Java Exercises for Beginners
Many people who tried learning to program never become programmers. Why? Among all the reasons, one can single out the most important: these people didn’t have enough practice. So if searching for Java programming exercises is a clever decision. Plus, practicing programming itself is a very exciting experience (much more exciting than reading theory!) and if you do it the right way, it will quickly lead you to your goal of becoming a programmer.
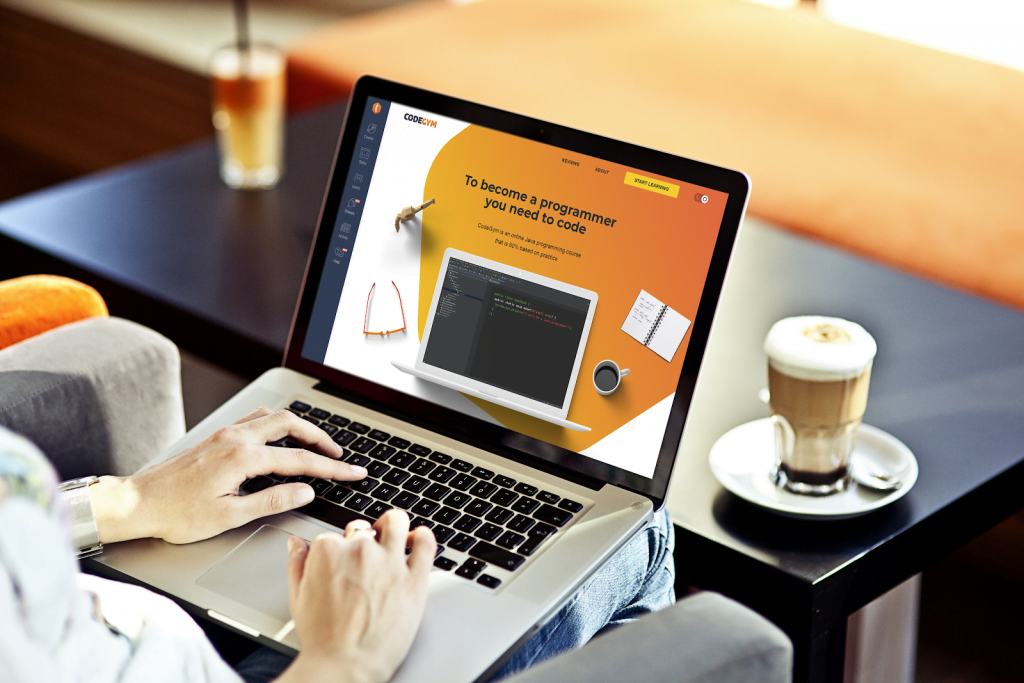
Why practice?
- “Learning to swim without diving into water”
- “How to play violin. No violin required!”
- “Java in six months without writing any code”.
What do these imaginary bestsellers have in common? Well, first of all they don’t exist. They could exist only as science fiction books, because they don’t work in reality. Swimming, playing a musical instrument and programming are all hands-on activities! Even if you learn the physics of the contact of rigid bodies with water, but at the same time avoid the pool, you will not learn to swim for sure. No matter how much you learn music theory, you won’t play the violin until you put years of practice into it. It’s the same with programming! Only you can learn it much faster than a violin.
By the way: theory is also important. If you devote 80% of your time to coding and 20% to learning theory, you will succeed!
How to organize your practicing
You can do it in different ways. The main thing is that these activities are suitable for you, that they are not too difficult and not too easy.
- Make a rough plan of your programming learning. You may use the table of contents for a Java book or the schedule of online courses as an example.
- Make a learning schedule. If you’re serious in your desire to become a software developer, it is a good idea to practice every day, or at least every other day. For how many hours? It depends on your free time.
- Actually learning. Dedicate 20% of learning time to theory reading and 80% to code. This is a proven formula and it works.
- Do not be lazy to make a short summary of your knowledge regularly. You may write a summary on paper or use your favorite application or blogging platform for it.
- Read your own code and someone else’s code carefully.
- Ask questions. If you don’t have programmers friends, check out specialized forums, there are many of them.
Where to find Java beginner’s exercises and how to solve them?
Okay, you may say. Where can I find Java exercises for beginners? And how do you make sure your code does what it should? In fact, when we created the CodeGym course, we started from exactly these needs of novice programmers.
