Object and Index Streams
I was going to write a post about how to blend a stream with the indices of each element, but the folks over on Baeldung have covered this subject enormously well! Given I’m part of their editorial team, I’m proud of them/us.
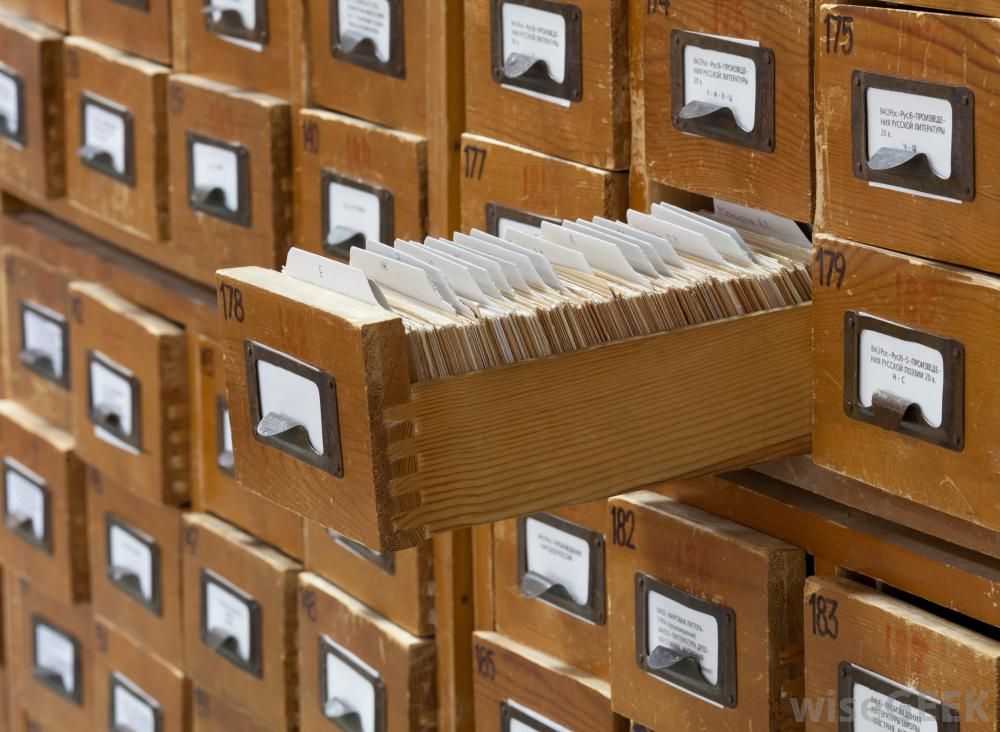
It’s interesting how functional programming in Java, Streams especially, have trained us out of some common patterns. Example:
List<Foo> foos = new ArrayList<>(); for (int i=0; i < bars.size(); i++) { foos.add(new Foo(i, bars.get(i)); }
The above code creates a new Foo from the index and the bar from a source list.
We don’t like using add in the midst of a for loop, when Stream.collect is a bigger signal that we’re pulling data from an iteration. Similarly, we don’t like hiding the effective Stream.map inside the loop, when we could express it more explicitly in a streaming declaration. We all know that the above ought to read:
List<Foo> foos = bars.stream() .map( ... something ...) .collect(toList());
The awkward challenge is that the something can’t easily be done.
How to choose what to do
On the whole, cooking a complex brew of functions around a streaming operation is a way of hiding the very thing that the stream was meant to show us – namely how the data from one place goes to another.
So here are some thoughts:
- Maybe just have a for loop – it might be easier
- If you find that the main player within the body of the operation is the index, then make a stream of indices, and mix in the object with a List.get at the last minute, assuming your list is efficiently indexed
- If the main player is an object, then maybe store a counter (AtomicInteger is a good bet) and increment it at the last minute, watching out for ordering and parallel streams
- If the code gets complex, try to divorce the streaming logic from your business logic by extracting a function
- Maybe delegate this to a library – the Baeldung article shows ProtonPack and others that might just be better and which come ready tested and documented.
Whatever you do, don’t make things difficult for yourself on a point of principle.
Published on Java Code Geeks with permission by Ashley Frieze, partner at our JCG program. See the original article here: Object and Index Streams Opinions expressed by Java Code Geeks contributors are their own. |
spam article?