Build Reactive REST APIs with Spring WebFlux – Part2
In continuation of the last post, in this article, we will see the reactive streams specification and one of its implementation called Project Reactor. Reactive Streams specification has the following interfaces defined. Let us see the details of those interfaces.
- Publisher → A Publisher is a provider of a potentially unlimited number of sequenced elements, publishing them as requested by its Subscriber(s)
public interface Publisher<T> { public void subscribe(Subscriber<? super T> s); }
- Subscriber → A Subscriber is a consumer of a potentially unbounded number of sequenced elements.
public interface Subscriber<T> { public void onSubscribe(Subscription s); public void onNext(T t); public void onError(Throwable t); public void onComplete(); }
- Subscription → A Subscription represents a one-to-one lifecycle of a Subscriber subscribing to a Publisher.
public interface Subscription { public void request(long n); public void cancel(); }
- Processor → A Processor represents a processing stage — which is both a Subscriber and a Publisher and obeys the contracts of both.
The class diagram of the reactive streams specification is given below.
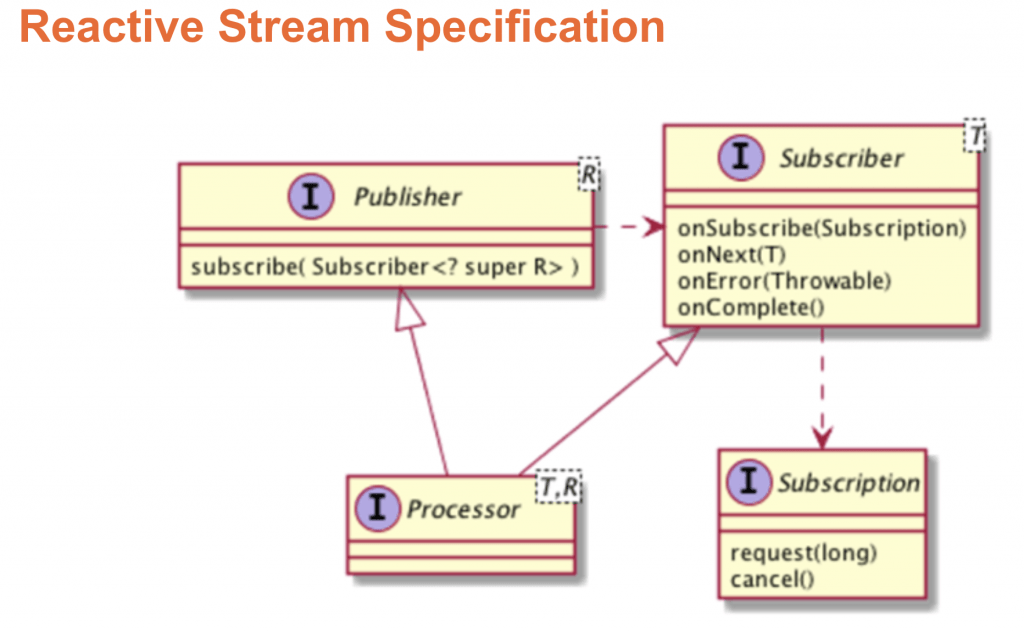
The reactive streams specification has many implementations. Project Reactor is one of the implementations. The Reactor is fully non-blocking and provides efficient demand management. The Reactor offers two reactive and composable APIs, Flux [N] and Mono [0|1], which extensively implement Reactive Extensions. Reactor offers Non-Blocking, backpressure-ready network engines for HTTP (including Websockets), TCP, and UDP. It is well-suited for a microservices architecture.
- Flux → It is a Reactive Streams
Publisher
with rx operators that emits 0 to N elements, and then complete (successfully or with an error). The marble diagram of the Flux is represented below.
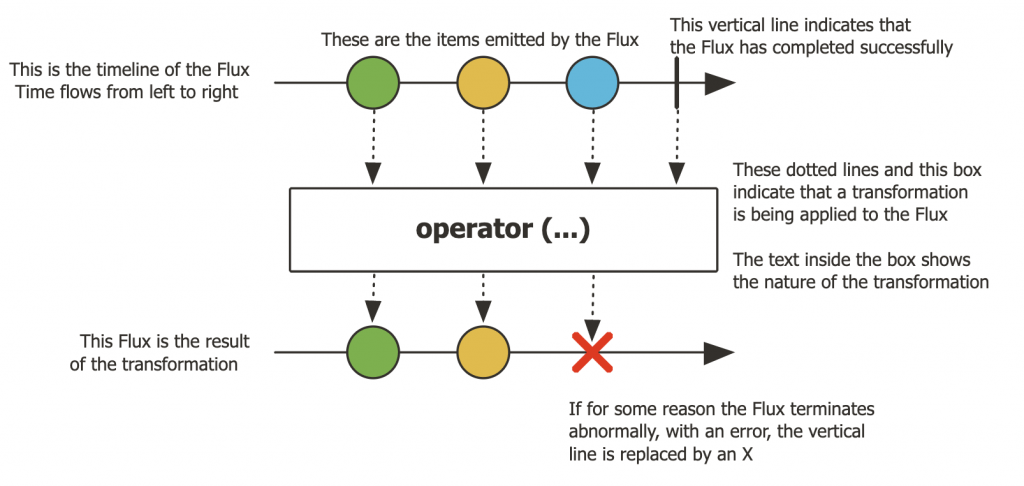
- Mono → It is a Reactive Streams
Publisher
with basic rx operators that completes successfully by emitting 0 to 1 element, or with an error. The marble diagram of the Mono is represented below.
