Unlocking Java 17 and Beyond with Spring Framework 6
Spring Framework, the de facto standard for enterprise Java development, has unveiled its latest iteration – Spring 6. This release marks a significant leap forward, not only embracing the power of Java 17 but also introducing a plethora of improvements that streamline development, enhance performance, and future-proof your applications. Let’s delve into the exciting new features of Spring 6 and explore how they can empower you to build robust and scalable applications.
1. Java 17: Unlocking the Potential
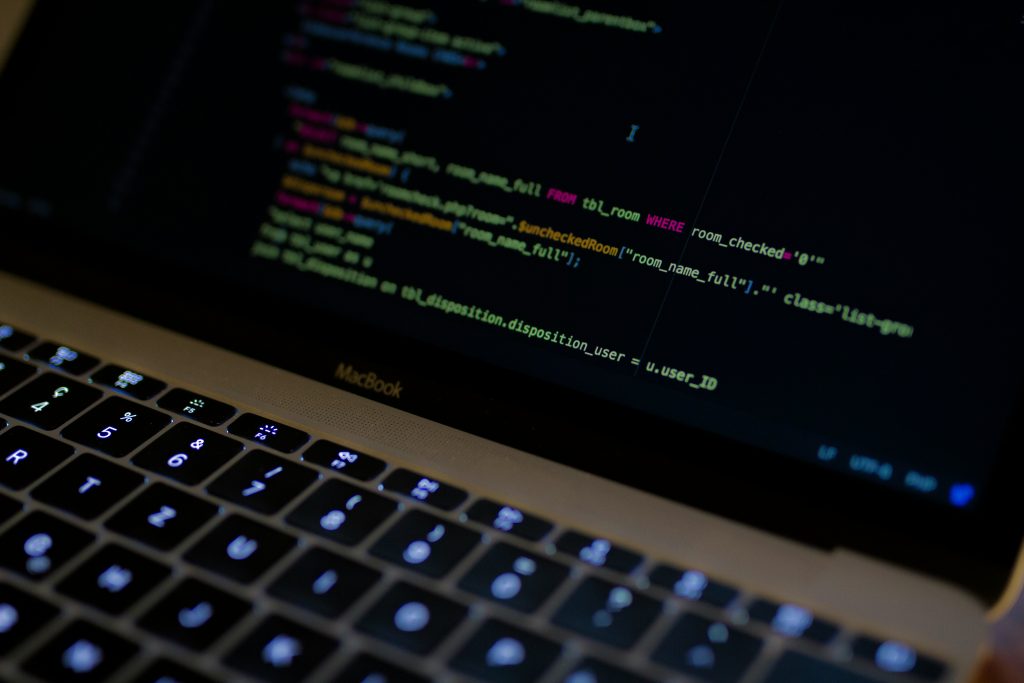
Spring 6 fully embraces Java 17, incorporating its powerful features seamlessly. Here are some key highlights:
- Records: Records introduce a concise and efficient syntax for creating immutable data classes, perfect for modeling simple data structures. Imagine creating a
Person
record with name and age:
record Person(String name, int age) {}
- Sealed Classes: Sealed classes restrict inheritance to a predefined set of subclasses, enhancing code safety and readability. This is valuable for modeling hierarchical relationships with clear boundaries.
- Switch Expressions: The new switch expressions provide a more readable and concise way to write conditional statements, making your code more elegant.
2. Reactive Revolution: Spring WebFlux Takes Center Stage
Spring WebFlux, the reactive web framework within Spring, receives a major boost in Spring 6. It now offers:
- Improved Performance: Spring WebFlux leverages Reactor 3.5, leading to significant performance enhancements for your reactive applications.
- Simplified Development: Streamlined APIs and better integration with other Spring components make reactive development more accessible.
- Enhanced Testing: Improved testing support ensures your reactive applications are robust and reliable.
Consider using Spring WebFlux for building highly scalable and responsive applications that excel under heavy loads, like real-time chat or data streaming services.
3. Security First: Spring Security 6.0 Fortifies Your Applications
Security remains paramount, and Spring 6 takes application protection to the next level with Spring Security 6.0:
- OAuth 3.0 Support: Spring Security now seamlessly integrates with OAuth 3.0, the latest standard for authorization, simplifying secure access management.
- Improved Web Application Firewall: The built-in Web Application Firewall (WAF) gets even stronger, protecting your applications from common attacks like SQL injection and cross-site scripting (XSS).
- Enhanced Authentication Providers: Spring Security supports various authentication providers like SAML and OpenID Connect out of the box, offering more flexibility for securing your applications.
Implement Spring Security 6.0 to safeguard your applications against evolving threats and ensure compliance with industry security standards.
4. Microservices Made Easy: Building Cloud-Native Apps with Spring Cloud 2023
Spring Cloud, a suite of tools for building microservices architectures, gets an update with Spring Cloud 2023:
- Simplified Service Discovery: Spring Cloud Alibaba integrates seamlessly, providing a powerful alternative to Netflix OSS for service discovery in cloud-native environments.
