Call That An Exception?
While this is a Java example to do with testing and wiremock, it relates to a more universal problem.
We were trying to retry Wiremock’s verify
method, which may be called by our test before the endpoint we’re checking is hit. In that situation, we’d want to try again a few seconds later in a loop until timing out. Interesting, the Wiremock client doesn’t provide a method like this, but meh, they’re easily created.
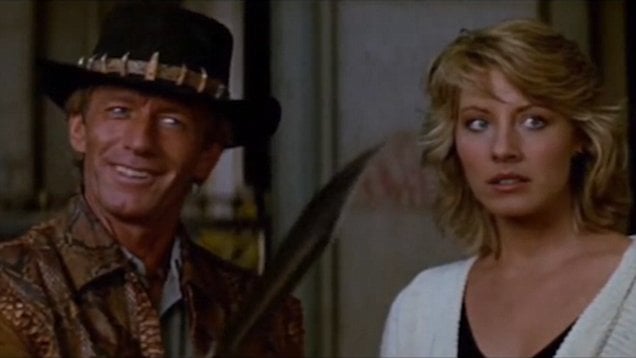
The type of object thrown was called VerificationException
so we wrote something like this:
01 02 03 04 05 06 07 08 09 10 | for ( int i= 0 ; i<maxRetries; i++) { try { verify... // try to verify return ; // verify ok } catch (Exception e) { LOG.info( "Oooh, it went wrong on try " + i); // let the loop run it again after a sleep sleep( 1000 ); } } |
It didn’t work. Our catch block wasn’t hit.
Digging deeper, and always read the source code of the open source libraries you use, it seems like the VerificationException
is derived from AssertionError
.
An Error
is not an Exception
. So why is the VerificationException
not called the VerificationError
? Our catch
block needed to catch Error
or Throwable
to work. Which it now does and it does work.
What Went Wong?
This is a case of violating the principle of least surprise. Because the thrown object was called exception, nobody would imagine that it was anything else. We needed to write a failing exception catcher, debug it, and read a couple of classes deep in the source code to find this mistake. Was it our mistake for expecting an exception to be an exception?
You can easily explain why they chose the misleading name, but if you have to explain something that violates the norm, then you would be better putting the effort in to make it not require an explanation.
Published on Java Code Geeks with permission by Ashley Frieze, partner at our JCG program. See the original article here: Call That An Exception? Opinions expressed by Java Code Geeks contributors are their own. |
It was your mistake to expect VerificationException to be Exception. Misleading or not, catch the expected exception instead of using Exception.
In some situations that may be true. In this situation, we were writing a general purpose retry strategy, which needed to retry all exceptions. The fact that one of the advertised exceptions was an Error was an unexpected move on the part of the author of that class.
Hi…
I’m Elena gillbert.An exception is an event, which occurs during the execution of a program, that disrupts the normal flow of the program’s instructions. When an error occurs within a method, the method creates an object and hands it off to the runtime system. … This block of code is called an exception handler.