That’s what CodeGym is
First, it is a universal learning platform for anyone learning Core Java. Except for the course here you can find articles on Java, a forum where you can ask questions about any programming topic, exercises to recreate classic video games, and much more. CodeGym course is dedicated to Core Java, covers all its topics and a little more.
Practice in CodeGym course: how it works?
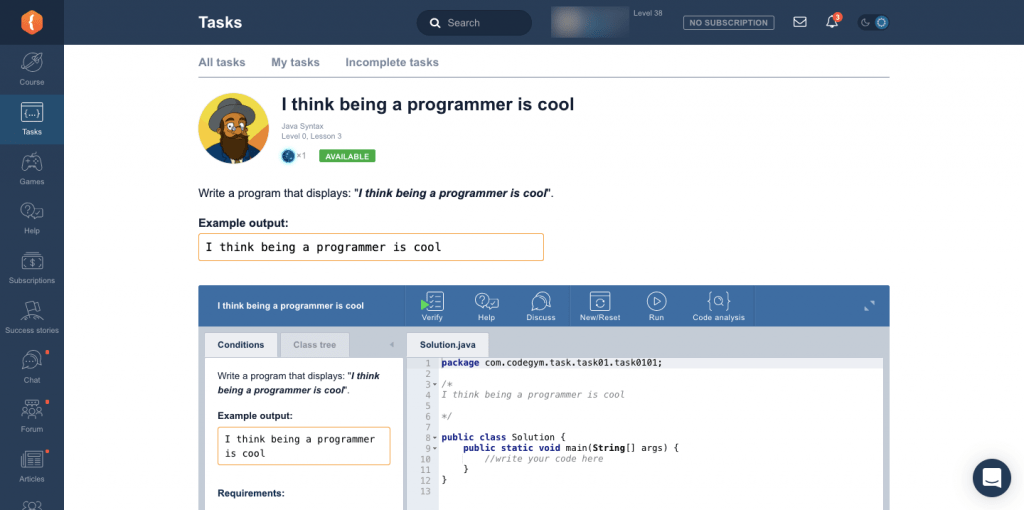
The course consists of lectures and a huge number of tasks. Most CodeGym students call the exercises system the main feature of the course.
- Firstly, there are more than 1200 coding tasks here. Some of them are very simple, others will make you sweat, think, ask questions, google…
- The condition of the problem is divided into subclauses, which greatly helps beginners not to get confused in the condition.
- An automatic solution validation is built into the course. When you have solved the problem, you send it for verification to a special CodeGym server. If the decision is correct, you get points — dark matter. Points get you access to the following lectures.
- If there is a mistake in the solution, a smart validator will tell you where it is and give you some tips on how to fix it.
- If you don’t understand how to solve the problem anyway, click on the help button, and you will find yourself in a special section of CodeGym Help — the other students and CodeGym buddies definitely help to solve the problem.
- You always can postpone the difficult task and come back to it later.
- By the way, you can solve problems both directly on the CodeGym website and via the IntelliJ IDEA development environment. It is a professional tool that almost all Java developers use.
CodeGym walkthrough achievement — Java Developer Skill!
Progressing through CodGym is like walking through a video game.
The course is divided into 4 parts. Each part has 10 levels. Your alter ego is the robot named Amigo — a novice programmer that you are pumping as an RPG protagonist.
How it works:
- You open a lecture, read it.
- If necessary, read additional materials and proceed to the coding tasks.
- You get points called dark matter for solving problems. You can spend them on opening new lectures.
- So gradually, lecture by lecture, task by task, level by level, you are pumping your skill as a Java programmer up.
- Once you complete all 40 levels, you will have a solid Core Java knowledge backed up by approximately 500 hours of hands-on practice.
What kind of Java exercises should you solve?
Java basics
The abc of language. Java basics means language constructs and the main general principles of programming. The first is language specific, the second is common to most programming languages.
Topics:
- First Java programs aka “Hello, World”. Keyboard output
- Variables, methods, and classes
- Primitive DataTypes: int, double, char, boolean
- Work with String
- Basics of keyboard input in Java
- Conditions (if-else)
- Loops (for and while)
- Introduction to Classes and Objects
- Arrays
Where to find the tasks: CodeGym, Java Syntax Quest, level 0 to 7.
