Factory Showdown: When One Becomes Many
In the bustling world of software development, factories are the unsung heroes. They churn out objects, the building blocks of your programs, ensuring consistency and efficiency. But what happens when one factory just isn’t enough? Enter the Factory Showdown, where the classic Factory pattern meets its more versatile counterpart, the Abstract Factory pattern.
This showdown isn’t about destruction, but evolution. Buckle up as we explore the limitations of the classic Factory and witness the rise of the Abstract Factory, a pattern that lets you create families of related objects, fostering flexibility and adaptability in your code.
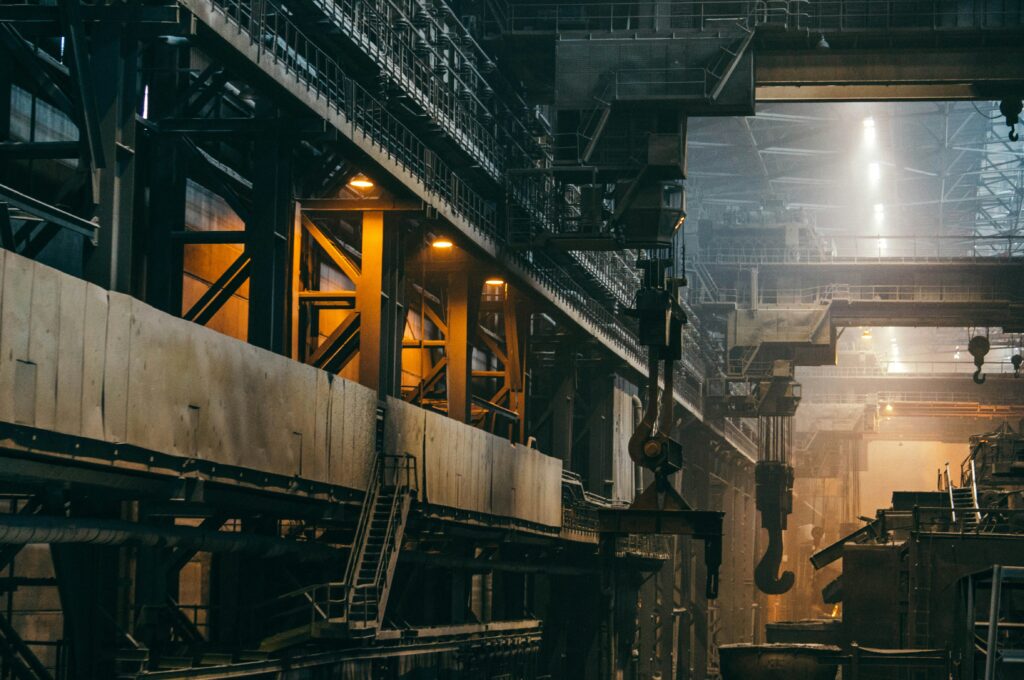
1. Introduction
Imagine building a mobile app. The Factory pattern would be like having a button press that always creates a new “message” object. It’s great for that one task, but if you wanted to create different objects like “photos” or “events” later, you’d have to rewrite parts of the app.
The Abstract Factory is like having a “content creator” section. You could choose a “message factory” to make messages, or a “photo factory” to make photos, all within the same section. This makes adding new content types much easier down the line.
2. The Humble Factory
In software development, creating objects is like building with Legos. You snap together different pieces (objects) to make something cool (your program). But imagine if you had to build a whole new creation station every time you wanted a different type of Lego piece. That’s kind of how things work without a factory pattern.
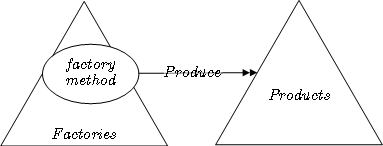
The Factory pattern acts like a dedicated Lego building station. Here’s how it works:
- Single Responsibility: This factory only focuses on one thing: creating a specific type of object. Let’s say it’s a “Car” object.
- Concrete Classes: The factory has all the instructions and parts (code) needed to build a Car object. It knows how to set the make, model, and year.
