Testing with Hoverfly and Java Part 2: Delays
On the previous post we implemented json and Java based Hoverfly scenarios..
Now it’s time to dive deeper and use other Ηoverfly features.
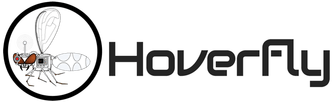
A big part of testing has to do with negative scenarios. One of them is delays. Although we always mock a server and we are successful to reproduce erroneous scenarios one thing that is key to simulate in todays microservices driven world is delay.
So let me make a server with a 30 secs delay.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 | public class SimulateDelayTests { private Hoverfly hoverfly; @BeforeEach void setUp() { .get( "/delay" ) .willReturn(success( "{\"username\":\"test-user\"}" , "application/json" ).withDelay( 30 , TimeUnit.SECONDS))); var localConfig = HoverflyConfig.localConfigs().disableTlsVerification().asWebServer().proxyPort( 8085 ); hoverfly = new Hoverfly(localConfig, SIMULATE); hoverfly.start(); hoverfly.simulate(simulation); } @AfterEach void tearDown() { hoverfly.close(); } } |
Let’s add the Delay Test
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 | @Test void testWithDelay() { var client = HttpClient.newHttpClient(); var request = HttpRequest.newBuilder() .build(); var start = Instant.now(); var res = client.sendAsync(request, HttpResponse.BodyHandlers.ofString()) .thenApply(HttpResponse::body) .join(); var end = Instant.now(); Assertions.assertEquals( "{\"username\":\"test-user\"}" , res); var seconds = Duration.between(start, end).getSeconds(); Assertions.assertTrue(seconds >= 30 ); } |
Delay simulation is there, up and running, so let’s try to simulate timeouts.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 | @Test void testTimeout() { var client = HttpClient.newHttpClient(); var request = HttpRequest.newBuilder() .timeout(Duration.ofSeconds( 10 )) .build(); assertThrows(HttpTimeoutException. class , () -> { try { client.sendAsync(request, HttpResponse.BodyHandlers.ofString()).join(); } catch (CompletionException ex) { throw ex.getCause(); } } ); } |
That’s it we got delays and timeouts!
Other test scenarios should contain state which is covered on the next tutorial.
Published on Java Code Geeks with permission by Emmanouil Gkatziouras, partner at our JCG program. See the original article here: Testing with Hoverfly and Java Part 2: Delays Opinions expressed by Java Code Geeks contributors are their own. |