Object-Oriented Programming (OOP)
Well, maybe OOP fundamentals is not the most difficult topic, but extremely important. It contains a lot of subtleties on which interviewers like to entrap future Java Juniors. The CodeGym course contains lectures to help you understand the philosophy of OOP and practical programming tasks for beginners that will help you understand the object-oriented principles through the practice.
Topics:
- Classes and objects in general
- Condition and behavior of the object
- Inheritance
- Encapsulation
- Polymorphism
- Overloading and overriding
- Abstraction and abstract classes
- Virtual Methods
- Interfaces
- Interface implementations
- InstanceOf
- Access Modifiers
- The order of Constructor calls
Where to learn and find tasks:
CodeGym Java Core Quest, levels 1, 2, 3, 4, 5.
Java Collections and Data Structures
If you understand the data structures and their fundamental differences, you can correctly apply them for different tasks. And this is a kind of superpower of the developer. Java Collection Framework is a hierarchy of interfaces and their implementations that is part of the JDK and allows the developer to use a large number of data structures out of the box. Java collections exercises are represented on CodeGym widely for beginners and intermediate students.
Topics:
- Arrays
- ArrayList, LinkedList
- HashSet, HashMap
- Iterable
- Collection Interface
- List Interface and implementations
- Map Hierarchy
- Set Interface and implementations
- Queue
- Trees, Red-Black trees
- Iterators
Where to learn and find tasks:
CodeGym Quest Java Syntax, levels 7, 8 (Collections, Arrays, and Lists for beginners)
CodeGym Quest Collections, levels 6, 7
Exceptions
Exception handling is a special mechanism that is responsible for working with abnormal situations in Java. This mechanism greatly simplifies the process of bug catching in Java programs. To use it effectively, you need to figure out how exception handling is arranged in Java. There are a bunch of such tasks on CodeGym, and you can meet them even in the very first Java Syntax quest.
Topics:
- Stack Trace
- Exceptions types
- Try Catch Finally construction
- Runtime Exceptions
- IOExceptions
- Multi-Catch
Where to learn and find tasks:
CodeGym Java Syntax Quest, level 9 and many other tasks.
Input/output streams
Admittedly, Java I/O streams are complex. However Java students start using Input/Output streams way before having any idea of them. Even the very first Java programs contain this System.out.println thing. According to I/O streams complexity at first it is almost impossible to understand their work, moreover, it is not necessary. It is better to do a little bit later, when you already know the basics and solve some coding tasks.
Topics:
- Introduction to Input/Output Streams
- FileInputStream and FileOutputStream
- InputStream and OutputStream
- BufferedInputStream
- Create your own wrapper for System.in
- Adapter
- Reader and Writer
- FileReader and FileWriter
- BufferedReader and InputStreamReader
- Your own wrapper for System.out
Where to learn and find tasks:
CodeGym Java Core Quest, levels 8, 9.
Generics
Generalization is the very essence of automation, that is, in a sense, and programming. So the topic of generics or generics in Java shouldn’t be overlooked either. CodeGym has Generic programming tasks.
Topics:
- What are Generics
- Generics: super, extends, list
- Type Erasure
- Generics: Class
- Generics: ? wildcard
Where to learn and find tasks:
CodeGym, mostly in the Java Collections Quest, starting at level 5.
Concurrency/Multithreading
Multithreading in Java is a legendary topic. On the one hand, it is multithreading that is admittedly the most difficult topic in whole Core Java. On the other hand, experts recognize that Concurrency is one of the very strong and well-organized features of Java. So you have to puzzle it over. The main thing is to solve a sufficient number of problems. Well, one cannot do without repeated reading of the theory as well.
Topics:
- What is thread
- Creating and starting new threads
- Join
- Creating and stopping threads: start, interrupt, sleep, yield
- Marker interface and deep copies
- Synchronized, volatile
- Deadlock, Wait. notify, notifyAll
Where to learn and find tasks:
CodeGym Java Core Quest, levels 6, 7; Java Multithreading Quest.
More tasks?
You will find even more Java Core + topics on CodeGym. For example:
- Object class and its methods
- Unit testing
- JSON/Jackson
- Design Patterns
- RMI and dynamic proxy
- Annotations
Well…
Good luck with your learning dear fellow future programmer. Stay calm and continue to code!