A guide to the InfluxDBMapper and QueryBuilder for Java Part: 2
Previously we setup an influxdb instance running through docker and we also run our first InfluxDBMapper code against an influxdb database.
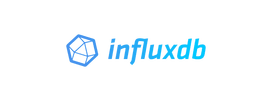
The next step is to execute some queries against influxdb using the QueryBuilder combined with the InfluxDBMapper.
Let’s get started and select everything from the table H2OFeetMeasurement.
01
02
03
04
05
06
07
08
09
10
|
private static final String DATABASE = "NOAA_water_database" ; public static void main(String[] args) { InfluxDBMapper influxDBMapper = new InfluxDBMapper(influxDB); Query query = select().from(DATABASE, "h2o_feet" ); List h2OFeetMeasurements = influxDBMapper.query(query, H2OFeetMeasurement. class ); } |
Let’s get more specific, we will select measurements with water level higher than 8.
1
2
3
|
Query query = select().from(DATABASE, "h2o_feet" ).where(gt( "water_level" , 8 )); LOGGER.info( "Executing query " +query.getCommand()); List higherThanMeasurements = influxDBMapper.query(query, H2OFeetMeasurement. class ); |
I bet you noticed the query.getCommand() detail. If you want to see the actual query that is being executed you can call the getCommand() method from the query.
Apart from where statements we can perform certain operations on fields such as calculations.
1
2
3
|
Query query = select().op(op(cop( "water_level" ,MUL, 2 ), "+" , 4 )).from(DATABASE, "h2o_feet" ); LOGGER.info( "Executing query " +query.getCommand()); QueryResult queryResult = influxDB.query(query); |
We just used the cop function to multiply the water level by 2. The cop function creates a clause which will execute an operation to a column. Then we are going to increment by 4 the product of the previous operation by using the op function. The op function creates a clause which will execute an operation with regards to two arguments given.
Next case is to select using a specific string field key-value
1
2
3
|
Query query = select().from(DATABASE, "h2o_feet" ).where(eq( "location" , "santa_monica" )); LOGGER.info( "Executing query " +query.getCommand()); List h2OFeetMeasurements = influxDBMapper.query(query, H2OFeetMeasurement. class ); |
Things can get even more specific and select data that have specific field key-values and tag key-values.
1
2
3
4
5
6
7
8
|
Query query = select().column( "water_level" ).from(DATABASE, "h2o_feet" ) .where(neq( "location" , "santa_monica" )) .andNested() .and(lt( "water_level" ,- 0.59 )) .or(gt( "water_level" , 9.95 )) .close(); LOGGER.info( "Executing query " +query.getCommand()); List h2OFeetMeasurements = influxDBMapper.query(query, H2OFeetMeasurement. class ); |
Since influxdb is a time series database it is essential to issue queries with specific timestamps.
1
2
3
4
|
Query query = select().from(DATABASE, "h2o_feet" ) .where(gt( "time" ,subTime( 7 ,DAY))); LOGGER.info( "Executing query " +query.getCommand()); List h2OFeetMeasurements = influxDBMapper.query(query, H2OFeetMeasurement. class ); |
Last but not least we can make a query for specific fields. I will create a model just for the fields that we are going to retrieve.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
package com.gkatzioura.mapper.showcase; import java.time.Instant; import java.util.concurrent.TimeUnit; import org.influxdb.annotation.Column; import org.influxdb.annotation.Measurement; @Measurement (name = "h2o_feet" , timeUnit = TimeUnit.SECONDS) public class LocationWithDescription { @Column (name = "time" ) private Instant time; @Column (name = "level description" ) private String levelDescription; @Column (name = "location" ) private String location; public Instant getTime() { return time; } public void setTime(Instant time) { this .time = time; } public String getLevelDescription() { return levelDescription; } public void setLevelDescription(String levelDescription) { this .levelDescription = levelDescription; } public String getLocation() { return location; } public void setLocation(String location) { this .location = location; } } |
And now I shall query for them.
1
2
|
Query selectFields = select( "level description" , "location" ).from(DATABASE, "h2o_feet" ); List locationWithDescriptions = influxDBMapper.query(selectFields, LocationWithDescription. class ); |
As you can see we can also map certain fields to a model. For now mapping to models can be done only when data come from a certain measurements. Thus we shall proceed on more query builder specific examples next time.
You can find the source code on github.
Published on Java Code Geeks with permission by Emmanouil Gkatziouras, partner at our JCG program. See the original article here: A guide to the InfluxDBMapper and QueryBuilder for Java Part: 2 Opinions expressed by Java Code Geeks contributors are their own. |