Introduction To Garbage Collection
A Garbage Collector (GC) is a program or mechanism that automatically frees up memory space in a computer’s memory (RAM) that is no longer being used by the program. It is a type of memory management system that helps prevent memory leaks and memory fragmentation, which can cause a program to crash or become unstable.
When a program creates an object or allocates memory, the Garbage Collector keeps track of its usage and determines whether it is still in use by the program. If the GC finds that the object or memory is no longer being used, it marks it as garbage and reclaims the memory.
There are different types of Garbage Collectors, including reference counting, tracing, and generational. Each type has its own approach to detecting and collecting garbage, and the best choice depends on the application’s requirements.
GC is commonly used in programming languages such as Java, Python, and C#. While GC can provide significant benefits in terms of memory management and program stability, it can also impact performance and introduce latency. Therefore, it is essential to consider the trade-offs when choosing the right GC algorithm for an application.
In this article we will present a general idea of what is a garbage collector, tips for organization and we will also persent some of their basics algorithms thoughout the years.
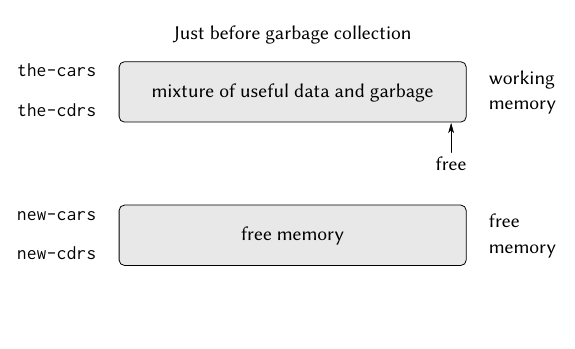
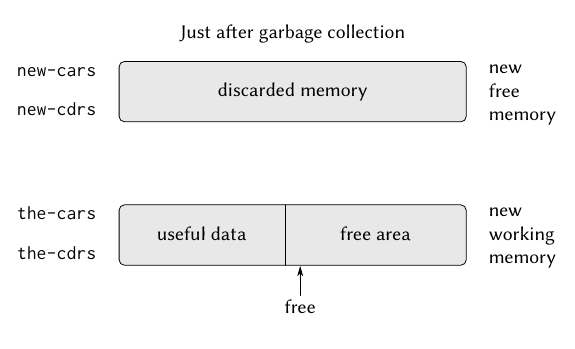
1. Pros and Cons about Garbage Collector
Garbage collection has both advantages and disadvantages, which are outlined below:
Pros:
- Automatic memory management: Garbage collection eliminates the need for manual memory management, which can be error-prone and time-consuming, especially in large or complex programs.
- Memory leak prevention: Garbage collection helps prevent memory leaks by automatically freeing up memory that is no longer being used by a program.
- Improved program stability: Garbage collection reduces the likelihood of program crashes due to memory exhaustion or other memory-related issues.
- Simplified programming: Garbage collection allows programmers to focus on writing application logic without having to worry about memory management, leading to simpler and more readable code.
Cons:
- Performance overhead: Garbage collection can have a performance overhead due to the need to periodically scan and free up memory. This can impact program response time and throughput, especially in real-time systems or systems with limited memory resources.
- Pause times: Garbage collection may cause pause times or “stop the world” events, where the program must pause execution while garbage collection is performed. This can impact the responsiveness of the program and may be unacceptable in some applications.
- Memory fragmentation: Garbage collection can lead to memory fragmentation, where free memory is broken up into smaller and smaller pieces, making it harder to find contiguous blocks of memory for allocation.
- Resource usage: Garbage collection may consume significant system resources, such as CPU time, memory, and I/O bandwidth. This can be a problem in resource-constrained environments or in applications that require high performance.
In summary, garbage collection is a powerful tool for managing memory in modern programming languages. While it offers many advantages, such as automatic memory management and memory leak prevention, it also has some drawbacks, such as performance overhead, pause times, and memory fragmentation, that must be carefully considered when designing and implementing programs.
2. Organization of Garbage Collector
The organization of a Garbage Collector (GC) can vary depending on the type of GC and the programming language or platform being used. However, there are some common components that are typically present in a GC implementation:
- Heap
The heap is a region of memory in computer systems where dynamic memory allocation occurs. It is used by programming languages such as Java, Python, and C++ to allocate memory dynamically during runtime. The heap is separate from the stack, which is another region of memory used to store local variables and function calls.
In Java, the heap is where objects are allocated using the “new” keyword. When an object is created, memory is allocated on the heap to store the object’s data. The amount of memory allocated depends on the object’s size and the JVM’s memory allocation policies.
The heap is managed by the Garbage Collector (GC), which automatically frees up memory that is no longer needed by the application. The GC uses various algorithms to detect and remove objects that are no longer in use by the application, allowing the heap to be reused for new object allocations.
The heap is organized into generations, typically three: young, old, and permanent. The young generation is where new objects are allocated. The GC uses a generational approach to manage the heap, which means that it treats objects differently based on their age. Young objects are garbage-collected more frequently, while older objects are moved to the old generation, where they are garbage-collected less frequently.
The permanent generation (PermGen) is a region of memory that is used to store metadata about classes and methods. In Java 8 and later, the PermGen was replaced by the Metaspace, which is a separate region of memory that can expand and contract dynamically.
The heap size can be configured using JVM options such as -Xms and -Xmx, which specify the minimum and maximum heap sizes, respectively. It is important to choose the right heap size based on the application’s requirements to avoid OutOfMemory errors or excessive GC activity.
In summary, the heap is a crucial part of memory management in Java and other programming languages. It provides a region of memory for dynamic memory allocation and is managed by the GC, which automatically frees up memory that is no longer needed by the application. Understanding how the heap works and how to tune it is essential for building efficient and stable applications.
- Root Set
In computer science, a root set is a set of pointers that serve as starting points for the garbage collector to find all live objects in memory. In other words, the root set contains all the objects that are directly or indirectly reachable from the program’s execution stack or from registers.
During garbage collection, the garbage collector starts with the root set and traces all objects that are reachable from the root set. Any objects that are not reachable from the root set are considered garbage and can be safely reclaimed by the garbage collector.
In Java, the root set typically includes the following:
- Local variables in the program’s execution stack
- Static fields in the program’s classes
- Objects referenced by the current thread’s call stack
- JNI (Java Native Interface) global references
- System classloader and bootstrap classloader
- JVM internal data structures
The root set is critical for the garbage collector’s ability to correctly identify all live objects in memory. If any live objects are not reachable from the root set, they will not be traced and will be incorrectly treated as garbage.
One important consideration for developers is to ensure that any objects that are intentionally kept alive (such as caches or global variables) are included in the root set. This can be accomplished by holding a reference to these objects in one of the root set components (such as a static field or JNI global reference).
- Mark Phase
The Mark phase is a critical step in the mark-and-sweep algorithm used by many garbage collectors to reclaim memory that is no longer being used by the application. During the Mark phase, the garbage collector traverses the heap to identify all live objects and mark them as such.
The Mark phase typically begins with the root set, which includes all objects that are directly or indirectly reachable from the program’s execution stack or from registers. The garbage collector then traces all objects that are reachable from the root set, marking them as live.
During the Mark phase, each object is inspected and marked using a flag or other mechanism that indicates that it is still in use by the application. This marking process is usually performed recursively, as objects that are marked as live may reference other objects that also need to be marked.
Once all live objects have been marked, the garbage collector moves on to the next phase of the algorithm, typically the Sweep phase. During the Sweep phase, the garbage collector frees all memory that is not marked as live.
One of the key challenges of the Mark phase is ensuring that all live objects are correctly identified and marked. If any live objects are not marked, they will be incorrectly treated as garbage and may be prematurely freed, leading to errors or crashes in the application. Conversely, if any objects that are no longer in use are mistakenly marked as live, memory will not be freed, leading to excessive memory usage and reduced application performance.
To mitigate these risks, many garbage collectors use conservative scanning techniques that assume any memory location that contains a pointer may be a valid reference to an object. While conservative scanning can be less accurate than other techniques, it can help ensure that all live objects are correctly identified and marked during the Mark phase.
- Sweep Phase
The sweep phase is a key part of the garbage collection process in memory management. The garbage collector is responsible for automatically freeing up memory that is no longer being used by the program.
During the sweep phase, the garbage collector scans the heap (the area of memory used for dynamic memory allocation) to identify any memory blocks that are no longer being used by the program. These blocks are then marked as free and added to the free list, which is a list of available memory blocks that can be allocated for future use.
The sweep phase is typically preceded by the mark phase, which involves marking all the live objects in the heap. The mark phase identifies which memory blocks are still in use and which are not. The sweep phase then identifies the memory blocks that are no longer in use and adds them to the free list.
Once the sweep phase is complete, the garbage collector can start allocating memory for new objects. The free list is used to keep track of which memory blocks are available for allocation.
- Compaction
Compaction is an optional process that is sometimes used in garbage collection to improve memory usage and reduce fragmentation. It is typically used in systems where memory is scarce or expensive, or where performance is critical.
During the compaction process, the garbage collector moves live objects in the heap closer together, freeing up contiguous blocks of memory. This reduces fragmentation and helps to ensure that memory is being used more efficiently.
The compaction process involves scanning the heap to identify live objects and moving them to new memory locations. Any unused memory is then consolidated and added to the free list for future use.
There are two main types of compaction: copying compaction and mark-and-sweep compaction. Copying compaction involves copying all live objects from one part of the heap to another, leaving unused memory in one contiguous block. Mark-and-sweep compaction involves identifying and relocating live objects, as well as cleaning up unused memory blocks in the process.
While compaction can be an effective way to reduce fragmentation and improve memory usage, it can also be resource-intensive and may impact performance. As a result, it is typically used only in situations where its benefits outweigh the potential drawbacks.
- Finalization
Finalization is a process in garbage collection that allows objects to perform cleanup operations before they are destroyed. It is typically used in object-oriented programming languages where objects may hold resources that need to be explicitly released, such as file handles, database connections, or network sockets.
When an object is no longer in use and is ready to be destroyed, the garbage collector first checks to see if the object has a finalizer. If it does, the garbage collector schedules the finalizer to be run at a later time, typically after the object has been marked as eligible for garbage collection.
During finalization, the object’s finalizer method is called, allowing it to perform any necessary cleanup operations. This might include releasing resources, closing files or connections, or performing any other actions that need to be taken before the object is destroyed.
It’s important to note that finalization can be resource-intensive and may impact performance, so it should be used sparingly and only when necessary. Additionally, the order in which finalizers are run is not guaranteed, which can sometimes lead to unexpected behavior or resource leaks.
As an alternative to finalization, many modern programming languages provide other mechanisms for resource management, such as automatic reference counting or garbage collectors that support deterministic destruction. These mechanisms can help to ensure that resources are released in a timely and predictable manner, without the need for finalization.
The above components are common to most GC implementations, but there can be variations and optimizations based on the programming language, platform, and GC algorithm used.
3. Important Things about Garbage Collector
Here are some important things to know about garbage collectors:
- Garbage collection is a process of automatic memory management that frees up memory that is no longer being used by a program.
- Garbage collection can help prevent memory leaks, which occur when memory is allocated but not freed, leading to memory exhaustion and program crashes.
- There are different types of garbage collection algorithms, such as mark-and-sweep, stop-and-copy, and generational garbage collection. Each algorithm has its own strengths and weaknesses and may be more suitable for different types of programs and workloads.
- Garbage collection can impact program performance and memory usage, especially in real-time systems or systems with limited memory resources. Careful tuning of the garbage collector settings may be necessary to achieve optimal performance.
- Some programming languages provide manual memory management, where the programmer is responsible for allocating and freeing memory. However, this can be error-prone and time-consuming, especially in large or complex programs.
- Garbage collection is not a silver bullet and does not eliminate the need for good programming practices, such as minimizing memory usage, avoiding circular references, and properly managing resources such as files, database connections, and network sockets.
Overall, garbage collection is an important and powerful tool for managing memory in modern programming languages. However, it is important to understand how garbage collection works and how it can impact program performance and memory usage, and to use it in conjunction with good programming practices to create robust and efficient programs.
4. Algorithms used by JDK 8 until JDK 12
Java Development Kit (JDK) 8 to 12 comes with different garbage collection algorithms that can be used depending on the application requirements. The default GC algorithm changed from JDK 8 to JDK 12. Here is a summary of the algorithms used by JDK 8 to JDK 12:
JDK 8:
- Serial GC: This is the default GC algorithm in JDK 8. It is a simple, single-threaded, stop-the-world algorithm that uses a mark-sweep-compact approach. It is suitable for small applications or applications with a small amount of data.
- Parallel GC: This is a multi-threaded GC algorithm that uses a similar approach to the Serial GC but with multiple threads to perform marking, sweeping, and compacting. It is suitable for applications that require higher throughput and can benefit from parallelism.
- CMS GC: The Concurrent Mark Sweep (CMS) GC is a concurrent GC algorithm that performs garbage collection concurrently with the application’s execution. It uses a mark-sweep approach and is suitable for applications that require low latency and can tolerate some fragmentation.
JDK 9:
- G1 GC: Garbage First (G1) is a parallel, concurrent, and compacting GC algorithm that is designed to provide high throughput and low latency. It divides the heap into regions and performs GC on each region separately. It is suitable for large applications with a large amount of data and can handle applications with mixed types of objects.
JDK 10:
- G1 GC (default): Starting from JDK 10, the G1 GC became the default GC algorithm.
JDK 11:
- Epsilon GC: This is a no-op GC algorithm that can be used for testing or performance benchmarking. It does not perform any garbage collection and allows applications to run without any memory management overhead.
JDK 12:
- Shenandoah GC: This is a concurrent, low-pause-time GC algorithm that uses a unique approach called “concurrent evacuation.” It is designed to provide low latency for large heap sizes and is suitable for applications that require predictable and consistent response times.
- G1 GC (default): Starting from JDK 12, the default GC algorithm is still G1 GC.
It is important to note that JDK also offers different GC tuning options that can affect the GC algorithm’s behavior and performance.
5. Conclusion
Garbage collection is an important feature of modern programming languages that helps automate memory management, prevent memory leaks, and improve program stability. However, it also has some drawbacks, such as performance overhead, pause times, and memory fragmentation, which must be carefully managed and optimized for different workloads and applications.
Overall, the benefits of garbage collection outweigh its drawbacks, as it simplifies programming and reduces the risk of memory-related issues, such as crashes and leaks. Garbage collection is constantly evolving, with new algorithms and techniques being developed to improve performance, reduce pause times, and adapt to different types of workloads and environments. As such, it remains an essential component of modern programming languages and an area of ongoing research and development.