React vs Angular: The Complete Comparison
In this post, we feature a comprehensive article on React vs Angular. We will compare two of the most popular JavaScript Frameworks. Both have their own strengths and weaknesses as compared to each other. We will take a look at those in detail in this piece. Hopefully this attempt will help make a choice for your upcoming projects. Lets get started without further delay.
We will compare React and Angular from the below perspectives:
Table Of Contents
1. Genesis
Jordan Walke, Software Engineer at Facebook, developed React. He was influenced by the XHP Framework for PHP. Consequently React was released as open source library in May of 2013 and has since matured as a popular UI development library. Though there were initial hiccups related to licensing issues. But once React 16.0.0 was released, in September of 2017, under standard MIT license all such concerns were laid to rest. The popularity and fame of React has grown by leaps and bounds even though it does not attempt to provide a one stop solution for application development.
Angular team, at Google, developed Angular. Angular is a complete rewrite of the AngularJS framework with sweeping changes. The first release of Angular or Angular 2 as initially referred to by the team was in September of 2016. Initially the naming of the new framework caused considerable confusion. But once the team decided to use AngularJS for versions 1.x and JS was dropped for versions 2.x confusion cleared up quickly.
2. React vs Angular – Architecture
React promotes a Component based architecture. With a typical React app being a tree or hierarchy of React components. React’s approach to components is different in that it does not split the component further into template and code files. Rather it encapsulates the UI and the code to manage it packaged neatly into a single component. The simplest of React component could just be a simple JavaScript function like below:
function SayHello() { return <h1>Hello JavaCodeGeeks!</h1>; }
Angular is also component based framework. Any Angular app is a hierarchy of components as well. Angular’s take on component is elaborate with separate template files and component style sheets and a file with code to provide behavior to a component. Although it is completely possible to provide the template and styles inline without spilling a component over into multiple files. Things get pretty involved in Real World applications. And packing everything into a single file might make the file look too busy. A typical Angular component declaration looks like below:
import { Component } from '@angular/core'; @Component({ selector: 'app-hello', template: '<h1>Hello JavaCodeGeeks</h1>', styleUrls: ['./hello.styles.css'] }) export class HelloComponent { }
3. Learning Curve
The learning curve with React is pretty flat. It does not take long for someone familiar with JavaScript, CSS and HTML to be productive with React. We are talking a couple of days to a week to get comfortable with the React way of development. The React site provides a complete guide. It is a a few hours read. The hands on tutorial allows getting feet wet while developing a tic tac toe game. Being productive is relatively quick with knowledge of React concepts using a path of your choice.
In comparison the Angular learning curve is steeper as there is a lot to get comfortable with. There site at Angular.io does a good job with their Tour of Heroes example. It still takes a bit longer to be up and running with Angular than React in my opinion. Given the holistic approach of Angular to application development this is expected too.
4. Mobile Platform Support
Facebook came out with React Native which enables native development for Android, iOS and UWP using React in March of 2015. Jordan Walke found a way to generate native UI from a background JavaScript thread. This concept was refined and developed into what we call React Native today. Facebook invested in this as they leaned towards providing a native app like experience as opposed to one based on HTML.
Angular powers the Ionic framework which allows creation of mobile experiences. It uses web technologies like JavaScript, CSS and HTML to allow development of hybrid applications. There is even a React Native Render project which allows creating a React Native Project in which an Angular application runs. Overall both React and Angular have extensive support for the Mobile Platform.
5. Tooling
Tool sets built around frameworks and libraries are considered just as important as the technology themselves. They on board enthusiasts to become full time patrons of the technology and contribute to it’s success and popularity.
React has a rich ecosystem of tools and libraries from third parties to aid development. Facebook’s create-react-app project generates a skeleton for a full blown React application. We can use it as follows, it places the generated application in a folder named react-app :
>npx create-react-app react-app
The generated React app structure looks like below:
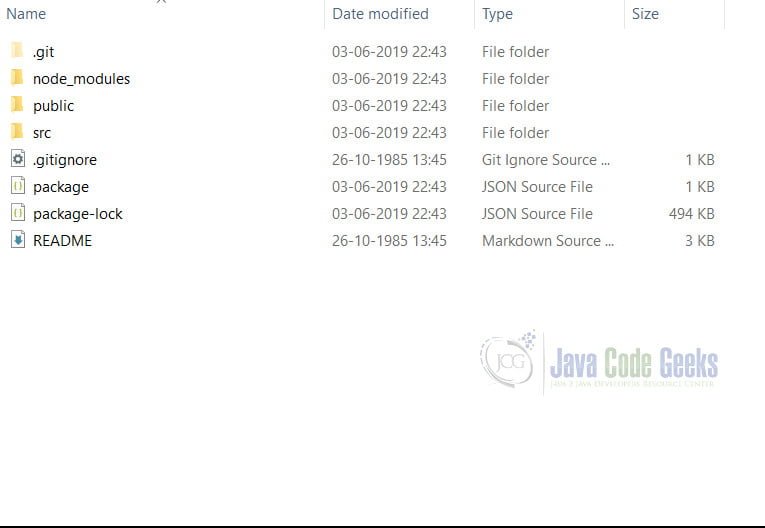
Apart from this libraries like Axios are available for example to make AJAX calls. Also, there are several IDEs, plugins for popular IDEs to aid React development. ReactIDE is a dedicated IDE for React development for example.
