10 Java Libraries to Supercharge Your Code
Hi, fellow Java enthusiasts! Whether you’re a coding pro or just getting the hang of things, there’s a cool trick up your sleeve – Java libraries. These little wonders can turn your code into a powerhouse, doing tricky stuff for you and making your job way easier. No need to start from scratch; these libraries handle complex tasks and add extra features to your code effortlessly. In this article, we’ll check out ten of these magic Java libraries that can make your coding journey smoother and your software seriously impressive. Let’s dive in and level up your coding game! 🚀
Check also here for 10 Popular Libraries for Java Unit and Integration Testing
Below we will present 10 Java Libraries to make your code awesome!
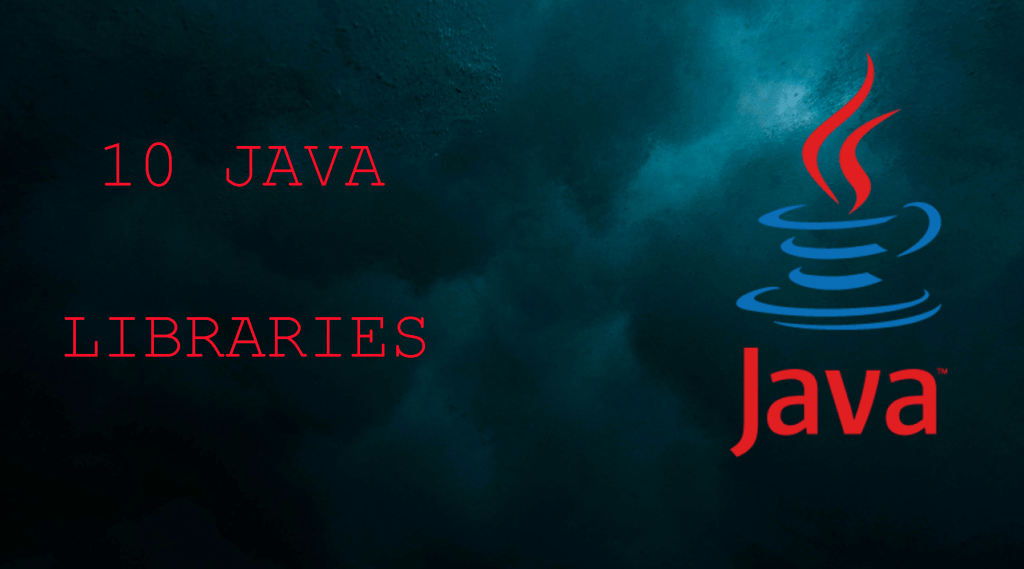
1. Guava (Google Core Libraries for Java):
Guava, developed by Google, is a set of core libraries for Java that provides additional functionality and utilities to complement the standard Java libraries. It’s designed to make common programming tasks simpler and more efficient, offering features that enhance code readability and maintainability.
One of Guava’s standout features is its collection utilities. It provides a rich set of collection types and utilities for working with them. The Immutable
collections, such as ImmutableList
and ImmutableMap
, are particularly notable. These collections are designed to be unmodifiable, thread-safe, and, importantly, offer better performance compared to their mutable counterparts in certain scenarios. For example, an ImmutableList
is ideal when you need to create a list that should not be modified after creation.
Guava also introduces functional programming concepts to Java, making code more expressive. It includes utilities for working with functional interfaces, such as Function
and Predicate
, allowing developers to write more concise and readable code.
Furthermore, Guava’s caching utilities are widely appreciated. The CacheBuilder
provides a fluent API for creating in-memory caches with features like expiration, automatic loading, and eviction policies. This is particularly useful in scenarios where caching can significantly improve application performance.
Considerations:
While Guava is a powerful library with numerous benefits, developers need to be mindful of its extensive functionality. For smaller projects or those not requiring the full breadth of features, the inclusion of Guava might introduce unnecessary complexity. It’s essential to weigh the benefits of using specific Guava features against the potential learning curve and additional dependencies.
Guava’s extensive documentation, along with Google’s active maintenance and community support, contributes to its accessibility. Developers often find the library’s functionality valuable, especially when dealing with collections, caching, and functional programming in Java.
Code Snippet:
Let’s look at a simple code snippet using Guava to create an immutable list:
import com.google.common.collect.ImmutableList; public class GuavaExample { public static void main(String[] args) { // Creating an immutable list using Guava ImmutableList<String> immutableList = ImmutableList.of("Java", "Guava", "Library"); // Printing the elements of the immutable list for (String element : immutableList) { System.out.println(element); } } }
In this example, ImmutableList.of
from Guava is used to create an immutable list. The list cannot be modified after creation, ensuring thread safety and immutability, which can be beneficial in various scenarios.
2. Apache Commons
Apache Commons is a collection of reusable Java components that provides a wide range of utilities and functionality to simplify common programming tasks. It serves as an extensive toolbox for Java developers, offering solutions to various challenges without the need to reinvent the wheel.
One of the notable features of Apache Commons is its versatility. The library covers diverse areas, including but not limited to configuration, file operations, mathematical operations, and text manipulation. Each component within Apache Commons is designed to be standalone, allowing developers to pick and choose the modules that best suit their needs.
For instance, the StringUtils
class provides a plethora of string manipulation methods, offering solutions for tasks like checking if a string is empty or null, capitalizing words, and performing various other string operations. This streamlines common string-handling tasks, making code more readable and concise.
Apache Commons’ Math
package introduces mathematical utilities that go beyond what’s available in the standard Java libraries. It includes methods for statistical operations, random number generation, and numerical analysis. This can be particularly helpful when dealing with complex mathematical computations in applications.
Considerations:
While Apache Commons is a valuable resource for Java developers, its extensive set of functionalities might be considered overkill for smaller projects with simpler requirements. Developers need to be aware of the available features and choose components judiciously based on the specific needs of their projects.
Additionally, Apache Commons’ modular design allows for flexibility, but developers should be cautious about introducing unnecessary dependencies. Each component should be evaluated for its relevance to the project to avoid potential bloat.
Code Snippet:
Let’s take a look at a simple code snippet using Apache Commons to check if a string is empty or null:
import org.apache.commons.lang3.StringUtils; public class ApacheCommonsExample { public static void main(String[] args) { // Checking if a string is empty or null using Apache Commons String text = "Hello, Apache Commons!"; if (StringUtils.isNotEmpty(text)) { System.out.println("The string is not empty or null."); } else { System.out.println("The string is either empty or null."); } } }
In this example, StringUtils.isNotEmpty
from Apache Commons is used to check if a string is not empty or null, providing a convenient and readable way to handle such scenarios.
3. Lombok
Lombok is a Java library that aims to reduce boilerplate code by introducing annotations to automatically generate common code structures during compilation. It simplifies the development process by automating the creation of methods like getters, setters, equals, hashCode, and toString, among others. Lombok’s goal is to enhance code readability and maintainability while reducing the amount of repetitive and verbose code that developers need to write.
One of Lombok’s key features is the @Data
annotation, which combines the functionality of several other annotations, such as @Getter
, @Setter
, @ToString
, @EqualsAndHashCode
, and @RequiredArgsConstructor
. By applying @Data
to a class, developers can automatically generate methods like getters and setters for all fields, a meaningful toString
method, and appropriate implementations for equals
and hashCode
. This results in more concise and cleaner code, especially for classes that primarily serve as data containers.
Lombok also introduces annotations like @Builder
for simplifying the creation of builder patterns, @Slf4j
for straightforward integration with the Simple Logging Facade for Java (Slf4j), and @NoArgsConstructor
and @AllArgsConstructor
for generating constructors with no arguments or all arguments, respectively.
Considerations:
While Lombok significantly reduces boilerplate code, developers should be aware that its usage involves a form of code generation. This might lead to less readable decompiled code, as the generated methods are not present in the actual source files. Developers need to ensure that their build tools and IDEs support Lombok properly to benefit from its features during both development and debugging.
Additionally, as with any library or tool that abstracts away code, it’s essential to consider the learning curve for team members who might not be familiar with Lombok. Clear documentation and communication within the development team can mitigate potential challenges.
import lombok.Data; @Data public class Person { private String name; private int age; }
In this example, the @Data
annotation automatically generates getters, setters, toString
, equals
, and hashCode
methods for the Person
class. This concise code allows developers to focus on the core logic of the class without the need to write boilerplate code for common methods.
4. Jackson
Jackson is a popular Java library for processing JSON data. Its primary goal is to provide a fast, efficient, and flexible way to serialize Java objects into JSON format and deserialize JSON content into Java objects. Jackson is widely used in the Java ecosystem, and its versatility makes it suitable for various scenarios, from simple data binding to more complex tasks like tree traversal and streaming.
One of Jackson’s key features is its simplicity in mapping Java objects to JSON and vice versa. It supports both annotation-based and programmatic configuration, giving developers the flexibility to choose the approach that best fits their needs. Annotations like @JsonProperty
allow fine-grained control over the mapping process, enabling developers to customize field names in the JSON representation.
Jackson also excels in supporting different data formats and handling polymorphic types. It provides modules for processing data in formats like XML, YAML, and Protocol Buffers. Additionally, Jackson’s support for polymorphism, including handling abstract classes and interfaces, makes it a powerful choice for scenarios where object hierarchies need to be accurately represented in JSON.
Considerations:
While Jackson offers comprehensive features, developers should be mindful of its configuration options to avoid unexpected behaviors. For instance, Jackson provides different modules and configuration settings for handling date and time formats, and developers need to ensure that the chosen configuration aligns with their application’s requirements.
Additionally, Jackson allows customization through various extension points, such as custom serializers and deserializers. While this flexibility is beneficial, it requires a good understanding of Jackson’s internals, and developers should exercise caution to avoid unnecessary complexity.
Code Snippet:
Let’s look at a simple code snippet using Jackson to serialize a Java object to JSON:
import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonExample { public static void main(String[] args) throws Exception { // Creating a simple Java object Person person = new Person("John Doe", 30); // Serializing the Java object to JSON ObjectMapper objectMapper = new ObjectMapper(); String json = objectMapper.writeValueAsString(person); // Printing the JSON representation System.out.println(json); } }
In this example, Jackson’s ObjectMapper
is used to serialize a Person
object to a JSON string. This straightforward code demonstrates Jackson’s ease of use for basic JSON serialization tasks.
5. Slf4j (Simple Logging Facade for Java)
Slf4j is a logging framework for Java designed to provide a simple facade or abstraction for various logging frameworks. Its primary purpose is to offer a standardized logging API that allows developers to code against a common interface while being able to switch or adapt to different logging implementations at runtime. Slf4j is not an implementation itself; instead, it serves as a bridge between the application and an underlying logging framework.
One of Slf4j’s key features is its simplicity. It introduces a set of logging methods, such as debug
, info
, warn
, and error
, making it easy for developers to incorporate logging statements into their code. By using Slf4j, developers can avoid direct dependencies on specific logging frameworks, making the code more modular and adaptable.
Slf4j’s design also facilitates efficient logging by allowing the deferred evaluation of log statements. This means that log messages are only constructed and formatted when necessary, reducing the performance impact of logging in scenarios where the logging level is not enabled.
Considerations:
One of the primary considerations when using Slf4j is the need to include an actual logging implementation at runtime. Slf4j serves as the facade, and developers need to choose a compatible logging framework, such as Logback or Log4j, and include its implementation in the project. This modular design allows for flexibility but requires additional configuration.
Another consideration is the careful use of placeholders in log messages. Slf4j supports placeholders in log messages, enabling deferred evaluation. However, improper use of placeholders can lead to unexpected behavior, and developers should be aware of the best practices for constructing log messages.
Code Snippet:
Let’s look at a simple code snippet using Slf4j for logging:
import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class Slf4jExample { // Creating a logger using Slf4j private static final Logger logger = LoggerFactory.getLogger(Slf4jExample.class); public static void main(String[] args) { // Logging statements using Slf4j logger.debug("Debug message"); logger.info("Info message"); logger.warn("Warning message"); logger.error("Error message"); } }
In this example, Slf4j’s Logger
interface is used to log messages at different levels. The actual logging implementation (e.g., Logback) will determine how these messages are handled at runtime.
6. JUnit
JUnit is a widely used testing framework for Java that provides a standardized way to write and execute tests. Its primary purpose is to simplify the process of unit testing—testing individual units or components of a software application in isolation. JUnit follows the principles of test-driven development (TDD), encouraging developers to write tests before implementing the corresponding code.
One of JUnit’s key features is its annotation-based approach to test creation. Developers use annotations such as @Test
to define test methods, making it clear which methods are part of the testing suite. JUnit also supports annotations like @Before
and @After
to denote setup and teardown methods, executed before and after each test method, respectively.
JUnit provides a rich set of assertion methods to validate expected outcomes, making it straightforward for developers to express test conditions. Assertions include methods like assertEquals
, assertTrue
, and assertNotNull
. When an assertion fails, JUnit provides informative messages, aiding developers in diagnosing and fixing issues quickly.
JUnit’s integration with various development environments and build tools, such as Eclipse and Maven, streamlines the testing process. The ability to run tests from the command line or as part of a continuous integration pipeline enhances the overall testing workflow.
Considerations:
While JUnit is a powerful testing framework, developers should be mindful of writing effective and meaningful test cases. It’s essential to focus on testing specific behaviors and edge cases to ensure comprehensive coverage. Additionally, maintaining a balance between the number of tests and the speed of test execution is crucial for efficient development workflows.
JUnit’s simplicity can sometimes lead to less emphasis on more complex testing scenarios, such as integration and system testing. In larger projects, combining JUnit with other testing frameworks or tools may be necessary to address diverse testing needs.
Code Snippet:
Let’s look at a simple code snippet using JUnit to test a basic arithmetic operation:
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; public class MathOperationsTest { @Test public void testAddition() { int result = MathOperations.add(3, 5); assertEquals(8, result, "Adding 3 and 5 should equal 8"); } }
In this example, the @Test
annotation marks the testAddition
method as a test case. The assertEquals
assertion checks if the result of adding 3 and 5 matches the expected value of 8. JUnit provides clear feedback in case of test failures, aiding developers in diagnosing issues.
7. Joda-Time
Joda-Time is a widely used date and time library for Java, designed to address shortcomings in the legacy java.util.Date
and java.util.Calendar
classes. It provides a more comprehensive and developer-friendly API for handling date and time operations. Joda-Time is known for its ease of use, immutability, and support for various calendar systems.
One of the key features of Joda-Time is its DateTime
class, which represents an instant in time with a time zone. This class, along with others like LocalDate
and LocalTime
, simplifies common date and time operations. Joda-Time introduces the concept of immutability, meaning that once a DateTime
object is created, its state cannot be changed. Immutability enhances thread safety and reduces the risk of unintended side effects.
Joda-Time provides a rich set of methods for manipulating and formatting dates and times. Operations such as addition, subtraction, and comparison are straightforward, making it easier for developers to express date-related logic in a clear and concise manner. The library also includes utilities for parsing and formatting date-time strings according to various patterns.
Another notable feature is Joda-Time’s support for different calendar systems, including the Gregorian, ISO, and Buddhist calendars. This flexibility is particularly valuable when dealing with internationalization or applications that need to handle dates in non-Gregorian calendars.
Considerations:
While Joda-Time is a powerful library, developers should be aware that Java has introduced the java.time
package starting from Java 8, providing a modern date and time API. For projects using Java 8 or later, migrating to the java.time
API might be a consideration, as it is part of the Java standard library.
Additionally, when working with Joda-Time, developers need to ensure proper handling of time zones, especially when dealing with applications that operate in different geographical regions. Understanding the nuances of time zone usage is crucial for accurate date and time calculations.
Code Snippet:
Let’s look at a simple code snippet using Joda-Time to calculate the difference in days between two dates:
import org.joda.time.DateTime; import org.joda.time.Days; public class DateDifferenceExample { public static void main(String[] args) { // Creating two DateTime objects representing different dates DateTime startDate = new DateTime(2022, 1, 1, 0, 0); DateTime endDate = new DateTime(2022, 1, 10, 0, 0); // Calculating the difference in days int daysDifference = Days.daysBetween(startDate, endDate).getDays(); // Printing the result System.out.println("The difference in days is: " + daysDifference); } }
In this example, Joda-Time’s DateTime
and Days
classes are used to calculate the difference in days between two dates. The result is then printed to the console.
8. Spring Framework
The Spring Framework is a comprehensive and widely adopted framework for building Java-based enterprise applications. It provides a holistic approach to application development, offering solutions for various aspects such as dependency injection, aspect-oriented programming, data access, transaction management, and more. One of the key goals of the Spring Framework is to simplify the development of complex, scalable, and maintainable Java applications.
One of the standout features of the Spring Framework is its support for Inversion of Control (IoC) and Dependency Injection (DI). IoC shifts the responsibility of managing object lifecycles and dependencies from the application code to the framework, promoting loosely coupled and modular designs. The DI mechanism allows developers to declare and inject dependencies through configuration, making the application more flexible and testable.
Spring’s AOP (Aspect-Oriented Programming) support enables developers to modularize cross-cutting concerns such as logging, security, and transaction management. This promotes cleaner code organization and reusability by separating concerns that would otherwise be scattered throughout the application.
The Spring ecosystem includes various projects and modules catering to different needs. For instance, Spring MVC provides a robust framework for building web applications, while Spring Data simplifies data access through consistent abstractions. Spring Boot, an opinionated extension of the framework, streamlines the process of building production-ready applications by providing defaults and conventions.
The framework’s integration with other technologies, such as Hibernate for ORM (Object-Relational Mapping), and its support for declarative transaction management contribute to its popularity in enterprise application development.
Considerations:
While the Spring Framework offers extensive capabilities, developers should carefully choose the modules that align with their application’s requirements. Overuse of certain features or modules may introduce unnecessary complexity.
Understanding the concepts of IoC, DI, and AOP is essential for effective utilization of the Spring Framework. Developers new to Spring should invest time in learning these core principles to maximize the benefits of the framework.
Code Snippet:
Let’s look at a simplified code snippet using Spring’s dependency injection:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class GreetingService { private final MessageProvider messageProvider; @Autowired public GreetingService(MessageProvider messageProvider) { this.messageProvider = messageProvider; } public String greet() { return "Hello, " + messageProvider.getMessage(); } }
In this example, the GreetingService
class relies on dependency injection to obtain a MessageProvider
. The @Autowired
annotation indicates that Spring should automatically inject the required dependency when creating an instance of GreetingService
. This promotes a decoupled and modular design.
9. Gson
Gson is a popular Java library developed by Google for serialization and deserialization of Java objects to and from JSON format. Its primary goal is to provide a simple and efficient way to work with JSON data in Java applications. Gson seamlessly converts Java objects to their JSON representation and vice versa, making it a valuable tool for communication between Java applications and web services.
One of the key features of Gson is its simplicity in usage. Developers can convert Java objects to JSON and JSON to Java objects with minimal code, often requiring just a single line of code for common scenarios. Gson’s design focuses on ease of integration, and it works well with complex object hierarchies, collections, and custom data types.
Gson supports customization through annotations, allowing developers to control the serialization and deserialization process. Annotations like @SerializedName
enable mapping Java field names to specific JSON property names. This flexibility is particularly useful when working with APIs that follow a specific JSON structure.
Gson also provides a powerful streaming API, allowing developers to efficiently process large JSON documents without the need to load the entire content into memory. This is beneficial for scenarios where memory efficiency is a concern.
Considerations:
While Gson is a versatile library, developers should be aware of potential security risks when deserializing untrusted JSON data. Maliciously crafted JSON payloads may lead to security vulnerabilities, such as code execution. It’s crucial to validate and sanitize input data before deserialization.