- Enhanced Configuration Management: Spring Cloud Config Server offers improved support for Git-based configuration management, making it easier to manage application configurations across environments.
- Streamlined API Gateway: Spring Cloud Gateway receives performance optimizations and new features, making it an even more robust choice for API management.
Utilize Spring Cloud to architect and deploy microservices that are modular, scalable, and resilient, perfectly suited for modern cloud environments.
5. Real World Examples
5.1 Java 17
Imagine an e-commerce application where you need to manage product information. Traditionally, you might define a Product
class like this:
private double price; private String description; public Product(String name, double price, String description) { this.name = name; this.price = price; this.description = description; } public String getName() { return name; } public void setName(String name) { this.name = name; } // Getters and setters for price and description omitted for brevity }
This approach works, but it’s verbose and requires writing getters and setters for each field. Records offer a cleaner and more efficient way to achieve the same functionality.
Here’s how a Product
record would look:
record Product(String name, double price, String description) {}
That’s it! This record defines all the necessary fields in a single line. No more boilerplate code for getters and setters. Accessing data is also streamlined:
Product phone = new Product("Pixel 7", 699.00, "The latest flagship from Google"); String phoneName = phone.name(); // Access name directly using dot notation double phonePrice = phone.price();
Benefits in the E-commerce Context:
- Improved Readability: Records make code more concise and easier to understand, especially for developers new to the project.
- Reduced Boilerplate: No need to write getters and setters, saving development time and effort.
- Efficient Data Access: Direct field access eliminates the need for unnecessary method calls, potentially improving performance.
- Immutability: Records are immutable by default, ensuring data integrity in your e-commerce application. This is crucial for maintaining product details like price and availability.
Beyond Basic Data Access:
Records can be used for more than just basic data access. You can leverage their features to:
- Define custom methods: Add methods specific to product logic, like calculating discounts or checking inventory.
- Integrate with Spring Data JPA: Use records seamlessly with Spring Data JPA for efficient data persistence and retrieval in your e-commerce application.
Conclusion:
Records offer a significant improvement over traditional class syntax for modeling data in Java applications. In the context of an e-commerce application, they simplify data access, reduce boilerplate code, and enhance code readability. By embracing records and other advancements in Java 17 and Spring 6, you can develop cleaner, more maintainable, and efficient e-commerce applications that delight your users.
5.2 Spring WebFlux vs. Blocking: Streaming Data Efficiently
In this example we will showcase a code snippet demonstrating a simple Spring WebFlux endpoint handling data streaming, highlighting its performance benefits compared to traditional blocking approaches
Traditional Blocking Approach:
Imagine a REST endpoint that retrieves and returns a list of 1000 product reviews from a database. Here’s a simplified example using Spring MVC:
@Controller public class ProductReviewController { @GetMapping("/reviews") public List<Review> getReviews() throws Exception { // Simulate database access: List<Review> reviews = reviewService.findAll(); return reviews; } }
This approach fetches all reviews at once, potentially blocking the server thread until the entire list is retrieved. This can be inefficient, especially for large datasets or under heavy load.
Spring WebFlux to the Rescue:
With Spring WebFlux, we can leverage reactive programming to handle the data stream efficiently. Here’s an alternative using WebFlux:
@Controller @RequestMapping("/reviews") public class ReactiveReviewController { @GetMapping public Flux<Review> getReviews() { return reviewService.findAllFlux(); } }
This code retrieves reviews asynchronously and emits them as a Flux
, a stream of data elements. The server can send each review to the client as soon as it’s available, without blocking the thread.
Performance Benefits:
- Non-blocking: Spring WebFlux avoids blocking threads, allowing the server to handle multiple requests concurrently, improving responsiveness and scalability.
- Backpressure: WebFlux supports backpressure, automatically adjusting the data flow based on the client’s capacity, preventing overwhelming the client with excessive data.
- Efficient Data Processing: Reactive libraries like Reactor optimize data processing, handling large datasets more efficiently than traditional approaches.
Code Snippet Comparison:
Feature | Traditional Blocking | Spring WebFlux |
---|---|---|
Data Access | Blocks the thread until all data is retrieved. | Retrieves data asynchronously in a stream. |
Data Return | Returns the entire list at once. | Emits data elements one by one as they become available. |
Thread Usage | Blocks a server thread. | Non-blocking, allowing concurrent requests. |
Scalability | Limited by available threads. | Handles large volumes of data efficiently. |
This is a simplified example. Real-world scenarios might involve filtering, transforming, or combining data streams, further highlighting the power of reactive programming.
By adopting Spring WebFlux and reactive approaches, you can build highly responsive and scalable web applications that efficiently handle data streams, especially for real-time scenarios or applications dealing with large datasets.
5.3 Secure Social Media Login with Spring Security and OAuth 3.0
Imagine a social media application where users can sign in using their existing accounts from popular platforms like Google, Facebook, or Twitter. Traditionally, managing these logins involved developing and maintaining separate authentication mechanisms for each platform, increasing complexity and potential security risks.