Angular has the angular cli which aids in development and generates application which works right out of the box. Visual Studio Code IDE is a great tool for Angular development. The ecosystem around angular edges out React with tons of third party component libraries to aid and speed up development of libraries. We can use the Angular CLI like below to generate an Angular Application:
>ng new my-angular-app
The above command scaffolds a new angular application and places it under the folder my-angular-app. The folder structure is as below:
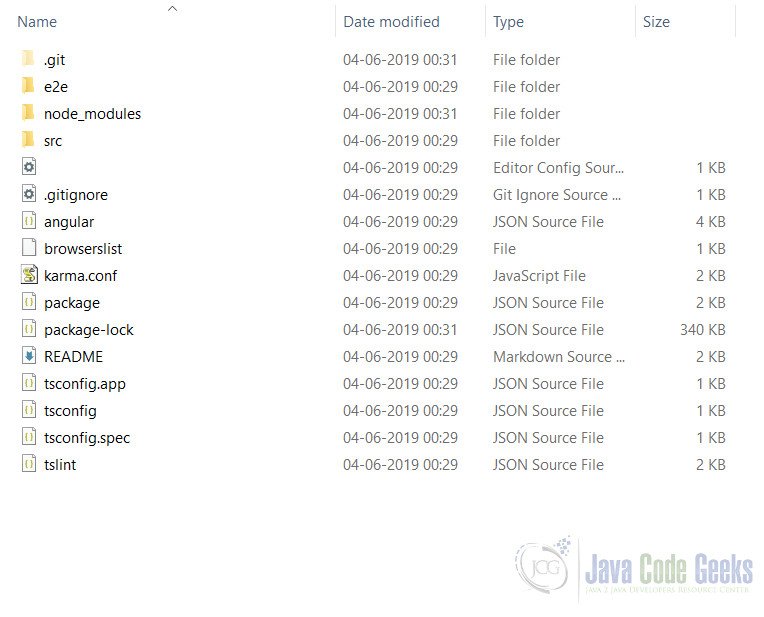
6. Template System
React uses JSX which allows to inject HTML into our JavaScript code. This term was coined by the React team. The React template engine uses the concept of a virtual DOM. The engine makes only necessary changes to the DOM as opposed to completely re rendering the entire component. This makes the updates to the DOM efficient and improves performance. On the other hand this requires React to maintain a virtual DOM in memory increasing RAM memory requirements.
Angular templates use HTML. To support extended behavior Angular supports creating custom tags and directives. Its interpolation syntax is quite powerful as well. It allows template expressions which enable dynamic behaviors.
7. Server side Capabilities
React does have server side rendering capabilities. We can render our components on the server and return plain HTML to the browser. Although server side rendering can increase response times and slow down the application. In certain use cases, like SEO, this is a necessity.
Angular Universal technology allows rendering angular applications on the server as well. This improves response times for low powered devices like mobile phones on 3G connections. This is based on the Nodejs Express Server. Angular site hosts an example to create a server side rendered version of the Tour of Heroes application.
8. Dependency Injection
React does not have dependency injection. Although there are examples out there to implement it using Higher Order Components. These are components which take as input components and generate new components. With that said React does not have native dependency injection.
Dependency Injection is one of the corner stone features of Angular framework. Everywhere you look in the framework it is at work. Angular has its own DI framework, it leads to more modular code. Also, it lends itself to testing by injecting mock instances to test components.
