A guide to the InfluxDBMapper and QueryBuilder for Java Part: 1
With the release of latest influxdb-java driver version came along the InfluxbMapper.
To get started we need to spin up an influxdb instance, and docker is the easiest way to do so. We just follow the steps as described here.
Now we have a database with some data and we are ready to execute our queries.
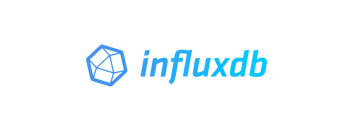
We have the measure h2o_feet
01
02
03
04
05
06
07
08
09
10
|
> SELECT * FROM "h2o_feet" name: h2o_feet -------------- time level description location water_level 2015 - 08 -18T00: 00 :00Z below 3 feet santa_monica 2.064 2015 - 08 -18T00: 00 :00Z between 6 and 9 feet coyote_creek 8.12 [...] 2015 - 09 -18T21: 36 :00Z between 3 and 6 feet santa_monica 5.066 2015 - 09 -18T21: 42 :00Z between 3 and 6 feet santa_monica 4.938 |
So we shall create a model for that.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
|
package com.gkatzioura.mapper.showcase; import java.time.Instant; import java.util.concurrent.TimeUnit; import org.influxdb.annotation.Column; import org.influxdb.annotation.Measurement; @Measurement (name = "h2o_feet" , database = "NOAA_water_database" , timeUnit = TimeUnit.SECONDS) public class H2OFeetMeasurement { @Column (name = "time" ) private Instant time; @Column (name = "level description" ) private String levelDescription; @Column (name = "location" ) private String location; @Column (name = "water_level" ) private Double waterLevel; public Instant getTime() { return time; } public void setTime(Instant time) { this .time = time; } public String getLevelDescription() { return levelDescription; } public void setLevelDescription(String levelDescription) { this .levelDescription = levelDescription; } public String getLocation() { return location; } public void setLocation(String location) { this .location = location; } public Double getWaterLevel() { return waterLevel; } public void setWaterLevel(Double waterLevel) { this .waterLevel = waterLevel; } } |
And the we shall fetch all the entries of the h2o_feet measurement.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
package com.gkatzioura.mapper.showcase; import java.util.List; import java.util.logging.Logger; import org.influxdb.InfluxDB; import org.influxdb.InfluxDBFactory; import org.influxdb.impl.InfluxDBImpl; import org.influxdb.impl.InfluxDBMapper; public class InfluxDBMapperShowcase { private static final Logger LOGGER = Logger.getLogger(InfluxDBMapperShowcase. class .getName()); public static void main(String[] args) { InfluxDBMapper influxDBMapper = new InfluxDBMapper(influxDB); List h2OFeetMeasurements = influxDBMapper.query(H2OFeetMeasurement. class ); } } |
After being successful on fetching the data we will continue with persisting data.
01
02
03
04
05
06
07
08
09
10
11
12
|
H2OFeetMeasurement h2OFeetMeasurement = new H2OFeetMeasurement(); h2OFeetMeasurement.setTime(Instant.now()); h2OFeetMeasurement.setLevelDescription( "Just a test" ); h2OFeetMeasurement.setLocation( "London" ); h2OFeetMeasurement.setWaterLevel( 1 .4d); influxDBMapper.save(h2OFeetMeasurement); List measurements = influxDBMapper.query(H2OFeetMeasurement. class ); H2OFeetMeasurement h2OFeetMeasurement1 = measurements.get(measurements.size()- 1 ); assert h2OFeetMeasurement1.getLevelDescription().equals( "Just a test" ); |
Apparently fetching all the measurements to get the last entry is not the most efficient thing to do. In the upcoming tutorials we are going to see how we use the InfluxDBMapper with advanced InfluxDB queries.
Published on Java Code Geeks with permission by Emmanouil Gkatziouras, partner at our JCG program. See the original article here: A guide to the InfluxDBMapper and QueryBuilder for Java Part: 1 Opinions expressed by Java Code Geeks contributors are their own. |