Python String split() Example
1. Introduction
Manipulating strings can be a very important aspect of programming due to the various number of ways in which strings can be manipulated. And one such way of manipulating strings is by splitting them based on several characters. You might want to split strings based on commas, semicolons and so much more. In our article for today, we would be looking at how the split
method in Python can help manipulate strings.
The method split
generally helps achieve the task of breaking strings into smaller chunks based on certain strings. You might have a long string grouped by a certain character and you want to break the string based on that character. That is where the method split
comes into play.
1.1 Syntax
The split
method is a part of the String
class in Python.
S.split(separator, maxsplit) -> List of Strings
The first thing you need is a string you want to split. Then the string you want to use as the separator. If no separator or None
is passed, whitespace is assumed as the separator. Then the maxsplit parameter is used to define how many splits should be done. By default, it splits into as many strings as possible based on the separator.
And the result is a list of the chunks of the string formed by breaking the initial string based on the separator.
2. Examples
Now, let us look at some examples of the method split
in action.
2.1 Python String split() Example 1
Imagine you have the string “What Do We Do Now”. This string is represented in memory as follows:
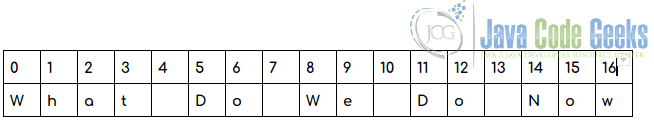
Now, imagine we have the following code
name = 'What Do We Do Now' name.strip('o', 2)
The above code means that we break the string into chunks. We break the string anywhere we see the letter ‘o’, in this case, the letter ‘o’ is our separator.
Now, we also pass in the number 2. The number 2 means that we can only split 2 times. So how does the python languages execute this task?
It begins until it gets to index 6 which is where the letter ‘o’ is found. Then it breaks a chunk of the string from index 0 to index 5 and puts it inside a list.
So the first chunk inside the list would be:
['What D']
Then the next thing we do is to continue until we find another letter ‘o’ at index 12, we break the chunk from the last ‘o’ at index 6 to index 12 and put into our list. Now we have 2 chunks of strings inside our list.
['What D', ' We D']
Remember, we limited the number of splits we are going to do to 2. So at this point, we stop splitting and return every other remaining string as a single chunk.
['What D', ' We D', ' Now']
You would realize that there is a letter “o” that could have been split, but we could not do that because the split was limited to 2 times.
And the final list returned contains only 3 chunks of strings.
2.2 Python String split() Example 2
Consider that a student name was entered all as one text and we need to break it into the student’s first name, last name, and other names.
studentName = 'Musa Kabuga Mailafia'
And when we call the split method without passing in any parameter
studentName.split()
The string is represented in memory as:

Since no explicit separator is used, the whitespace is used as the default separator, and the split is done till the end of the string since the maximum number of splits was not explicitly defined.
And the result would be:
['Musa', 'Kabuga', 'Mailafia']
Meaning that the student name is split by default based on the whitespace and a list of three strings is returned.
2.3 Python String split() Example 3
Consider another example where a student passes 3 strings for his/her name, but we only need to split the name into first name and last name. As such, we only want to split once. Split into his/her first name and every other string is the last name.
studentName = 'Faiz Bashir Sheik'
Then we call the split
method, but only pass the parameter to limit the number of strings to be returned in the list.

studentName.split(maxsplit = 1)
Since the maximum number of splits was specified, the string is split only once. And since the separator was not passed, the whitespace is used by default. As such, the result that would be returned in this case would be:
['Faiz', 'Bashir Sheik']
2.4 Python String split() Example 4
Consider the following:
string_value = 'How,Do,You,Do,Kabir'
And we want to split the string since we do not want to have the commas in the string.
To start, we first visualize how it would look like in memory. In memory, it would look like this:

Then we call the split function with the separator we want to use:
string_value.split(",")
And the result returned would be:
['How', 'Do', 'You', 'Do', 'Kabir']
2.5 Python String split() Example 5
Let us consider the following example
student_address = '11, Malali Str; 10; Afr'
Imagine what you need is the address which is the first chunk of the string before the first semi-colon. How do you go ahead with this?
Imagine the string first in memory

Then we call the split
function with the semicolon (;) as the separator, then the maximum splits needed as 1. So the function looks as follows:
student_address.split(';', maxsplit = 1)
And the result returned would be:
['11, Malali Str', '10; Afr']
2.6 Python String split() Example 6
When the separator to be used to break the string does not exist in the string, what happens?
Let us look at one more example.
student_name = 'Alameen Ibrahim'
And we want to split the string based on the separator ‘,’, and the separator does not exist in the string.
student_name.split(',')
Can you guess what the output would be?
['Alameen Ibrahim']
A list containing just the exact string that was to be split would be returned.
3. Python String split() – Conclusion
Manipulating strings can be a very stressful operation if we do not know the right tools to use. So in Python, one of such tools that enable us to manipulate strings is the split
method. It allows to break a string into chunks based on a separator and also gives a maximum number of splits that can be done at any given time.
4. Download the Source Code
This was an example of how to use the split
method in Python
You can download the full source code of this example here: Python String split() Example