JVM with no garbage collection
JVM community keeps on adding new GC and recently new one was added and it is called Epsilon and is very special one. Epsilon only allocates memory but will not reclaim any memory.
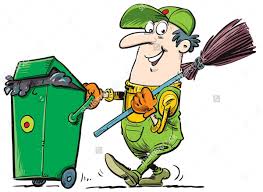
It might look like what is use of GC that does not perform any garbage collection. This type of Garbage Collector has special use and we will look into some.
Where this shinny GC can be used ?
Performance Testing
If you are developing solution that has tight latency requirement and limited memory budget then this GC can be used to test limit of program.
Memory Pressure Testing
Want to know extract transient memory requirement by your application. I find this useful if you are building some pure In-Memory solution.
Bench marking Algorithm.
Many time we want to test the real performance of new cool algorithm based on our understanding of BIG (O) notion but garbage collector adds noise during testing.
Low Garbage
Many times we do some optimization in algorithm to reduce garbage produced and GC like epsilon helps in scientific verification of optimization.
How to enable epsilon GC
JVM engineers have taken special care that this GC should not enabled by default in production , so to use this GC we have to use below JVM options
-XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC -Xlog:gc
One question that might be coming in your mind what happens when memory is exhausted ? JVM will stop with OutofMemory Error.
Lets look at some code to test GC
How to know if epsilon is used in JVM process?
Java has good management API that allows to query current GC being used, this can also be used to verify what is the default GC in different version of java.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | public class VerifyCurrentGC { public static void main(String... args) { var gcBeans = ManagementFactory.getGarbageCollectorMXBeans(); gcBeans.stream().forEach(gc -> { out.println(format( "GC Name : %s" , gc.getName())); var poolNames = gc.getMemoryPoolNames(); if (poolNames != null ) { List.of(poolNames).forEach(pool -> out.println(format( "Pool name %s" , pool))); } else { out.println( "No memory pools for " + gc.getName()); } }); } } |
Run above code with below options
-XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC VerifyCurrentGC
How does code behave when memory is exhausted.
I will use below code to show how new GC works.
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 | public class MemoryAllocator { public static final int KB = 1024 ; static int mbToAllocate = Integer.getInteger( "mb" , 1000 ); public static void main(String[] args) { System.out.println(String.format( "Start allocation of %s MBs" , mbToAllocate)); for (var i = 0 ; i < mbToAllocate; i++) { var garbage = new byte [KB * KB]; } System.out.println( "I was Alive after allocation" ); } } |
Running above code with default GC and requesting 5GB allocation causes no issue (java -Xlog:gc -Dmb=5024 MemoryAllocator) and it produces below output
[0.016s][info][gc] Using G1
[0.041s][info][gc] Periodic GC disabled
Start allocation of 5024 MBs
[0.197s][info][gc] GC(0) Pause Young (Concurrent Start) (G1 Humongous Allocation) 116M->0M(254M) 3.286ms
[0.197s][info][gc] GC(1) Concurrent Cycle
[0.203s][info][gc] GC(1) Pause Remark 20M->20M(70M) 4.387ms
[0.203s][info][gc] GC(1) Pause Cleanup 22M->22M(70M) 0.043ms
[1.600s][info][gc] GC(397) Concurrent Cycle 6.612ms
[1.601s][info][gc] GC(398) Pause Young (Concurrent Start) (G1 Humongous Allocation) 52M->0M(117M) 1.073ms
[1.601s][info][gc] GC(399) Concurrent Cycle
I was Alive after allocation
[1.606s][info][gc] GC(399) Pause Remark 35M->35M(117M) 0.382ms
[1.607s][info][gc] GC(399) Pause Cleanup 35M->35M(117M) 0.093ms
[1.607s][info][gc] GC(399) Concurrent Cycle 6.062ms
Lets add some memory limit ( java -XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC -Xlog:gc -Xmx1g -Dmb=5024
MemoryAllocator)
[0.011s][info][gc] Resizeable heap; starting at 253M, max: 1024M, step: 128M
[0.011s][info][gc] Using TLAB allocation; max: 4096K
[0.011s][info][gc] Elastic TLABs enabled; elasticity: 1.10x
[0.011s][info][gc] Elastic TLABs decay enabled; decay time: 1000ms
[0.011s][info][gc] Using Epsilon
Start allocation of 5024 MBs
[0.147s][info][gc] Heap: 1024M reserved, 253M (24.77%) committed, 52640K (5.02%) used
[0.171s][info][gc] Heap: 1024M reserved, 253M (24.77%) committed, 103M (10.10%) used
[0.579s][info][gc] Heap: 1024M reserved, 1021M (99.77%) committed, 935M (91.35%) used
[0.605s][info][gc] Heap: 1024M reserved, 1021M (99.77%) committed, 987M (96.43%) used
Terminating due to java.lang.OutOfMemoryError: Java heap space
This particular run caused OOM error and is good confirmation that after 1GB this program will crashed.
Same behavior is true multi thread program also, refer to MultiThreadMemoryAllocator.java for sample.
Unit Tests are available to test features of this special GC.
I think Epsilon will find more use case and adoption in future and this is definitely a good step to increase reach of JVM.
All the code samples are available Github repo
If you like the post then you can follow me on twitter .
Published on Java Code Geeks with permission by Ashkrit Sharma, partner at our JCG program. See the original article here: JVM with no garbage collection Opinions expressed by Java Code Geeks contributors are their own. |