Factory Method Design Pattern
Factory Method pattern is one of the popular creational design patterns. It doesn’t specifically rely on a factory object to create the objects. Rather, the idea is to use a separate method in the same class to create an object.
Factory Method pattern defines an interface for creating objects but lets the subclasses decide how to instantiate its objects. Each subclass must define its Factory Method.
In this tutorial, we’ll learn how to implement a Factory Method design pattern with the help of an example of a VehicleStore.
Defining a VehicleStore:
Let’s start by defining a VehicleStore class:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 | public abstract class VehicleStore { public Vehicle orderVehicle(VehicleType type) { Vehicle vehicle = createVehicle(type); vehicle.getServiced(); vehicle.polish(); vehicle.provideClearance(); return vehicle; } public abstract Vehicle createVehicle(VehicleType type); } |
Where the VehicleType is an enum defining the type of vehicle:
1 | public enum VehicleType { BIKE, CAR, TRUCK, CRANE }; |
Note that we have defined the createVehicle() method as abstract; the one which will be responsible for the creation of a specific type of a vehicle.
Defining SubClasses:
Now, let’s say we have two types of a VehicleStore – LightVehiclesStore and HeavyVehiclesStore:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | public class LightVehiclesStore extends VehicleStore { @Override public Vehicle createVehicle(VehicleType type) { switch (type) { case BIKE : return new Bike(); case CAR : return new Car(); } return null ; } } public class HeavyVehiclesStore extends VehicleStore { @Override public Vehicle createVehicle(VehicleType type) { switch (type) { case TRUCK : return new Truck(); case CRANE : return new Crane(); } return null ; } } |
The LightVehiclesStore is responsible for selling lightweight vehicles like a bike or a car. On the other hand, HeavyVehiclesStore sells trucks and cranes.
As we can see, both of these subclasses override the createVehicle() method.
UML Diagram for Our Example:
We can represent the above example with a UML diagram similar to:
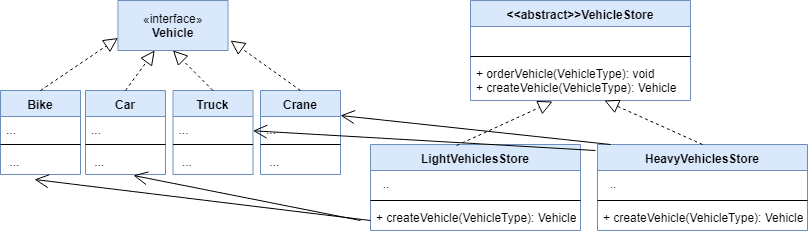
The type of Vehicle we create is decided in the concrete subclasses. Also, we have exposed the VehicleStore as the common interface for these subclasses.
We can further go a step ahead and define a Factory Object for the VehicleStore to avoid exposing these subclasses directly to the client code.
Conclusion:
In this tutorial, we explored an important creational pattern – the Factory Method Pattern. The central idea of this pattern is to let the subclasses decide how to instantiate objects.
Some of the popular implementations of this pattern are available in our Java API. Some of these include Calendar.getInstance(),java.text.NumberFormat.getInstance() and java.util.ResourceBundle.getBundle() methods.
Published on Java Code Geeks with permission by Shubhra Srivastava, partner at our JCG program. See the original article here: Factory Method Design Pattern Opinions expressed by Java Code Geeks contributors are their own. |
Need some examples explaining how to create each obj car, bike etc, particularly in a main method of a drive class.
They are no special objects/classes. You can customize those classes to suit your needs say by defining a few properties and then passing them into the constructor.
Didn’t used all of that to keep things simple..