Hands on Optional value
Optional is in air due to coronavirus, everything is becoming optional like optional public gathering , optional work from home, optional travel etc.
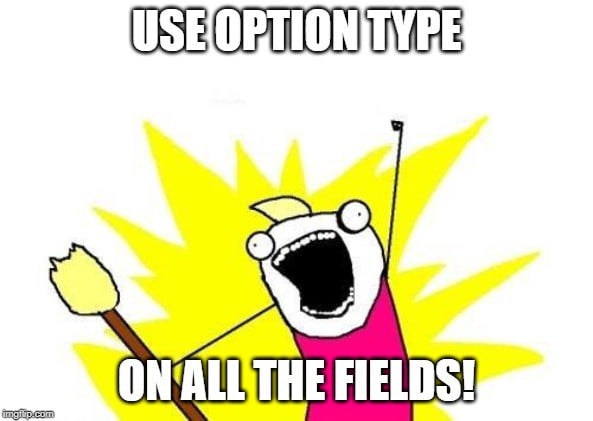
I though it is good time to talk about real “Optional” in software engineering that deals with NULL reference.
Tony Hoare confessed that he made billion dollar mistake by inventing Null. If you have not seen his talk then i will suggest to have look at Null-References-The-Billion-Dollar-Mistake.
I will share some of the anti pattern with null and how it can be solved using abstraction like Optional or MayBe.
For this example we will use simple value object that can have some null values.
public class Person { final String firstName; final String lastName; final String email; // This can be null final String phone; //This can be null }
This value object can have null value for email & phone number.
Scenario: Contact Person on both email and phone number
Not using optional
First attempt will be based on checking null like below
//Not using optional if (p.email != null) { System.out.println("Sending email to " + p.email); } if (p.phone != null) { System.out.println("Calling " + p.phone); }
This is how it has been done for years. One more common pattern with collection result.
List<Person> p = searchPersonById("100"); if (p.isEmpty()) { System.out.println("No result"); } else { System.out.println("Person" + p.get(0)); }
Use optional in wrong way
Optional<String> phone = contactNumber(p); Optional<String> email = email(p); if (phone.isPresent()) { System.out.println("Calling Phone " + phone.get()); } if (email.isPresent()) { System.out.println("Sending Email " + email.get()); }
This is little better but all the goodness of Optional is thrown away by adding if/else block in code.
Always Happy optional
//Always Happy Optional<String> phone = contactNumber(p); Optional<String> email = email(p); System.out.println("Calling Phone " + phone.get()); System.out.println("Sending Email " + email.get());
It is good be happy but when you try that with Optional you are making big assumption or you don’t need optional.
Nested property optional
For this scenario we will extend Person object and add Home property. Not everyone can own home so it is good candidate that it will be not available .
Lets see how contacting person scenario work in this case
//Nested Property if (p.getHome() != null) { System.out.println("Sending Postal mail " + p.getHome().address); } if (p.getHome() != null && p.getHome().getInsurance() != null) { System.out.println("Sending Notification to insurance " + p.getHome().getInsurance().getAgency()); }
This is where it start to become worse that code will have tons of nested null checks.
Priority based default
for this scenario we first try to contact person on home address and if it is not available then contact on office address.
//Address has priority , first home and then Office if (p.home != null) { System.out.println("Contacted at home address " + p.home.address); return; // Magical return for early exit } if (p.office != null) { System.out.println("Contacted at office address " + p.office.address); return; // Magical return for early exit }
Such type of scenario require use of advance control flow for early return and makes code hard to understand and maintain.
These are some of the common pattern where optional are not used or used in wrong way.
Optional usage patterns
Lets look at some of good ways of using optional.
Make property optional based on domain knowledge
It is very easy to makes property optional.
public Optional<String> getEmail() { return Optional.ofNullable(email); } public Optional<String> getPhone() { return Optional.ofNullable(phone); }
Yes it is allowed to make get Optional, no one will hang you for that and feel free to do that without fear. Once that change is done we can write something like below
//Use Optional p.getEmail().ifPresent(email -> System.out.println("Sending email to " + email)); p.getPhone().ifPresent(phone -> System.out.println("Calling " + phone)); //Optional for Collection or Search type of request OptionalIt looks neat, first step to code without explicit if else on application layer.
Use some power of Optional
//Use IfPresent & other cool things phone .filter(number -> hasOptIn(number)) .ifPresent(number -> System.out.println("Calling Phone " + number)); email .filter(m -> hasOptIn(m)) .ifPresent(m -> System.out.println("Sending Email " + m));Optional is just like stream, we get all functional map,filter etc support. In above example we are checking for OptIn before contacting.
Always happy optional
Always happy optional that calls"get" without check will cause runtime error on sunday midnight, so it advised to use ifPresent
//Don't do this System.out.println("Calling Phone " + phone.get()); System.out.println("Sending Email " + email.get()); //Use ifPresent to avoid runtime error phone.ifPresent(contact -> System.out.println("Sending email to " + contact)); email.ifPresent(contact -> System.out.println("Calling " + contact));Nested Optional
p.getHome().ifPresent(a -> System.out.println("Sending Postal mail " + a.address)); p.getHome() .flatMap(Person.Home::getInsurance) .ifPresent(a -> System.out.println("Sending Notification to insurance " + a.agency));Flatmap does the magic and handles null check for home and convert insurance object also.
Priority based default
//Address has priority , first home and then Office Optional<String> address = Stream .of(person.getHome().map(Home::getAddress), person.getOffice().map(Office::getAddress)) .filter(Optional::isPresent) .map(Optional::get) .findFirst(); address .ifPresent(add -> System.out.println("Contacting at address " + add));This example is taking both home & office address and pick the first one that has value for sending notification. This particular pattern avoids lots of nested loops.
Else branch
Optional has lots of ways to handle else part of the scenario like returning some default value(orElse) , lazy default value (orElseGet) or throw exception(orElseThrow).
What is not good about optional
Each design choice has some trade off and optional also has some. It is important to know what are those so that you can make careful decision.
Memory overhead
Optional is container that holds value, so extra object is created for every value. Special consideration is required when it holds primitive value. If some performance sensitive code will be impacted by extra object creation via optional then it is better to use null.
Memory indirection
As optional is container , so every access to value need extra jump to get real value. Optional is not good choice for element in array or collection.
No serialization
I think this is good decision by Jdk team that does not encourage people to make instance variable optional. You can wrap instance variable to Optional at runtime or when required for processing.
Published on Java Code Geeks with permission by Ashkrit Sharma, partner at our JCG program. See the original article here: Hands on Optional value
Opinions expressed by Java Code Geeks contributors are their own.
Hi…
I’m Elena gillbert.An optional value either contains a value or contains nil to indicate that the value is missing. Write a question mark ( ? ) after the type of a value to mark the value as optional. Why would you want to use an optional value?
This is good question. I wanted to write about this in blog but missed it. ‘?’ operator is available in some language like groovy and it is used for safe navigation of null value. This option was considered by java but they dropped it and took some inspiration from Scala or haskell. Null safe operator has few thing that does not make it that good selection. 1 – It Hides the real question like why object is null or does it has any business meaning 2 – Does not have option to handle the else part like supply some default… Read more »