Object-Relational Mapping (ORM) with NetSuite Data Entities in Java
Object-relational mapping (ORM) techniques make it easier to work with relational data sources and can bridge your logical business model with your physical storage model. Follow this tutorial to integrate connectivity to NetSuite data into a Java-based ORM framework, Hibernate.
You can use Hibernate to map object-oriented domain models to a traditional relational database. The tutorial below shows how to use the CData JDBC Driver for NetSuite to generate an ORM of your NetSuite repository with Hibernate.
Though Eclipse is the IDE of choice for this article, the CData JDBC Driver for NetSuite works in any product that supports the Java Runtime Environment. In the Knowledge Base you will find tutorials to connect to NetSuite data from IntelliJ IDEA and NetBeans.
Install Hibernate
Follow the steps below to install the Hibernate plug-in in Eclipse.
- In Eclipse, navigate to Help -> Install New Software.
- Enter “http://download.jboss.org/jbosstools/neon/stable/updates/” in the Work With box.
- Enter “Hibernate” into the filter box.
- Select Hibernate Tools.

Start A New Project
Follow the steps below to add the driver JARs in a new project.
- Create a new project. Select Java Project as your project type and click Next. Enter a project name and click Finish.
- Right-click the project and click Properties. Click Java Build Path and then open the Libraries tab.
- Click Add External JARs to add the cdata.jdbc.netsuite.jar library, located in the lib subfolder of the installation directory.
Add a Hibernate Configuration File
Follow the steps below to configure connection properties to NetSuite data.
- Right-click on the new project and select New -> Hibernate -> Hibernate Configuration File (cfg.xml).
- Select src as the parent folder and click Next.
- Input the following values:
- Hibernate version:: 5.2
- Database dialect: Derby
- Driver class: cdata.jdbc.netsuite.NetSuiteDriver
- Connection URL: A JDBC URL, starting with jdbc:netsuite: and followed by a semicolon-separated list of connection properties.The User and Password properties, under the Authentication section, must be set to valid NetSuite user credentials. In addition, the AccountId must be set to the Id of a company account that can be used by the specified User. The RoleId can be optionally specified to log in the user with limited permissions.See the “Getting Started” chapter of the help documentation for more information on connecting to NetSuite.Built-in Connection String DesignerFor assistance in constructing the JDBC URL, use the connection string designer built into the NetSuite JDBC Driver. Either double-click the JAR file or execute the jar file from the command-line.
java -jar cdata.jdbc.netsuite.jar
Fill in the connection properties and copy the connection string to the clipboard.

A typical JDBC URL is below:
jdbc:netsuite:Account Id=XABC123456;Password=password;User=user;Role Id=3;Version=2013_1;
Connect Hibernate to NetSuite Data
Follow the steps below to select the configuration you created in the previous step.
- Switch to the Hibernate Configurations perspective: Window -> Open Perspective -> Hibernate.
- Right-click on the Hibernate Configurations panel and click Add Configuration.
- Set the Hibernate version to 5.2.
- Click the Browse button and select the project.
- For the Configuration file field, click Setup -> Use Existing and select the location of the hibernate.cfg.xml file (inside src folder in this demo).
- In the Classpath tab, if there is nothing under User Entries, click Add External JARS and add the driver jar once more. Click OK once the configuration is done.
- Expand the Database node of the newly created Hibernate configurations file.

Reverse Engineer NetSuite Data
Follow the steps below to generate the reveng.xml configuration file. You will specify the tables you want to access as objects.
- Switch back to the Package Explorer.
- Right-click your project, select New -> Hibernate -> Hibernate Reverse Engineering File (reveng.xml). Click Next.
- Select src as the parent folder and click Next.
- In the Console configuration drop-down menu, select the Hibernate configuration file you created above and click Refresh.
- Expand the node and choose the tables you want to reverse engineer. Click Finish when you are done.
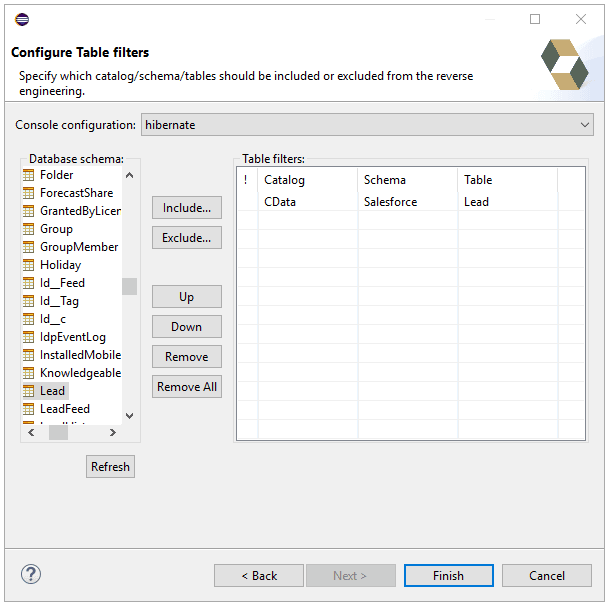
Configure Hibernate to Run
Follow the steps below to generate plain old Java objects (POJO) for the NetSuite tables.
- From the menu bar, click Run -> Hibernate Code Generation -> Hibernate Code Generation Configurations.
- In the Console configuration drop-down menu, select the Hibernate configuration file you created in the previous section. Click Browse by Output directory and select src.
- Enable the Reverse Engineer from JDBC Connection checkbox. Click the Setup button, click Use Existing, and select the location of the hibernate.reveng.xml file (inside src folder in this demo).
- In the Exporters tab, check Domain code (.java) and Hibernate XML Mappings (hbm.xml).
- Click Run.
One or more POJOs are created based on the reverse-engineering setting in the previous step.
Insert Mapping Tags
For each mapping you have generated, you will need to create a mapping tag in hibernate.cfg.xml to point Hibernate to your mapping resource. Open hibernate.cfg.xml and insert the mapping tags as so:
<hibernate-configuration> <session-factory name=""> <property name="hibernate.connection.driver_class"> cdata.netsuite.NetSuiteDriver </property> <property name="hibernate.connection.url"> jdbc:netsuite:Account Id=XABC123456;Password=password;User=user;Role Id=3;Version=2013_1;<!--?xml version="1.0" encoding="UTF-8"?--> </property> <property name="hibernate.dialect"> org.hibernate.dialect.SQLServerDialect </property> <mapping resource="SalesOrder.hbm.xml"></mapping> </session-factory> </hibernate-configuration>
Execute SQL
Using the entity you created from the last step, you can now search and modify NetSuite data:
import java.util.*; import org.hibernate.Session; import org.hibernate.cfg.Configuration; import org.hibernate.query.Query; public class App { public static void main(final String[] args) { Session session = new Configuration().configure().buildSessionFactory().openSession(); String SELECT = "FROM SalesOrder S WHERE Class_Name = :Class_Name"; Query q = session.createQuery(SELECT, SalesOrder.class); q.setParameter("Class_Name","Furniture : Office"); List<SalesOrder> resultList = (List<SalesOrder>) q.list(); for(SalesOrder s: resultList){ System.out.println(s.getCustomerName()); System.out.println(s.getSalesOrderTotal()); } } }
Ready to get started?
Learn more about the CData JDBC Driver for NetSuite or download a free trial: