Annotation Handling and JPMS
TLDR; Instead of annotation.getClass().getMethod("value")
call annotation.annotationType().getMethod("value")
.
All Java developers have heard about annotations. Annotations are with us since Java 1.5 (or only 1.6 if you insist). Based on my experience interviewing candidates I feel that most Java developers know how to use annotations. I mean, most developers know that it looks like @Test
, or @Override
and that they come with Java or with some library and they have to be written in front of a class, method, or variable.
A few developers know that you can also define an annotation in your code using @interface
and that your code can do some metaprogramming using the annotation. Even fewer know that annotations can be processed by annotation processors and some of them can be processed during run time.
I could continue but long story short is that annotations are a mystery for most Java developers. If you think I am wrong stating how clueless related to the annotations most of the Java developers are, then consider that the number of programmers, (or coders, generally) was growing exponentially during the last 30 years and Java developers, especially, was doing so during the last 20 years and it is still growing exponentially. The exponential function has this feature: If the number of whatnots is growing exponentially then most of the whatnots are young.
That is the reason why most Java developers are not familiar with annotations.
To be honest, annotation handling is not something simple. It deserves its own article, especially when we want to handle annotations while using module systems.
During the final touches of the release 1.2.0 of the Java::Geci code generation framework I ran into a problem that was caused by my wrong use of annotations and reflection. Then I realized that probably most of the developers who handle annotations using reflection are doing so the same wrong way. There was hardly any clue on the net to help me understand the problem. All I found was a GitHub ticket and based on the information there I had to figure out what is really happening.
So let’s refresh a bit what annotations are and after that let’s have a look at what we may be doing wrong that was okay so far but may cause trouble when JPMS comes into the picture.
What is an annotation?
Annotations are interfaces that are declared using the interface
keyword preceded with the @
character. This makes the annotation usable in the code the way we got used to. Using the name of the annotation interface with the @
in front of it (e.g.: @Example). The most frequently used such annotation is @Override
that the Java compiler is using during compile time.
Many frameworks use annotations during run-time, others hook into the compilation phase implementing an annotation processor. I wrote about annotation processors and how to create one. This time we focus on the simpler way: handling annotations during run-time. We do not even implement the annotation interface, which is a rarely used possibility but is complex and hard to do as the article describes.
To use an annotation during run-time the annotation has to be available during run-time. By default, the annotations are available only during compile-time and do not get into the generated byte-code. It is a common mistake to forget (I always do) to put the @Retention(RetentionPolicy.RUNTIME)
annotation on the annotation interface and then starting to debug why I cannot see my annotation when I access it using reflection.
A simple run-time annotation looks like the following:
1
2
3
4
5
|
@Retention (RetentionPolicy.RUNTIME) @Repeatable (Demos. class ) public @interface Demo { String value() default "" ; } |
The annotations have parameters when used on classes, on methods, or on other annotated elements. These parameters are methods in the interface. In the example, there is only one method declared in the interface. It is called value()
. This is a special one. This is a kind of default method. If there are no other parameters of an annotation interface, or even if there are but we do not want to use the other parameters and they all have default values then we can write
1
|
@Demo ( "This is the value" ) |
instead of
1
|
@Demo (value= "This is the value" ) |
If there are other parameters that we need to use then we do not have this shortcut.
As you can see annotations were introduced on top of some existing structure. Interfaces and classes are used to represent annotations and it was not something totally new introduced into Java.
Starting with Java 1.8 there can be multiple annotations of the same type on an annotated element. You could have that feature even before Java 1.8. You could define another annotation, for example
1
2
3
4
|
@Retention (RetentionPolicy.RUNTIME) public @interface Demos { Demo[] value(); } |
and then use this wrapper annotation on the annotated element, like
1
2
3
4
5
|
@Demos (value = { @Demo ( "This is a demo class" ), @Demo ( "This is the second annotation" )}) public class DemoClassNonAbbreviated { } |
To ease the tendinitis, caused by excessive typing, Java 1.8 introduced the annotation Repeatable
(as you can see on the annotation interface Demo
) and that way the above code can be written simply as
1
2
3
4
|
@Demo ( "This is a demo class" ) @Demo ( "This is the second annotation" ) public class DemoClassAbbreviated { } |
How to read the annotation using reflection
Now that we know that the annotation is just an interface the next question is how can we get information about them. The methods that deliver the information about the annotations are in the reflection part of the JDK. If we have an element that can have an annotation (e.g. a Class
, Method
or Field
object) then we can call getDeclaredAnnotations()
on that element to get all the annotations that the element has or getDeclaredAnnotation()
in case we know what annotation we need.
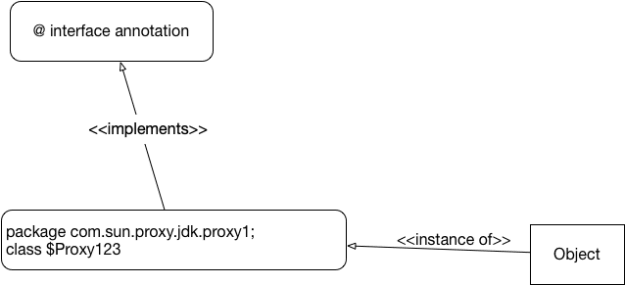
The return value is an annotation object (or an annotation array in the first case). Obviously, it is an object because everything is an object in Java (or a primitive, but annotations are anything but primitive). This object is the instance of a class that implements the annotation interface. If we want to know what string the programmer wrote between the parenthesis we should write something like
1
2
3
4
5
|
final var klass = DemoClass. class ; final var annotation = klass.getDeclaredAnnotation(Demo. class ); final var valueMethod = annotation.getClass().getMethod( "value" ); final var value = valueMethod.invoke(annotation); Assertions.assertEquals( "This is a demo class" , value); |
Because value is a method in the interface, certainly implemented by the class that we have access to through one of its instances we can call it reflectively and get back the result, which is "This is a demo class"
in this case.
