Missing Parameterized Test
Sometimes, what you need is a parameterized test. They do the job of representing a single way of testing with an input table full of use cases and expected outcomes.
In some ways, a test suite is just a set of inputs and expected outputs, but a parameterized test is one where the execution of the test is pretty uniform.
It may be tempting to handle subtle variants in parameterized tests by putting some conditional logic in a generic test, fed loads of diverse inputs, but be wary of that.
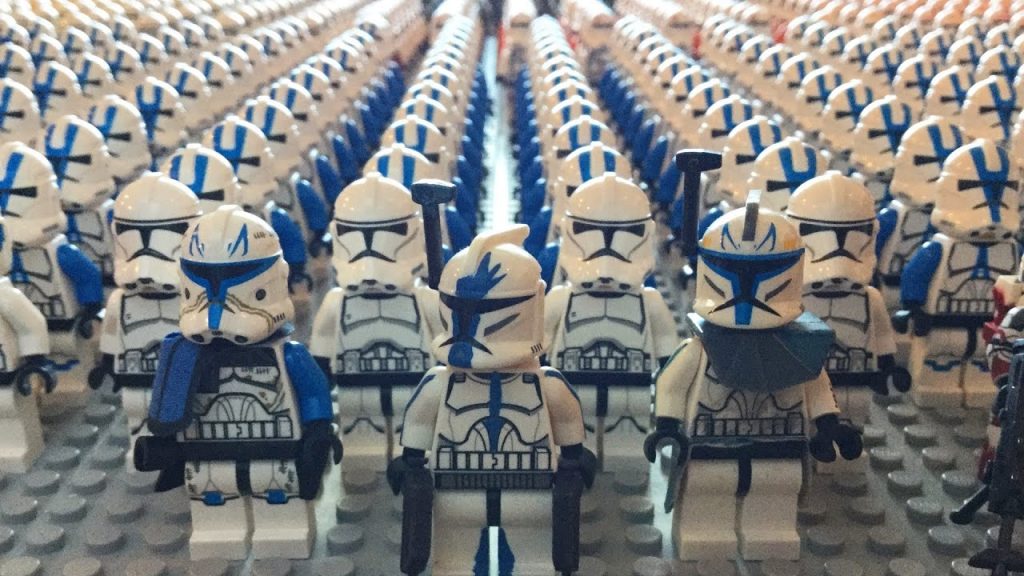
If You Don’t Use Parameterized Tests
You’re missing out on:
- Expressing groups of related tests in a more structured way
- Using the table of inputs/outputs as a way to help you spot gaps in your tests, think up more use cases, and cheaply add them
- Less test code to maintain
Do I Need A Parameterized Test?
Symptoms:
- A family of tests have the same test code
- The names of the tests end up with
_example2
or similar on them – suggesting that you’re having a test naming problem, but also suggesting that there’s no real distinction between the use cases, other than the data
But… if there’s only a 2 or 3 examples, then perhaps the parameterized test is overkill.
What Do They Look Like?
An example in JUnit 5:
01 02 03 04 05 06 07 08 09 10 11 | @ParameterizedTest @CsvSource ({ "a,A" , "b,B" , "bbb,BBB" , "bBbB,BBBB" }) void capitalizerTurnsInputToCapitals(String input, String expected) { assertThat(Capitalizer.toCapitals(input)) .isEqualTo(expected); } |
Published on Java Code Geeks with permission by Ashley Frieze, partner at our JCG program. See the original article here: Missing Parameterized Test Opinions expressed by Java Code Geeks contributors are their own. |
It is a theoretical example with strings as in almost every documentation. Bad (or maybe good for type safety) is that Java annotations do not support method references. JUnit5 is just a new JUnit4. A pure Java framework with all limitations of Java. See this example with Spock/Groovy https://github.com/Tibor17/dropwizard-rest-samples/blob/master/spock-tests/src/test/groovy/x/AcceptanceFeatureIT.groovy#L69 in Spock the parameterized stuff is used as a code. Java annotation support only compile time constants and therefore no code in annotations which real programmers in companies need in real life. Having listeners is a glue code in Java framework because Java has these limitations but Groovy does not. As… Read more »
I think that JUnit5 supports other forms of parameter sources, allowing you to express parameters outside of the limitations of annotations. That said, you’re right, it gets long-winded. In my article https://codingcraftsman.wordpress.com/2016/11/30/so-you-want-to-write-bdd-tests/ – I refer to other test running, including SpectrumBDD, which use a functional-style of programming to create tests, and may solve the paramterized test problem better for you. See https://github.com/greghaskins/spectrum/blob/master/docs/GherkinDSL.md, particularly the
withExamples
piece.