Spring boot custom banner generation
Whenever we start a Spring Boot application a text message shown below is displayed . This is called as a banner.
Now, wouldn’t it be wonderful if we could create a custom banner which is specific to our Spring Boot application and use it instead of default Spring Boot banner. There are many ways to generate and use spring boot custom banner.
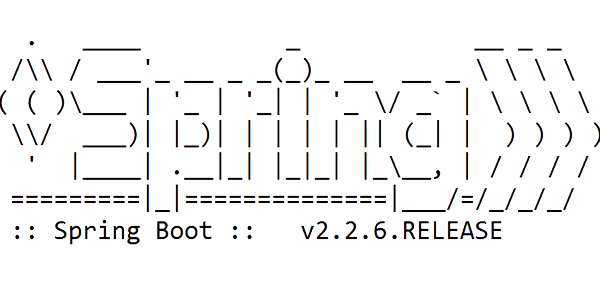
We will cover following topics in detail,
- Custom spring boot banner generator and generation
- Configure spring boot application to use custom banner
- Disable spring boot banner
1. Custom spring boot banner generator and generation
For showing the custom banner at application startup we need a banner.
We can create custom banner by our self in text file, can create programatically or use various online free tools.
We can have banner in plain text file by using the Ascii charters or in image format.
The banner in the plain text format is faster to load and easier to maintain. So in this blog we will be using text banner but you can use as per your choice.
1.1 Spring boot custom banner online generator:
There are so many Ascii banner generator tools are available online like,
- https://devops.datenkollektiv.de/banner.txt/index.html
- http://www.network-science.de/ascii/
- https://textkool.com/en/ascii-art-generator?hl=full&vl=full&font=Red%20Phoenix&text=stacktraceguru
- http://patorjk.com/software/taag/#p=display&f=Doom&t=stacktraceguru
- https://manytools.org/hacker-tools/ascii-banner/
- https://www.kammerl.de/ascii/AsciiSignature.php
1.2 Programmatic banner generation:
Spring boot framework provides a Banner interface that allows us to create banners.
We need a class that implements Banner interface and overrides printBanner() method for configuring custom banner.
01 02 03 04 05 06 07 08 09 10 11 | import java.io.PrintStream; import org.springframework.boot.Banner; import org.springframework.core.env.Environment; public class CustomBanner implements Banner { @Override public void printBanner(Environment arg0, Class<?> arg1, PrintStream arg2) { arg2.println( "###############################" ); arg2.println( "###### Spring boot banner ######" ); arg2.println( "###############################" ); } } |
The most important thing to note is that, the banner configured in printBanner() method will only be used if we do not have configured banner in properties or banner file.
In the banner, we can use following placeholders for dynamic values.
Variable | Description |
---|---|
${application.version} | Displays application version number e.g. 1.0 |
${application.formatted-version} | Displays application version number with bracket and v e.g. (v1.0) |
${spring-boot.version} | Displays Spring Boot version e.g. 2.2.7.RELEASE |
${spring-boot.formatted-version} | Displays Spring Boot version with bracket and v e.g. (v2.2.7.RELEASE) |
${application.title} | Displays application titleas declared in MANIFEST.MF. e.g. MyApp. |
2. Configure spring boot application to use custom banner
After the banner is generated, we need to make it available for application to use.
By default spring boot uses file named banner.txt or banner.(png|jpg|gif) in the src/main/resources directory.
We can store at this location the file with name banner.txt.
We can also store in different location with any file name.
If we decide to store in other than src/main/resources/banner.txt, we need to configure the file location so that application can use it.
2.1 We can configure using following properties:
1 2 | spring.banner.image.location=classpath:custom-banner.png spring.banner.location=classpath:/path/bannerfile.txt |
2.2 Configure banner by a program:
We can configure banner in SpringApplication class using setBanner() method.
1 2 3 4 5 6 7 8 | @SpringBootApplication public class BootApplication { public static void main(String[] args) { SpringApplication application = new SpringApplication(BootApplication. class ); application.setBanner( new CustomBannner()); application.run(args); } } |
3. Disable spring boot banner
If you don’t want the banner it is also possible to disable the banner.
In spring boot, we can disable the banner using configuration file or via a program.
Disabling banner using configuration file is the most flexible and recommended way as is easier and can be reverted easily if required.
3.1 Disable using configuration file:
Spring boot supports multiple ways to configure the application. Like using application.properties, application.yaml file.
3.1.1 Disable banner using application.properties file:
If we add following line to application.properties file the startup banner will be disabled
1 | spring.main.banner-mode=off |
3.1.2 Disable banner using application.yaml file:
If we add following lines to application.yaml file the startup banner will be disabled
1 2 3 | spring: main: banner-mode: "off" |
3.2. Disable banner from application code:
In spring boot code we can configure application using SpringApplication or SpringApplicationBuilder. We can also use java 8 features lambda expression in sring boot application.
3.2.1 Disable banner using the SpringApplication:
1 2 3 | SpringApplication app = new SpringApplication(MyApplication. class ); app.setBannerMode(Banner.Mode.OFF); app.run(args); |
3.2.2 Disable banner using the SpringApplicationBuilder:
1 2 3 | new SpringApplicationBuilder(MyApplication. class ) .bannerMode(Banner.Mode.OFF) .run(args) |
Fast track reading
- Banner is a fancy text message displayed at spring boot application start up
- We can create custom banner our self or generate using online free tools
- Banner can be in a text or image format
- Text format banner is faster to load
- Spring boot banner can have placeholders for dynamic values
- We can also disable spring boot banner
References:
- https://docs.spring.io/spring-boot/docs/current/reference/html/spring-boot-features.html#boot-features-banner
- https://www.javacodemonk.com/custom-banner-in-spring-boot-93a85044
- https://www.concretepage.com/spring-boot/spring-boot-custom-banner-example
Other Topics
- SAM functional interface
- Java 8 use of Optional class
- Java 8 method reference
- Mockito whenThen vs whenAnswer
Published on Java Code Geeks with permission by Stacktraceguru, partner at our JCG program. See the original article here: Spring boot custom banner generation Opinions expressed by Java Code Geeks contributors are their own. |