What is the problem with this approach
Generally nothing as long as we are not in the realm of JPMS. We get access to the method of the class and invoke it. We could get access to the method of the interface and invoke it on the object but in practice, it is the same. (Or not in case of JPMS.)
I was using this approach in Java::Geci. The framework uses the @Geci
annotation to identify which class needs generated code inserted into. It has a fairly complex algorithm to find the annotations because it accepts any annotation that has the name Geci
no matter which package it is in and it also accepts any @interface
that is annotated with a Geci
annotation (it is named Geci
or the annotation has an annotation that is Geci
recursively).
This complex annotation handling has its reason. The framework is complex so the use can be simple. You can either say:
1
|
@Geci ( "fluent definedBy='javax0.geci.buildfluent.TestBuildFluentForSourceBuilder::sourceBuilderGrammar'" ) |
or you can have your own annotations and then say
1
|
@Fluent (definedBy= "javax0.geci.buildfluent.TestBuildFluentForSourceBuilder::sourceBuilderGrammar" ) |
The code was working fine up until Java 11. When the code was executed using Java 11 I got the following error from one of the tests
1
2
3
4
|
java.lang.reflect.InaccessibleObjectException: Unable to make public final java.lang.String com.sun.proxy.jdk.proxy1.$Proxy12.value() accessible: module jdk.proxy1 does not "exports com.sun.proxy.jdk.proxy1" to module geci.tools |
(Some line breaks were inserted for readability.)
The protection of JPMS kicks in and it does not allow us to access something in the JDK we are not supposed to. The question is what do we really do and why do we do it?
When doing tests in JPMS we have to add a lot of --add-opens
command-line argument to the tests because the test framework wants to access the part of the code using reflection that is not accessible for the library user. But this error code is not about a module that is defined inside Java::Geci.
JPMS protects the libraries from bad use. You can specify which packages contain the classes that are usable from the outside. Other packages even if they contain public interfaces and classes are only available inside the module. This helps module development. Users cannot use the internal classes so you are free to redesign them so long as long the API remains. The file module-info.java
declares these packages as
1
2
3
|
module javax0.jpms.annotation.demo.use { exports javax0.demo.jpms.annotation; } |
When a package is exported the classes and interfaces in the package can be accessed directly or via reflection. There is another way to give access to classes and interfaces in a package. This is opening the package. The keyword for this is opens
. If the module-info.java
only opens
the package then this is accessible only via reflection.
The above error message says that the module jdk.proxy1
does not include in its module-info.java
a line that exports com.sun.proxy.jdk.proxy1
. You can try and add an add-exports jdk.proxy1/com.sun.proxy.jdk.proxy1=ALL_UNNAMED
but it does not work. I do not know why it does not work, but it does not. And as a matter of fact, it is good that it is not working because the package com.sun.proxy.jdk.proxy1
is an internal part of the JDK, like unsafe
was, that caused so much headache to Java in the past.
Instead of trying to illegally open the treasure box let’s focus on why we wanted to open it in the first place and if we really need to access to that?
What we want to do is get access to the method of the class and invoke it. We can not do that because the JPMS forbids it. Why? Because the Annotation objects class is not Demo.class
(which is obvious since it is just an interface). Instead it’s a proxy class that implements the Demo
interface. That proxy class is internal to the JDK and so we can not call annotation.getClass()
. But why would we access the class of the proxy object, when we want to call the method of our annotation?
Long story short (I mean a few hours of debugging, investigating and understanding instead of mindless stackoverflow copy/paste that nobody does): we must not touch the value()
method of the class that implements the annotation interface. We have to use the following code:
1
2
3
4
5
|
final var klass = DemoClass. class ; final var annotation = klass.getDeclaredAnnotation(Demo. class ); final var valueMethod = annotation.annotationType().getMethod( "value" ); final var value = valueMethod.invoke(annotation); Assertions.assertEquals( "This is a demo class" , value); |
or alternatively
1
2
3
4
5
|
final var klass = DemoClass. class ; final var annotation = klass.getDeclaredAnnotation(Demo. class ); final var valueMethod = Demo. class .getMethod( "value" ); final var value = valueMethod.invoke(annotation); Assertions.assertEquals( "This is a demo class" , value); |
(This is already fixed in Java::Geci 1.2.0) We have the annotation object but instead of asking for the class of it we have to get access to the annotationType()
, which is the interface itself that we coded. That is something the module exports and thus we can invoke it.
Mihály Verhás, my son, who is also a Java developer at EPAM usually reviews my articles. In this case, the “review” was extended and he wrote a non-negligible part of the article.
Published on Java Code Geeks with permission by Peter Verhas, partner at our JCG program. See the original article here: Annotation Handling and JPMS Opinions expressed by Java Code Geeks contributors are their own. |