging: Debugging and tracing events across components can be challenging, requiring robust monitoring and logging.
- Potential Event Cascades: A chain of events may be triggered, leading to complexities in understanding the flow of actions.
- Learning Curve: Developers need to adapt to an asynchronous, event-driven mindset, which may have a learning curve.
Example Code (Java/Spring Boot):
EventProducer.java
@Component public class EventProducer { @Autowired private ApplicationEventPublisher eventPublisher; public void produceEvent(String message) { EventData event = new EventData(message); eventPublisher.publishEvent(new CustomEvent(this, event)); } }
CustomEvent.java
public class CustomEvent extends ApplicationEvent { private final EventData eventData; public CustomEvent(Object source, EventData eventData) { super(source); this.eventData = eventData; } public EventData getEventData() { return eventData; } }
EventConsumer.java
@Component public class EventConsumer implements ApplicationListener<CustomEvent> { @Override public void onApplicationEvent(CustomEvent event) { EventData eventData = event.getEventData(); // Logic to process the event data... System.out.println("Event Received: " + eventData.getMessage()); } }
In this Java/Spring Boot example, we have an Event-Driven Architecture with three components: EventProducer
, CustomEvent
, and EventConsumer
. The EventProducer
produces an event, the CustomEvent
represents the event, and the EventConsumer
processes the event asynchronously. This showcases the decoupled nature of event-driven systems, where components communicate through events.
5. Service-Oriented Architecture (SOA)
Service-Oriented Architecture (SOA) is an architectural pattern where an application is composed of loosely coupled and independently deployable services. These services expose functionalities through well-defined interfaces, promoting reusability and flexibility in software design.
Pros:
- Reusability of Services: Services can be reused across different applications, enhancing efficiency and reducing development efforts.
- Easy Maintenance: Independent services are easier to maintain as changes in one service do not affect others.
- Easier Integration: Services can be integrated into various applications, fostering interoperability across different platforms.
Cons:
- Complex Integration: Integrating services may require additional effort due to the need for standardized interfaces and communication protocols.
- Potential for Performance Bottlenecks: Centralized services may become a bottleneck if not designed for high performance.
- Dependency on Network: Service communication over a network introduces the dependency on network reliability and latency.
Example Code (Java/Spring Boot):
UserService.java
@RestController @RequestMapping("/users") public class UserService { @Autowired private UserRepository userRepository; @GetMapping("/{userId}") public ResponseEntity<User> getUser(@PathVariable Long userId) { return ResponseEntity.ok(userRepository.findById(userId) .orElseThrow(() -> new ResourceNotFoundException("User not found with id: " + userId))); } @PostMapping public ResponseEntity<User> createUser(@RequestBody User user) { // Business logic to create a new user... return ResponseEntity.status(HttpStatus.CREATED).body(userRepository.save(user)); } }
In this Java/Spring Boot example, the UserService
represents a service in a Service-Oriented Architecture. It exposes functionalities related to user data through well-defined endpoints. Other services could exist independently, each focusing on specific functionalities. This demonstrates the modular and loosely coupled nature of services in a Service-Oriented Architecture.
6. Model-View-Controller (MVC)
Model-View-Controller (MVC) is a software architectural pattern that separates an application into three interconnected components: the model (data and business logic), the view (user interface), and the controller (handles user input and updates the model). This separation of concerns promotes modularity and maintainability.
Pros:
- Separation of Concerns: Divides the application into distinct responsibilities, making it easier to manage and maintain.
- Modular Design: Each component (model, view, and controller) can be developed and modified independently, promoting code reuse.
- Easy to Understand: The clear separation of responsibilities makes it easier for developers to understand and work on different parts of the application.
Cons:
- Potential for Overuse of Controllers: In some implementations, controllers may become bloated, leading to maintenance challenges.
- Increased Complexity: In larger applications, the number of components and interactions can lead to increased complexity.
- Learning Curve: Developers new to MVC may initially find it challenging to grasp the concept of separation of concerns.
