Patching Null++
Here’s a curiosity.
In my current project we’re looking at making HTTP PATCH requests. If we’re strictly following the REST standards, we might do things differently, but in our use case, a PATCH is a partial update, caused by discovering some fields of our object to have changed.
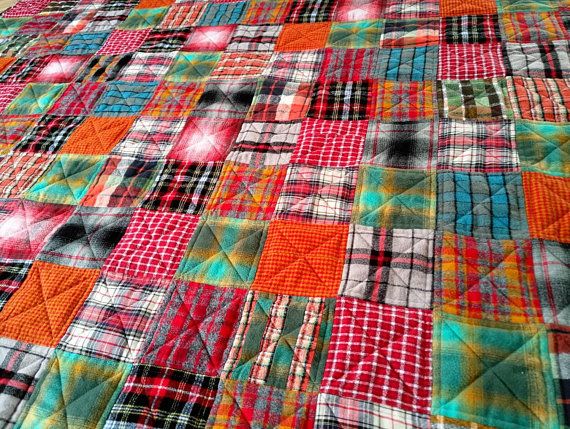
Imagine the object:
{ "id": 123, "status": "ACTIVE", "isInternational": false, "customer": { "name": "Mr Foo", "rank": "A", "nextOrder": 987 } }
If the status changed, you might imagine an update that reads like this:
{ "id": 123, "status": "INACTIVE", "customer": { "nextOrder": null } }
This partial object can be merged with the existing object to produce the change we want.
Null vs Not Mentioned
If we loaded the partial object into a strongly typed object (in Java, say), then the object would have null
all over the place where bits were missing.
If we wanted to take that loaded partial object and apply it to the data, we’d have a problem. Any null
in that loaded version would be ambiguous. Would it mean:
- Please set this field to
null
? - The request didn’t mention the field
Clearly you can’t load a partial into strong type, or a template of the whole object, and keep its meaning as a delta to an existing object.
Boolean?
This leads to another weird thought. Many people incorrectly think that boolean
has two states:
- True
- False
While this is true, it’s quite common to find situations where there’s a hidden third state of null
. Tri-state booleans are an anti-pattern, but if assembling data from multiple sources, or in cases where some fields are themselves conditional on others, it may be unsurprising to find a boolean value set to null
.
It turns out that with the above, there’s a fourth state – not mentioned.
That’s ridiculous!
Published on Java Code Geeks with permission by Ashley Frieze, partner at our JCG program. See the original article here: Patching Null++ Opinions expressed by Java Code Geeks contributors are their own. |
You just found out what JavaScript models as null vs undefined. Here’s a valid TypeScript snippet for a boolean:
const a: boolean = true;
const b: boolean = false;
const c: boolean = null;
const d: boolean = undefined;
Great point. And once you add
truthy
andfalsy
to boolean, then life becomes even more nuanced!