Here’s a simple code example (in Java) to illustrate this:
public class Car { private String make; private String model; private int year; public Car(String make, String model, int year) { this.make = make; this.model = model; this.year = year; } @Override public String toString() { return "Car: " + make + " " + model + " (" + year + ")"; } } // The Factory (only creates Car objects) public class CarFactory { public Car createCar(String make, String model, int year) { return new Car(make, model, year); } } // Usage public class Main { public static void main(String[] args) { CarFactory factory = new CarFactory(); Car car = factory.createCar("Honda", "Civic", 2023); System.out.println(car); // Output: Car: Honda Civic (2023) } }
The Java code shows:
- A
Car
class with attributes and methods. - A
CarFactory
that createsCar
objects with specific details. - A
Main
class using the factory to get a newCar
object, keeping the object creation logic separate.
Benefits of the Factory Pattern:
- Improved Readability: Your code is clearer because object creation logic is separated into the factory class.
- Maintainability: If you need to change how Car objects are created, you only modify the factory code, not the entire program.
- Loose Coupling: Your code doesn’t rely on the specific details of how Car objects are built, making it more flexible.
Limitations of the Factory Pattern:
- Limited Flexibility: This factory can only create Car objects. If you wanted to create Trucks or Motorcycles later, you’d need separate factories for each, which can become cumbersome.
- Adding New Object Types is Tricky: If you need a new object type (like Bicycle), you might have to change the existing factory or create a whole new one.
The Factory pattern is a great tool when you know exactly what objects you’ll need and they’re closely related. But if you need more flexibility to create different types of objects, then the Abstract Factory pattern might be a better champion for your coding needs!
3. The Rise of the Abstract Factory
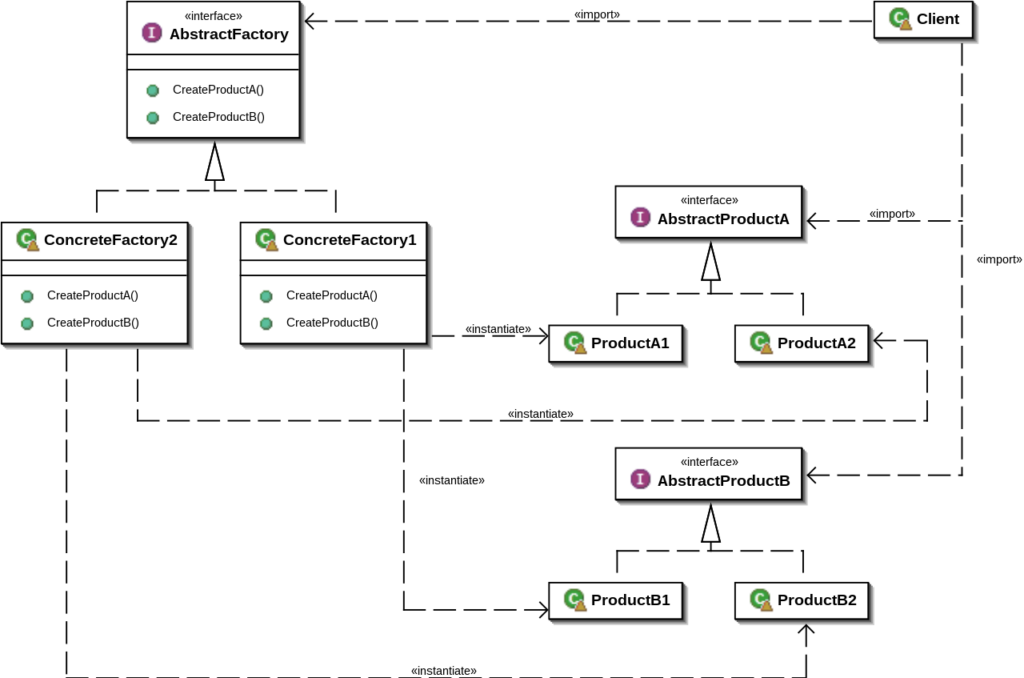
The Factory pattern was great for its dedicated creation stations, but what if you needed a whole workshop for different types of objects? Enter the Abstract Factory pattern, a more versatile champion.
Imagine you’re building furniture. The Factory pattern would be like having a dedicated chair-making station. The Abstract Factory, however, is like a furniture workshop with different sections:
- Chair Factory: Creates various chairs (dining, office, etc.)
- Table Factory: Creates different tables (coffee, dining, etc.)
Key Concepts of the Abstract Factory:
- Abstract Factory Interface: This acts like the workshop blueprint. It defines methods for creating each type of object in the family (e.g.,
createChair()
andcreateTable()
). - Concrete Factory Classes: These are the actual sections of the workshop, implementing the Abstract Factory interface. Each concrete factory specializes in creating a specific family of objects (e.g.,
ModernFurnitureFactory
andRusticFurnitureFactory
).