9. Data Binding
React supports Uni directional or one way data binding. Any changes in the state leads to re rendering of the UI elements but any change in the UI does not automatically update the model. We need to figure out on our own how to update the backing state with changes. One way is to use callbacks to achieve it. The technique is referred to as Controlled Components and looks like below:
class SayHello extends React.Component { constructor(props) { super(props); this.state = { name: "JavaCodeGeeks" }; } updateName(e){ this.setState({ name: $event.target.value }); } render() { return (<div> <input value={this.state.name} onChange="this.updateName" /> <h1>Hello {this.state.name}</h1> </div>); } }
The above code shows an input
tag on screen and as we type into it updates the Heading text. The initial text is Hello JavaCodeGeeks but as I start to type in my name the result is like below:
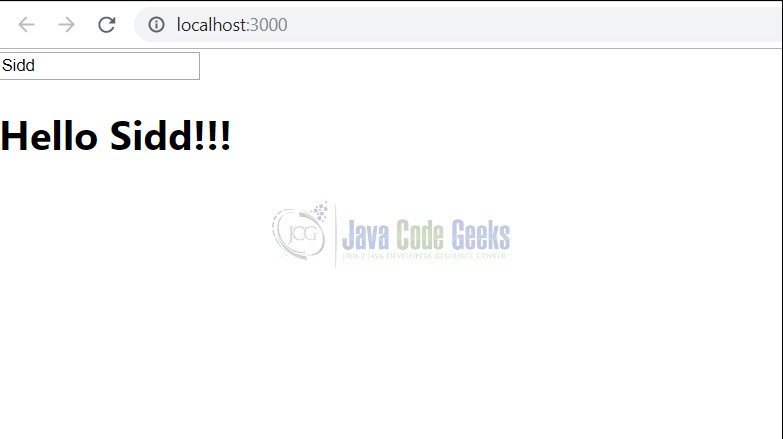
Angular supports two data binding. That is, any changes to the model reflect in the UI and any changes to the UI leads to changes in the backing model state as well. This is more convenient and easy to use.
10. Ajax Requests
React supports fetch to make Ajax requests to the server. With that said the support for fetch is sketchy right now. There are other options available like the Axios library for making Ajax requests to the server. Since React only makes up for the View in the MVC architecture of the Front end it does not address all the concerns for creating a complete application.
Angular has the HTTP module to provide a one stop solution to make Ajax requests to the server. Angular is a more complete solution to Enterprise application development. Although some like the freedom React gives to use different tools, libraries and frameworks for the Model and Controller aspects of the MVC architecture.
11. Memory Footprint
React library weighs in at ~100Kb minified and compressed which makes it quite light weight. Although there are complaints that the RAM requirements of React are higher owing to its implementation of the virtual DOM. The virtual DOM is an in memory replica of the real DOM albeit lighter than the actual DOM, still is quite taxing.
The Angular framework is a heavy weight with the library tipping the scales at ~500 kilobytes. With Angular’s holistic approach to application development and the feature set it offers it is inline with expectations. There is rarely, if any, need for multiple other frameworks, tools or libraries when using Angular. In contrast, developing solely with React is likely to hamper efforts.
12. Test driven Development
React supports test driven development like any framework out there. There are external tools and frameworks available out there to enable TDD with React. Some of the popular ones include Jest and Cypress that allow writing end to end tests for React applications.
Karma and protractor tools are effective while practicing TDD with Angular. Angular with its dependency injection feature enables testing components in isolation by injecting mock services. As well as writing end to end tests to test features.
13. Library vs Framework
React is a library and does not enforce opinion with regards to the rest of the stack. It gives freedom to select other tools, libraries and frameworks to complete the stack for development of a complete application. As an example we can use React for just a couple of widgets in an existing application. By just including a couple of script tags and our component JavaScript file like so:
<html> <head> <script src="https://unpkg.com/react@16/umd/react.production.js" crossorigin></script> <script src="https://unpkg.com/react-dom@16/umd/react- dom.production.js" crossorigin></script> <!-- Load our React component. --> <script src="my_component.js"></script7gt; </head> <body></body> </html>
Angular is a holistic framework with a comprehensive approach to enable Enterprise application development. Because of its rich set of features there is little, if any, need to look around for other tools, frameworks or libraries for additional features. The React example above wherein a React component or two could be used in an existing application is not possible with Angular. Angular is more of a all or nothing sort of deal.
14. React vs Angular – Conclusion
In this post we saw a React vs Angular comparison. We can say that React with its minimalist approach gives a lot of freedom and flexibility to decide our own stack. In converse, with its holistic approach the Angular framework leaves little else to desire. Both have their pros and cons. To decide between the two of them we need to be aware of our particular use case. To start off with a Enterprise Application from scratch the Angular framework appears favorite whereas in cases where we expect a lot of extensibility and expansion of features in the future the React framework looks like the winner.
This again? The horse is dead, please stop beating it.
React is view engine, angular is a framework. They’re both fine, time to stop brand building and go do something productive.