How to fix an illegal start of expression in Java
Have you ever come across the error illegal start of expression in Java and wondered how to solve it? Let’s go through the post and study how we address the Illegal start of expression Java error.
This is a dynamic error, which means that the compiler finds something that doesn’t follow the rules or syntax of Java programming. Beginners mostly face this bug in Java. Since it is dynamic, it is prompted at compile time i.e., with the javac statement.
This error can be encountered in various scenarios. The following are the most common errors. They are explained on how they can be fixed.
1. Prefixing the Local Variables with Access Modifiers
Variables inside a method or a block are local variables. Local variables have scope within their specific block or method; that is, they cannot be accessed anywhere inside the class except the method in which they are declared. Access modifiers: public, private, and protected are illegal to use inside the method with local variables as its method scope defines their accessibility.
This can be explained with the help of an example:
Class LocalVar { public static void main(String args[]) { int variable_local = 10 } }
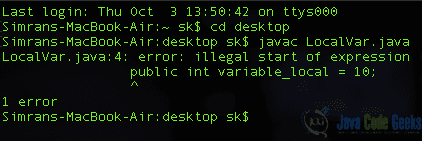
2. Method Inside of Another Method
A method cannot have another method inside its scope. Using a method inside another method would throw the “Illegal start of expression” error. The error would occur irrespective of using an access modifier with the function name.
Below is the demonstration of the code:
Class Method { public static void main (String args[]) { public void calculate() { } } }
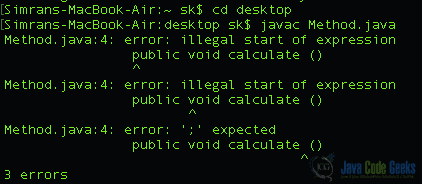
Class Method { public static void main (String args[]) { void calculate() { } } }
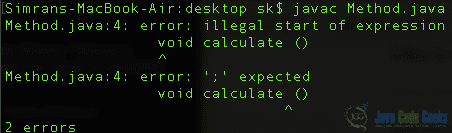
3. Class Inside a Method Must Not Have Modifier
Similarly, a method can contain a class inside its body; this is legal and hence would not give an error at compile time. However, make note classes do not begin with access modifiers, as modifiers cannot be present inside the method.
In the example below, the class Car is defined inside the main method; this method is inside the class Vehicle. Using the public modifier with the class Car would give an error at run time, as modifiers must not be present inside a method.
class Vehicle { public static final void main(String args[]) { public class Car { } } }

4. Missing Curly “{}“ Braces
Skipping the curly braces of any method block can result in having an “illegal start of expression” error. The error will occur because it would be against the syntax or against the rules of Java programming, as every block or class definition must start and end with curly braces. The developer might also need to define another class or method depending on the requirement of the program. Defining another class or method would, in turn, have modifiers as well, which is illegal for the method body.
In the following code, consider the class Addition, the method main adds two numbers and stores them in the variable sum. Later, the result is printed using the method displaySum. An error would be shown on the terminal as the curly brace is missing at the end of the method main.
public class Addition { static int sum; public static void main(String args[]) { int x = 8; int y= 2; sum=0; sum= x + y; { System.out.println("Sum = " + sum); } }
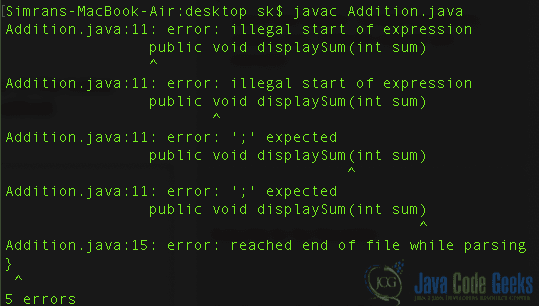
5. String Character Without Double Quotes “”
Initializing string variables without the double quotes is a common mistake made by many who are new to Java, as they tend to forget the double quotes and later get puzzled when the error pops up at the run time. Variables having String data type must be enclosed within the double quotes to avoid the “illegal start of expression” error in their code.
The String variable is a sequence of characters. The characters might not just be alphabets, they can be numbers as well or special characters like @,$,&,*,_,-,+,?, / etc. Therefore, enclose the string variables within the double quotes to avoid getting an error.
Consider the sample code below; the missing quotes around the values of the variable operator generates an error at the run time.
import java.util.*; public class Operator { public static void main(String args[]) { int a = 10; int b = 8; int result =0; Scanner scan = new Scanner(System.in); System.out.println("Enter the operation to be performed"); String operator= scan.nextLine(); if(operator == +) { result = a+b; } else if(operator == -) { result = a-b; } else { System.out.prinln("Invalid Operator"); } System.out.prinln("Result = " + result); }
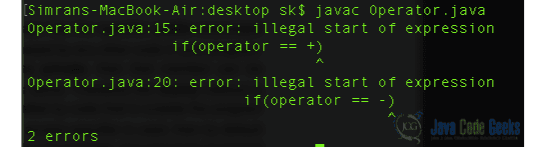
6. Summary
To sum up, the “Illegal start of expression” error occurs when the Java compiler finds something inappropriate with the source code at the time of execution. To debug this error, try looking at the lines preceding the error message for missing brackets, curly braces, or semicolons and check the syntax.
Useful tip: Remember, in some cases, a single syntax error sometimes can cause multiple “Illegal start of expression” errors. Therefore, evaluating the root cause of the error and always recompiling when you fix the bug that means avoiding making multiple changes without compilation at each step.
7. Download the Source Code
You can download the full source code of this article here: How to fix an illegal start of expression in Java
Last updated on Jan. 10th, 2022
So your first example is not the one that shows the error?