Simulating & troubleshooting StackOverflowError in Scala
In this series of simulating and troubleshooting performance problems in Scala, let’s discuss how to simulate StackOverflowError. StackOverflowError is a runtime error, which is thrown, when a thread’s stack size exceeds its allocated memory limit.
Sample Program
Here is a sample Scala program, which generates the StackOverflowError:
package com.yc class StackOverflowApp { } object StackOverflowApp { def main(args: Array[String]): Unit = { System.out.println("hit enter to start the StackOverflowApp") System.in.read() start() } def start(): Unit = { another() } def another(): Unit = { start() } }
You can notice the sample program contains the ‘StackOverflowApp’ class. This program does the following:
- ‘main()’ method of this class invokes ‘start()’ method
- ‘start()’ method invokes ‘another()’ method
- ‘another()’ method invokes ‘start()’ method once again
Thus ‘start()’ and ‘another()’ methods (i.e. #b and #c) call each other recursively for an infinite number of times.
As per the implementation, the start() method and the another() method will be added to the thread’s stack frame an infinite number of times. Thus, after a few thousand iterations, the thread’s stack size limit would be exceeded. Once the stack size limit is exceeded it will result in ‘StackOverflowError’.
Execution
When we executed above program, as expected ‘java.lang.StackOverflowError’ was thrown in seconds:
java.lang.StackOverflowError at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) at com.yc.StackOverflowApp$.start(StackOverflowApp.scala:15) at com.yc.StackOverflowApp$.another(StackOverflowApp.scala:19) : :
How to diagnose ‘java.lang.StackOverflowError’?
You can diagnose StackOverflowError either through a manual or automated approach.
Manual approach
When an application experiences ‘StackOverflowError’ it will be either printed in the application log file or in a standard error stream. From the stacktrace you will be able to figure which line of code causing the infinite looping.
Automated approach
On the other hand, you can also use yCrash open source script, which would capture 360-degree data (GC log, 3 snapshots of thread dump, heap dump, netstat, iostat, vmstat, top, top -H,…) from your application stack within a minute and generate a bundle zip file. You can then either manually analyze these artifacts or upload it to yCrash server for automated analysis.
We used the Automated approach. Once the captured artifacts were uploaded to the yCrash server, it instantly generated the below root cause analysis report highlighting the source of the problem.
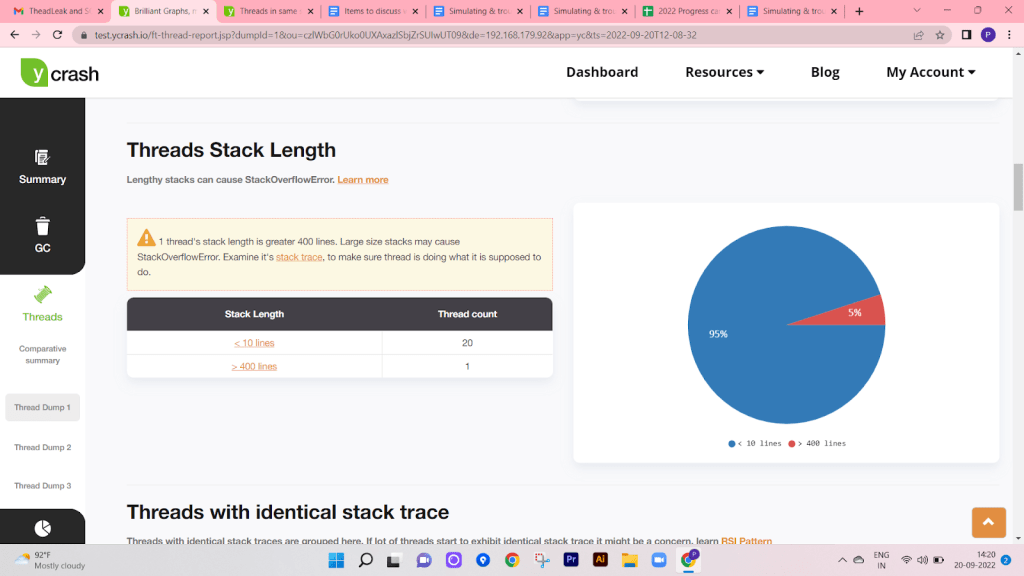
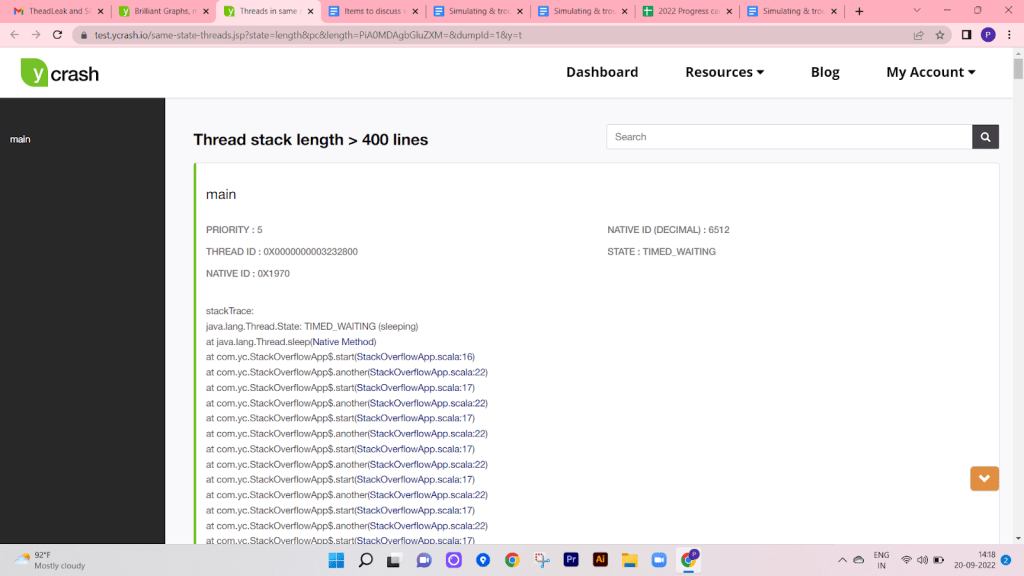
You can notice the yCrash tool precisely pointing out the thread stack length is greater than 400 lines and it has potential to generate StackOverflowError. The Tool also points out the stack trace of the thread which is going on an infinite loop.
Using this information from the report, one can easily go ahead and fix the StackOverflowError.
Published on Java Code Geeks with permission by Ram Lakshmanan, partner at our JCG program. See the original article here: Simulating & troubleshooting StackOverflowError in Scala Opinions expressed by Java Code Geeks contributors are their own. |