Enter OAuth 3.0 and Spring Security:
OAuth 3.0 provides a standardized protocol for secure authorization, allowing users to grant access to their data on one platform (e.g., Google) to another platform (e.g., your social media app) without sharing their password. Spring Security seamlessly integrates with OAuth 3.0, simplifying and securing user authentication in your application.
Here’s how it works:
- User initiates login: The user clicks the “Login with Google” button on your social media app.
- Redirection to Google: Your application redirects the user to Google’s authorization server.
- User grants access: The user logs in to Google and grants your application specific permissions (e.g., access to profile information).
- Authorization code exchange: Google sends an authorization code back to your application.
- Token retrieval: Your application exchanges the code for an access token from Google.
- User authentication: Your application uses the access token to authenticate the user without requiring their Google password.
- User information retrieval: Optionally, your application can retrieve basic user information from Google for profile creation.
Benefits of using OAuth 3.0 with Spring Security:
- Simplified Authentication: No need to develop and maintain separate login mechanisms for each platform.
- Improved Security: Users don’t share their passwords with your application, reducing the risk of credential theft.
- Standardized Protocol: Ensures interoperability with various social media platforms.
- Reduced Development Time: Spring Security handles most of the OAuth 3.0 complexities.
- Enhanced User Experience: Seamless login with familiar platforms improves user satisfaction.
Code Snippet Example:
Here’s a simplified code snippet demonstrating how Spring Security handles OAuth 3.0 login with Google:
@Configuration public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/login", "/error").permitAll() .anyRequest().authenticated() .and() .oauth2Login() .loginPage("/login") .defaultSuccessUrl("/home") .clientRegistration() .clientId("...") // Google client ID .clientSecret("...") // Google client secret .provider("google") .build(); } }
This code configures Spring Security to use Google login with OAuth 3.0 and redirect successful logins to the /home
page.
Real-world implementations might involve additional configurations, handling user data securely, and integrating with your application’s user management system.
By leveraging Spring Security and OAuth 3.0, you can create secure and convenient user authentication in your social media application, enhancing user trust and reducing development complexity.
5.4 Spring Cloud Alibaba Simplifies Service Discovery in a Travel Booking Microservices System
Imagine a microservices-based travel booking system where various services handle functionalities like flight search, hotel booking, and payment processing. Service discovery becomes crucial for these services to communicate and collaborate effectively. Here’s how Spring Cloud Alibaba simplifies this process:
Traditional Service Discovery:
- Netflix OSS: Many developers traditionally use Netflix OSS tools like Eureka for service discovery. While functional, managing and scaling Eureka clusters can be complex, especially in large deployments.
- Challenges: Centralized server (Eureka) becomes a single point of failure, and scaling it can be cumbersome.
Spring Cloud Alibaba to the Rescue:
- Alibaba Service Registry: Spring Cloud Alibaba integrates seamlessly with Alibaba Cloud’s Service Registry, a highly scalable and reliable service discovery solution.
- Benefits:
- Distributed: No single point of failure, ensuring high availability.
- Elastic: Scales automatically based on traffic demands.
- Integrated with Alibaba Cloud: Leverages Alibaba Cloud infrastructure for enhanced performance and security.
Example: Flight Search and Hotel Booking:
- Microservices Register: The flight search and hotel booking microservices register themselves with the Alibaba Service Registry.
- Service Discovery: When a user searches for flights, the booking service discovers the flight search service using the registry.
- Communication and Data Exchange: The booking service retrieves flight information from the search service and presents it to the user.
- Similar process for Hotel Booking: The booking service discovers and communicates with the hotel booking service to complete the reservation.
Additional Benefits:
- Simplified Configuration: Spring Cloud Alibaba provides convenient configuration management tools for microservices.
- Resilience and Fault Tolerance: Built-in mechanisms handle service failures and ensure system uptime.
- Monitoring and Observability: Integrated tools for monitoring microservices and troubleshooting issues.
Code Snippet Example:
Here’s a simplified code snippet demonstrating service registration with Alibaba Service Registry:
@Configuration public class ServiceConfig extends AlibabaRegistrationConfig { @Override public ServiceRegistry getRegistry() { return new NacosServiceRegistry(); // Use Nacos, Alibaba's service registry } }
This code configures a microservice to register with the Nacos registry, a component of Alibaba Service Registry.
By adopting Spring Cloud Alibaba, you can simplify service discovery, enhance scalability, and leverage the robust features of Alibaba Cloud in your microservices-based travel booking system (or any other microservices architecture). This leads to a more reliable, resilient, and ultimately better user experience for your customers.
5. Conclusion: Spring into the Future with Spring 6
Spring Framework 6 represents a significant leap forward, empowering developers to build high-performance, secure, and future-proof applications. By embracing Java 17, reactive programming with Spring WebFlux, robust security with Spring Security, and microservices architecture with Spring Cloud, you can unlock new possibilities and stay ahead of the curve. So, dive into Spring 6 and embark on your journey to building the next generation of innovative applications!
Remember, this is just a starting point. Spring 6 offers a vast array of features and improvements beyond what we’ve covered. Explore the official documentation, online tutorials, and community resources to delve deeper and unlock the full potential of Spring 6 for your next development project.