Example Code (Java/Spring Boot):
UserController.java (Controller)
@Controller @RequestMapping("/users") public class UserController { @Autowired private UserService userService; @GetMapping("/{userId}") public String getUser(Model model, @PathVariable Long userId) { User user = userService.getUser(userId); model.addAttribute("user", user); return "user-details"; // View name } @PostMapping public String createUser(@ModelAttribute User user) { userService.createUser(user); return "redirect:/users"; } }
User.java (Model)
@Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String username; // Other user-related fields... // Getters and setters... }
user-details.html (View)
<html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>User Details</title> </head> <body> <h1>User Details</h1> <p th:text="${user.username}"></p> <!-- Other user details... --> </body> </html>
In this Java/Spring Boot example, the UserController
serves as the controller, User
as the model, and user-details.html
as the view. This demonstrates the MVC pattern, where the controller handles user input, the model manages data and business logic, and the view presents the user interface.
7. Serverless Architecture
Serverless architecture is a cloud computing model where cloud providers manage the infrastructure, automatically scaling resources based on demand. Applications are divided into small, independent functions that are executed in response to events or HTTP requests. Serverless eliminates the need for manual infrastructure management.
Pros:
- Cost-Effective: Pay only for actual usage, as there are no fixed infrastructure costs.
- Automatic Scaling: Automatically scales based on demand, handling varying workloads efficiently.
- Focus on Code: Developers can focus on writing code without managing servers or infrastructure.
Cons:
- Limited Execution Time: Functions typically have a maximum execution time, limiting long-running processes.
- Potential Latency: Cold starts may introduce latency as functions need to be initialized.
- Dependency on Cloud Provider: Tightly coupled with the chosen cloud provider’s serverless platform.
Example Code (JavaScript/AWS Lambda):
lambda-function.js
<!DOCTYPE html> // AWS Lambda function example exports.handler = async (event) => { // Logic to process the event const message = event.message || 'Hello, Serverless World!'; return { statusCode: 200, body: JSON.stringify({ message }), }; };
In this JavaScript example, exports.handler
defines an AWS Lambda function. The function processes an event, and the response includes a status code and a message. This is a simple illustration of a serverless function that can be triggered by various events, such as HTTP requests or changes in a storage bucket. Developers write code, and the cloud provider takes care of infrastructure provisioning and scaling.
8. Repository Pattern
The Repository Pattern is a design pattern that abstracts the data access logic from the rest of the application. It provides a centralized interface to interact with data storage, allowing the application to use a consistent API for accessing and managing data. This pattern promotes separation of concerns by isolating database operations.
Pros:
- Abstraction of Data Access: Provides a clean and consistent API for data access, abstracting away the underlying storage details.
- Centralized Logic: Centralizes data access logic, making it easier to manage and maintain.
- Unit Testing: Facilitates unit testing by allowing the substitution of actual data storage with mock repositories.
Cons:
- Potential Abstraction Overhead: In simpler applications, introducing a repository may add unnecessary complexity.
- Learning Curve: Developers new to the pattern may face a learning curve in understanding the additional layer of abstraction.
- Customization Challenges: Repositories may not perfectly fit every scenario, requiring customization and additional interfaces.
Example Code (Java/Spring Boot):
UserRepository.java
@Repository public interface UserRepository extends JpaRepository<User, Long> { // Custom query methods if needed Optional<User> findByUsername(String username); }
UserService.java
@Service public class UserService { @Autowired private UserRepository userRepository; public User getUserById(Long userId) { return userRepository.findById(userId) .orElseThrow(() -> new ResourceNotFoundException("User not found with id: " + userId)); } public User getUserByUsername(String username) { return userRepository.findByUsername(username) .orElseThrow(() -> new ResourceNotFoundException("User not found with username: " + username)); } public List<User> getAllUsers() { return userRepository.findAll(); } public void saveUser(User user) { userRepository.save(user); } public void deleteUser(Long userId) { userRepository.deleteById(userId); } }
In this Java/Spring Boot example, the UserRepository
interfaces with the database, and the UserService
uses this repository to perform various data access operations. The Repository Pattern abstracts away the details of how data is retrieved or stored, providing a clear and consistent API for the application to interact with the underlying database.
Conclusion
In conclusion, the discussed architectural patterns offer diverse approaches to design and organize software. Whether it’s the simplicity of the Monolithic Architecture, the flexibility of Microservices, or the clean separation in MVC, each pattern serves specific needs. The key is to choose the right pattern based on the project’s requirements and scalability. Remember, there’s no one-size-fits-all solution; it’s about finding the best fit for your application’s goals and future growth