Gson may not be the most suitable choice for scenarios requiring high-performance JSON processing, as it prioritizes ease of use over absolute speed. In situations where ultra-fast serialization and deserialization are critical, alternative libraries optimized for performance may be considered.
Code Snippet:
Let’s look at a simple code snippet using Gson to serialize a Java object to JSON:
import com.google.gson.Gson; public class Person { private String name; private int age; // Getter and setter methods... public static void main(String[] args) { // Creating a Person object Person person = new Person(); person.setName("John Doe"); person.setAge(30); // Serializing the Person object to JSON Gson gson = new Gson(); String json = gson.toJson(person); // Printing the JSON representation System.out.println(json); } }
In this example, Gson’s toJson
method is used to serialize a Person
object to a JSON string. The resulting JSON representation can be easily sent over a network or stored in a file.
10. RxJava
RxJava is a reactive programming library for Java that extends the principles of the ReactiveX (Reactive Extensions) pattern to the Java programming language. Reactive programming is centered around the idea of asynchronous and event-driven data streams, providing a powerful paradigm for handling and composing asynchronous operations.
One of the key features of RxJava is the concept of Observables and Observers. An Observable represents a stream of data that can be observed, and an Observer subscribes to and reacts to events emitted by the Observable. This allows developers to express complex asynchronous operations in a concise and declarative manner.
RxJava provides a rich set of operators that enable developers to transform, filter, and combine data streams. These operators facilitate the manipulation and composition of asynchronous events, making it easier to express complex workflows. Operations such as map
, filter
, and merge
become powerful tools in the RxJava toolkit.
Concurrency and parallelism are inherent in RxJava. The library allows developers to work with schedulers to control the execution context of Observables and manage concurrency. This is particularly beneficial when dealing with complex applications where multiple asynchronous tasks need to be coordinated.
RxJava’s error-handling mechanisms, such as the onError
callback, provide a structured approach to deal with errors in asynchronous workflows. This helps in creating robust and fault-tolerant applications.
Considerations:
While RxJava offers powerful abstractions for handling asynchronous programming, there is a learning curve associated with reactive programming concepts. Developers transitioning to RxJava may need time to familiarize themselves with Observables, Observers, and the wide array of operators available.
RxJava might be overkill for simpler applications where the benefits of reactive programming are not fully realized. It is well-suited for scenarios with complex and asynchronous requirements, such as UI interactions, network requests, and event-driven architectures.
Code Snippet:
Let’s look at a simple code snippet using RxJava to create and observe a stream of integers:
import io.reactivex.Observable; import io.reactivex.Observer; import io.reactivex.disposables.Disposable; public class RxJavaExample { public static void main(String[] args) { // Creating an Observable emitting integers from 1 to 5 Observable<Integer> observable = Observable.just(1, 2, 3, 4, 5); // Creating an Observer to subscribe to the Observable Observer<Integer> observer = new Observer<Integer>() { @Override public void onSubscribe(Disposable d) { // Not used in this example } @Override public void onNext(Integer value) { System.out.println("Received: " + value); } @Override public void onError(Throwable e) { System.err.println("Error: " + e.getMessage()); } @Override public void onComplete() { System.out.println("Complete"); } }; // Subscribing the Observer to the Observable observable.subscribe(observer); } }
In this example, an Observable is created to emit integers from 1 to 5, and an Observer is used to subscribe to and react to the emitted values. The subscribe
method initiates the observation, and the Observer’s methods handle the received values, errors, and completion signal.
Wrapping Up
In the dynamic landscape of Java development, these libraries play the role of magic wands, making coding experiences more delightful and efficient. Gson turns Java objects into JSON effortlessly, Spring simplifies complex enterprise application development, and RxJava revolutionizes asynchronous programming with its reactive approach.
Each library brings unique strengths to the table, but it’s essential for developers to choose wisely based on their project requirements. Gson is excellent for JSON manipulation, Spring empowers enterprise-level applications, and RxJava transforms the way we handle asynchronous tasks.
As you embark on your Java coding adventures, consider these libraries as invaluable companions, ready to add a touch of magic to your development journey. Happy coding!