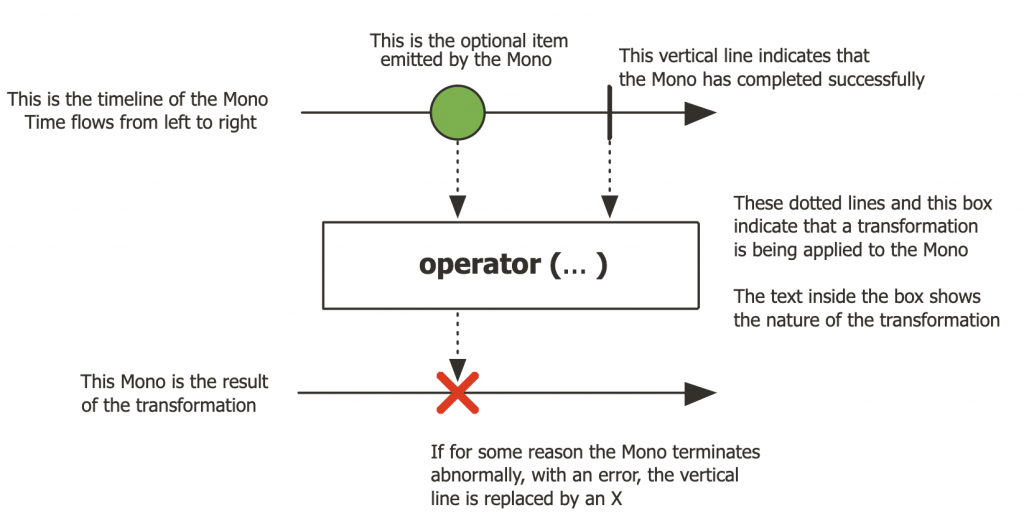
As Spring 5.x comes with Reactor implementation, if we want to build REST APIs using imperative style programming with Spring servlet stack, it still supports. Below is the diagram which explains how Spring supports both reactive and servlet stack implementations.
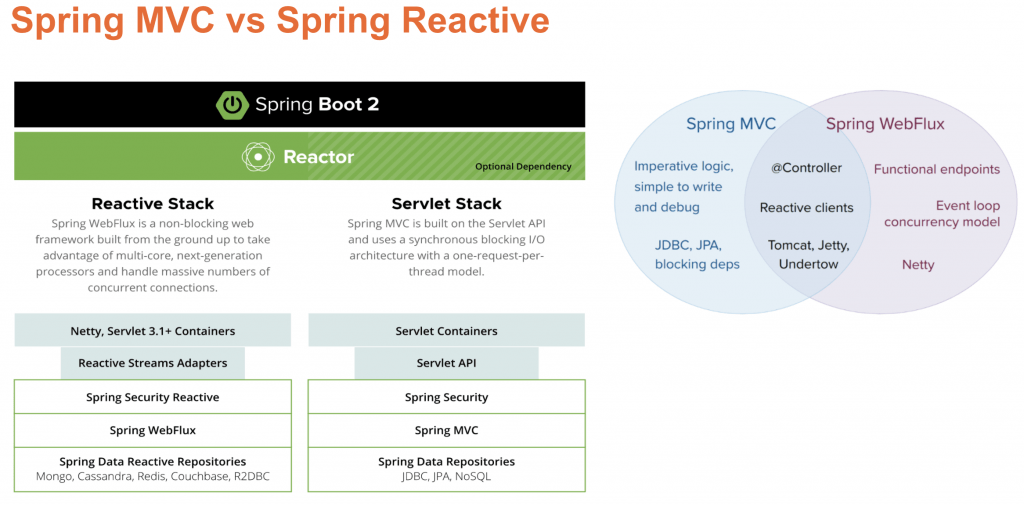
In the coming article, we will see an example application with reactive APIs. Until then, Happy Learning!!
Published on Java Code Geeks with permission by Siva Janapati, partner at our JCG program. See the original article here: Build Reactive REST APIs with Spring WebFlux – Part2 Opinions expressed by Java Code Geeks contributors are their own. |