Benefits of the Abstract Factory Pattern:
- Increased Flexibility: You can easily switch between creating modern or rustic furniture families by using different concrete factories.
- Better Code Organization: The code is more organized because object creation logic for each family is grouped within its concrete factory.
- Scalability: Adding new families of objects (like lamps or bookshelves) is simpler because you just create a new concrete factory following the same interface.
Abstract Factory in Action: Building UIs for Different Platforms
Imagine building a mobile app. You might need different UI elements (buttons, menus) depending on whether it’s for Android or iOS. The Abstract Factory shines here:
- Abstract UIFactory: Defines methods for creating UI elements (e.g.,
createButton()
andcreateMenu()
). - AndroidUIFactory: Creates Android-specific buttons and menus.
- iOSUIFactory: Creates iOS-specific buttons and menus.
This allows you to choose the appropriate factory based on the target platform, keeping your code flexible and adaptable.
Code Snippet (Simplified Java Example):
// Abstract UIFactory defines methods for creating UI elements interface UIFactory { Button createButton(String text); Menu createMenu(String title); } // Concrete Factory (Android) creates Android-specific UI elements class AndroidUIFactory implements UIFactory { @Override public Button createButton(String text) { return new AndroidButton(text); } @Override public Menu createMenu(String title) { return new AndroidMenu(title); } } // Concrete Factory (iOS) creates iOS-specific UI elements class iOSUIFactory implements UIFactory { @Override public Button createButton(String text) { return new iOSButton(text); } @Override public Menu createMenu(String title) { return new iOSMenu(title); } } // Usage (choosing the right factory) UIFactory factory; if (isAndroid()) { factory = new AndroidUIFactory(); } else { factory = new iOSUIFactory(); } Button button = factory.createButton("Click Me"); // ... (similarly create menus)
This is a simplified example. In practice, the concrete factory classes would likely have more complex logic for creating the actual UI elements.
The Abstract Factory pattern empowers you to create families of related objects with ease, making your code more flexible and adaptable to changing requirements.
4. Choosing Your Champion
Imagine you’re building a house. Both the Factory and Abstract Factory patterns can help you construct different parts, but they excel in different scenarios. Here’s a breakdown to guide your decision:
Use the Factory Pattern When:
- Limited Set of Related Objects: If you know exactly what objects you need (doors, windows) and they’re closely related, the Factory pattern shines. It’s efficient for creating a specific type of object consistently.
- Simple Object Creation: The Factory pattern keeps things straightforward for well-defined object types. There’s no complex hierarchy of factories to manage.
Real-World Factory Pattern Examples:
- Document Creation: A factory might create different document objects (PDF, Word) based on the chosen format.
- Database Access: A factory could handle creating connections to different database types (MySQL, Oracle).
Use the Abstract Factory Pattern When:
- Flexibility is Key: If you need to create different families of objects (doors and windows for different architectural styles – modern, rustic), the Abstract Factory takes the crown. It allows you to easily switch between creating objects from different families.
- Scalability for Future Needs: The Abstract Factory makes adding new object families easier. You just create a new concrete factory that follows the same interface. This promotes code that’s adaptable to future requirements.
Real-World Abstract Factory Pattern Examples:
- UI Frameworks: Frameworks like Qt or GTK+ use Abstract Factories to create UI elements (buttons, menus) for different platforms (Windows, Linux).
- Game Development: Abstract Factories can handle creating different types of enemies or weapons depending on the game level or theme.
Choosing Wisely:
Think about the types of objects you need to create and how much flexibility you might require in the future. Here’s a simple analogy:
- Factory Pattern: Like having a dedicated oven that perfectly bakes delicious cookies (limited types).
- Abstract Factory Pattern: Like having a versatile kitchen appliance that can bake cookies, cakes, and even pizzas (different families of baked goods).
5. Conclusion
The Factory and Abstract Factory patterns are both valuable tools in your software development toolbox. They offer efficient and organized ways to create objects, but their strengths lie in different areas.
The Factory pattern excels when you need to create a limited set of well-defined, related objects. It simplifies object creation and promotes code readability.
The Abstract Factory pattern shines when flexibility is paramount. It allows you to create different families of objects with ease, making your code adaptable to changing requirements.