Convert Float ArrayList to Primitive Array in Java
In Java, collections such as ArrayList<Float>
are often used to store a dynamic list of objects. However, there might be scenarios where we need to convert this list of Float
objects into a primitive float array (float[]
). This process involves several steps due to the differences between object wrappers (Float
) and primitive types (float
). This article explores multiple methods to achieve this conversion.
1. Using Loop
One straightforward way to convert an ArrayList<Float>
to a float[]
is by using a loop. This method manually iterates through the list and assigns each Float
object to the corresponding position in the primitive float array.
FloatListToArray.java
public class FloatListToArray { public static void main(String[] args) { ArrayList<Float> list = new ArrayList<>(); list.add(1.1f); list.add(2.2f); list.add(3.3f); float[] array = new float[list.size()]; for (int i = 0; i < list.size(); i++) { array[i] = list.get(i); } // Printing the primitive array System.out.println(Arrays.toString(array)); } }
An ArrayList<Float>
named floatList
is created and populated. A primitive float array floatArray
is initialized with the same size as the ArrayList
. A for
loop iterates through each element of the ArrayList
and assigns it to the corresponding index in the primitive array.
Output: The printed elements of the primitive array are:
[1.1, 2.2, 3.3]
2. Using Streams (Java 8 and above)
The Stream API provides a more functional approach to converting collections. This method leverages mapToDouble
and toArray
methods to perform the conversion.
FloatListToStreamArray.java
public class FloatListToStreamArray { public static void main(String[] args) { ArrayList<Float> floatList = new ArrayList<>(); floatList.add(1.1f); floatList.add(2.2f); floatList.add(3.3f); double[] floatArray = floatList.stream() .mapToDouble(Float::doubleValue) .toArray(); // Print the primitive array System.out.println(Arrays.toString(floatArray)); } }
It is worth mentioning that the above code results in a double[]
array rather than a float[]
array due to the absence of a FloatStream
in the Java Stream API.
3. Using Apache Commons Lang
The Apache Commons Lang library provides a utility class ArrayUtils
that simplifies the conversion between different types of arrays. This method requires adding the Apache Commons Lang dependency to your project.
Maven dependency (pom.xml)
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.14.0</version> </dependency>
FloatListToCommonsArray.java
public class FloatListToCommonsArray { public static void main(String[] args) { ArrayList<Float> floatList = new ArrayList<>(); floatList.add(1.1f); floatList.add(2.2f); floatList.add(3.3f); Float[] floatObjectArray = floatList.toArray(Float[]::new); float[] floatArray = ArrayUtils.toPrimitive(floatObjectArray); // Printing the primitive array System.out.println(Arrays.toString(floatArray)); } }
In this approach, An ArrayList<Float>
named floatList
is created and populated. The toArray()
method converts the ArrayList
to an array of Float
objects. We then use the ArrayUtils.toPrimitive()
method from Apache Commons Lang converts the Float
array to a primitive float
array.
Output: The elements of the primitive array are printed as follows:
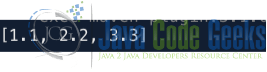
4. Using Eclipse Collections with Collectors2
Eclipse Collections is a collections framework that offers additional utilities and data structures not found in the standard Java Collections framework. Using Eclipse Collections, we can leverage the Collectors2
utility to simplify the conversion of an ArrayList<Float>
to a primitive float
array. This method provides a clean and efficient way to handle the conversion using the Stream API combined with Eclipse Collections.
First, ensure that Eclipse Collections is added to your project. You can include it using Maven:
Maven dependency (pom.xml)
<dependency> <groupId>org.eclipse.collections</groupId> <artifactId>eclipse-collections-api</artifactId> <version>11.1.0</version> </dependency> <dependency> <groupId>org.eclipse.collections</groupId> <artifactId>eclipse-collections</artifactId> <version>11.1.0</version> </dependency>
FloatArrayListToPrimitiveArray.java
import java.util.ArrayList; import org.eclipse.collections.api.factory.primitive.FloatLists; import org.eclipse.collections.api.list.primitive.MutableFloatList; import org.eclipse.collections.impl.collector.Collectors2; public class FloatListToPrimitiveArray { public static void main(String[] args) { // Initialize ArrayList with some values ArrayList floatList = new ArrayList(); floatList.add(1.1f); floatList.add(2.2f); floatList.add(3.3f); // Convert ArrayList to MutableFloatList using Eclipse Collections MutableFloatList floatMutableList = floatList.stream() .collect(Collectors2 .collectFloat(Float::floatValue,FloatLists.mutable::empty)); // Convert MutableFloatList to float array float[] floatArray = floatMutableList.toArray(); // Print the array for (float f : floatArray) { System.out.print(f + " "); } } }
In this code, an ArrayList
named floatList
is created and populated. Using Eclipse Collections, the floatList
is converted into a MutableFloatList
using a stream. The Collectors2.collectFloat
method, combined with FloatLists.mutable::empty
, efficiently collects the Float
values from the list and converts them into a MutableFloatList
which handles primitive floats.
The MutableFloatList
is then converted to a primitive float
array using the toArray
method. The output is:
1.1 2.2 3.3
4.1 Advantages of Using Eclipse Collections
- Efficiency: Eclipse Collections provides optimized data structures and methods for handling primitive types, resulting in better performance.
- Simplicity: Using
Collectors2
simplifies the conversion process by reducing boilerplate code. - Flexibility: Eclipse Collections offers a wide range of utilities and data structures that enhance Java’s standard collection framework.
5. Conclusion
In this article, we explored several methods to convert a Float
ArrayList
to a primitive float
array in Java. We examined simple approaches such as using a for loop as well as more advanced techniques involving streams. Additionally, we discussed using Eclipse Collections with the Collectors2
utility for an efficient and streamlined conversion process. Each method offers its advantages: the for-loop method provides simplicity and control, streams offer a concise and functional approach, and Eclipse Collections enhance both efficiency and simplicity.
6. Download the Source Code
This was an article on how to convert a Float ArrayList to a primitive Array using Java.
You can download the full source code of this example here: Java convert float arraylist primitive array